Closed MarcoAntonino closed 6 years ago
This is a very specific question unfortunately, it may be hard to find someone experienced with this.
@MarcoAntonino did you find a solution?
Hi @JasonSowers I found a workaround but I'm not so satisfied.
I'm using the google API for Calendars (no OAuth). But there are a couple of limitations: first they works only with public calendars and second I'm pretty sure the you can only read this calendars so you cannot add, modify or delete events.
For what I need it's ok, but It would be great if I could use the OAuth.
Here's the guide that I followed for the API: link
System Information
Bot Info As registered in the Bot Developer Portal at https://dev.botframework.com
Issue Description
Hello, I'm trying to create a bot that can show user the events on his Google Calendar. When the user writes the "cal" command, the bot shows all the events. When I run the bot on the localhost, this works perfectly. But when I send the command "cal" on the remote server on AppHarbor, I get this message: Request to 'https://calbot00.apphb.com/api/messages' failed: [500] Internal Server Error" and this serviceUrl.
As you can see, I'm writing every step on text file called "ex.txt". With the "command read" I print every line of the ex.txt file on the bot dialog. Reading ex.txt, the last comment on ex.txt is "UserCredential credential created" so I guess that there are some problems in the part of the code that starts with
using (var stream = new FileStream(
For the Google Calendar Api I'm following this guideCode Example
Expected Behavior
Sendig the command "cal", the bot should print all the events in a google calendar. This in my localhost, works
Actual Results
On the left the behavior in localhost, on the right the issue in remote server.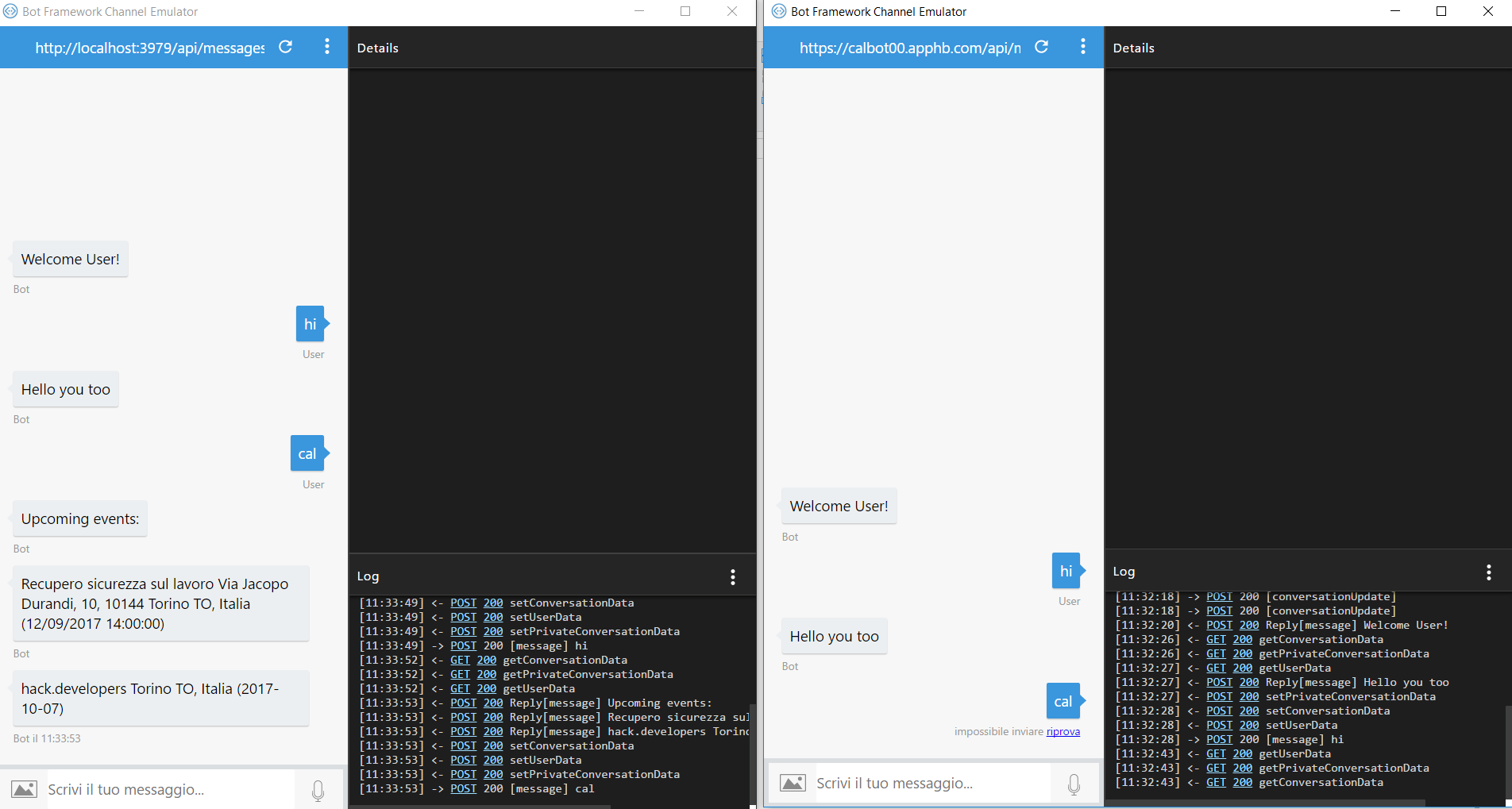
Thanks for the help, Marco