Open Mathias-Ooms opened 4 years ago
I'm trying to achieve a high frame rate from the client.simGetImages() for depthview. The highest frame rate I got was around 15 FPS with the following settings (below). I've tried to change the viewmode to "NoDisplay" but this drops the frame rate to 1 FPS and lowering the resolution in the CaptureSettings didn't help either.
Will test this, but the FPS drop in "NoDisplay" doesn't make sense. Lowering resolution should also help
Is there a way to disable newly added animals?
AFAIK, no, you'll have to modify the uncooked env for this, but need to see if it's possible Edit: Opened https://github.com/microsoft/AirSim/pull/2561 to see if it's possible and works, WIP
@rajat2004 That merged pull request solved this issue?
@jonyMarino I haven't yet tried out the new simDestroyObject
, simSpawnObject
etc APIs, but they should work
Will try to test them out soon and maybe open a PR with some example files
OS: Ubuntu 18.04.4 LTS Using AirSim Neighborhood binary v1.3.0-Linux Python version: 3.6.9 My hardware: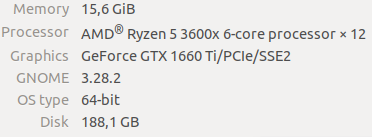
Dear developers, I'm trying to achieve an AirSim RL environment (much like the OpenAI environments). Following the car RL example in the documentation, I've created the following class below. However I stumbled upon some issues:
I'm trying to achieve a high frame rate from the client.simGetImages() for depthview. The highest frame rate I got was around 15 FPS with the following settings (below). I've tried to change the viewmode to "NoDisplay" but this drops the frame rate to 1 FPS and lowering the resolution in the CaptureSettings didn't help either.
Running the binary for a long time results sometimes in a sudden crash (log below), I'm running the binary as follows: ./AirSimNH.sh -vulkan -novsync -benchmark (also tried with -opengl4 flag, but eventually crashes as well)
Is there a way to disable newly added animals?
Much thanks in advance!
The Environment class with main loop to measure FPS:
Settings.json: settings.txt
Crash log: log.txt