Closed hungdao-testing closed 2 years ago
You only see logs from the test runner process, all tests run in separate worker processes and if you want to see their output printed to the runner console you'll need to plumb it manually via onStdOut and onStdErr listener methods:
onStdOut(chunk: string | Buffer, test: void | TestCase, result: void | TestResult): void {
console.log(`onStdOut: ${chunk}`);
}
onStdErr(chunk: string | Buffer, test: void | TestCase, result: void | TestResult): void {
console.log(`onStdErr: ${chunk}`);
}
File appender on the other hand should work just fine as both test runner and worker processes can write to it.
Context:
"log4js": "^6.4.6",
Code Snippet log_utils.ts
test_listener.ts
global_hook
test file
playwright.config.ts
Describe the bug
When the test is triggered, only the defined log on the test_listener file and the global_hook files run, but not for the spec and the other files (as authentication file,...)
Here is the output, you guys could see there is no lines related to the test file and the others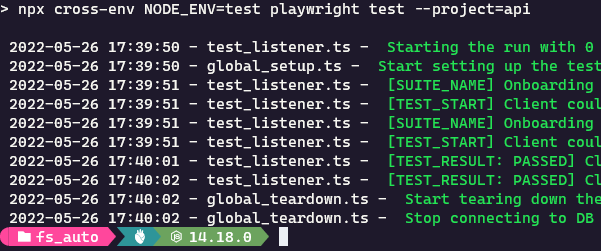