Closed CodeSwimBikeRunner closed 5 years ago
Hi there! Unfortunately we don't plan to update the ANGLE NuGet package anymore. I strongly recommend that you build ANGLE directly from the master ANGLE repository, where you'll pick up the latest and greatest features/fixes: https://opensource.google.com/projects/angle
If you still have trouble building then I suggest contacting the master ANGLE repository's mailing list here: https://groups.google.com/forum/#!forum/angleproject
Thanks Austin
I am trying to build a cross platform game, using the OpenGL ES 2.0 spec/libraries. Windows 10 UWP should be supported, so I am not sure what I am doing wrong that is causing tons of errors around GL objects/methods not being defined.
I have tried two methods of importing "angle" first, I added the nuget package, and added the package include directory in the project. Then I tried cloning the repo and just adding the include directory. Both of them yield the same series of errors.
Here is an example output.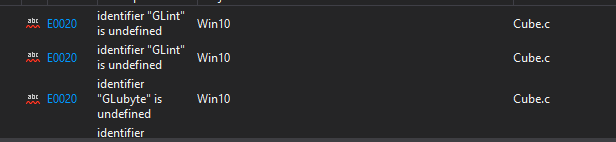
Here is my code.
float _rotation;
static GLint vertices[][3] = { { -0x10000, -0x10000, -0x10000 }, { 0x10000, -0x10000, -0x10000 }, { 0x10000, 0x10000, -0x10000 }, { -0x10000, 0x10000, -0x10000 }, { -0x10000, -0x10000, 0x10000 }, { 0x10000, -0x10000, 0x10000 }, { 0x10000, 0x10000, 0x10000 }, { -0x10000, 0x10000, 0x10000 } };
static GLint colors[][4] = { { 0x00000, 0x00000, 0x00000, 0x10000 }, { 0x10000, 0x00000, 0x00000, 0x10000 }, { 0x10000, 0x10000, 0x00000, 0x10000 }, { 0x00000, 0x10000, 0x00000, 0x10000 }, { 0x00000, 0x00000, 0x10000, 0x10000 }, { 0x10000, 0x00000, 0x10000, 0x10000 }, { 0x10000, 0x10000, 0x10000, 0x10000 }, { 0x00000, 0x10000, 0x10000, 0x10000 } };
GLubyte indices[] = { 0, 4, 5, 0, 5, 1, 1, 5, 6, 1, 6, 2, 2, 6, 7, 2, 7, 3, 3, 7, 4, 3, 4, 0, 4, 7, 6, 4, 6, 5, 3, 0, 1, 3, 1, 2 };
void Cube_setupGL(double width, double height) { // Initialize GL state. glDisable(GL_DITHER); glHint(GL_PERSPECTIVE_CORRECTION_HINT, GL_FASTEST); glClearColor(1.0f, 0.41f, 0.71f, 1.0f); glEnable(GL_CULL_FACE); glShadeModel(GL_SMOOTH); glEnable(GL_DEPTH_TEST);
}
void Cube_tearDownGL() { }
void Cube_update() { _rotation += 1.f;
} void Cube_prepare() { glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); }
void Cube_draw() { glMatrixMode(GL_MODELVIEW); glLoadIdentity(); glTranslatef(0, 0, -3.0f); glRotatef(_rotation * 0.25f, 1, 0, 0); // X glRotatef(_rotation, 0, 1, 0); // Y
}
ifndef HelloCubeNativemain__
define HelloCubeNativemain__
include
ifdef ANDROID
include <GLES/gl.h>
elif APPLE
include <OpenGLES/ES1/gl.h>
elif WINDOWS
include "GLES2/gl2.h"
include "GLES2/gl2ext.h"
include "GLES2/gl2platform.h"
include "GLES2/gl2ext_angle.h
endif
void Cube_setupGL(double width, double height); void Cube_tearDownGL(); void Cube_update(); void Cube_prepare(); void Cube_draw();
endif / defined(HelloCubeNativemain__) /