Open rustyhectors opened 1 year ago
I can confirm that with the following:
// test.mjs
import puppeteer from 'puppeteer';
(async () => {
const browser = await puppeteer.launch({
executablePath: '/Users/maxschmitt/Library/Caches/ms-playwright/chromium-1048/chrome-mac/Chromium.app/Contents/MacOS/Chromium',
});
while (true) {
console.log("connecting")
const browser2 = await puppeteer.connect({
browserWSEndpoint: browser.wsEndpoint(),
});
browser2.disconnect();
}
})().catch(e => {
console.error(e);
process.exit(1);
});
the memory also grows linearly. But it grows very slowly for me, after 80k connections the Renderer reached 1GB.
For comparison it took about 2100 iterations with code I provided to get to 1GB memory in the Renderer. I also ran your puppeteer code and confirmed I saw about the same number of iterations to get to 1GB (~80k).
@rustyhectors it's an upstream issue, can you file it there (https://crbug.com) ?
Filed an issue here https://bugs.chromium.org/p/chromium/issues/detail?id=1418465.
We are conducting a sort of mix between a continuous and a load test. Since other tools, such as Artillery, can't represent our scenario, I'm repurposing Playwright for this somewhat. Multiple browsers are opened in tabs, and in an endless loop, a few actions are carried out in all tabs in succession. In any case, I observe with Chromium also a slight linear increase: Starting at about 2.5 GB RAM, the utilization has increased to about 7GB after a good 3 hours. With Firefox, it starts at about 5 GB, and after a significantly shorter period of time, it has reached 8GB. Very noticeable is the behavior with Safari. Here is the utilization shortly after the test began:
@yury-s the bug ticket filed with chrome was closed as wont-fix
. What should we do with this issue?
Context:
Code Snippet
Help us help you! Put down a short code snippet that illustrates your bug and that we can run and debug locally. For example:
Describe the bug Chrome memory usage grows in the "renderer" process.
Baseline before running the repro: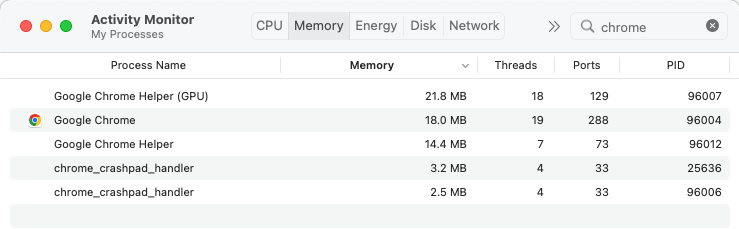
Right after starting: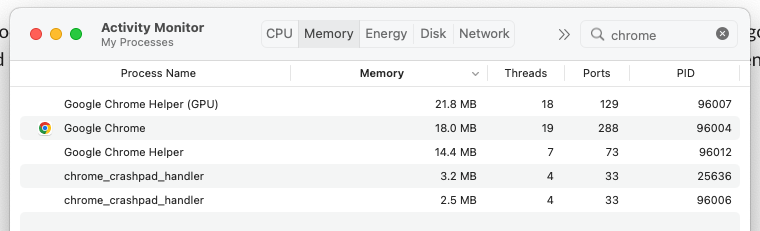
A minute or two: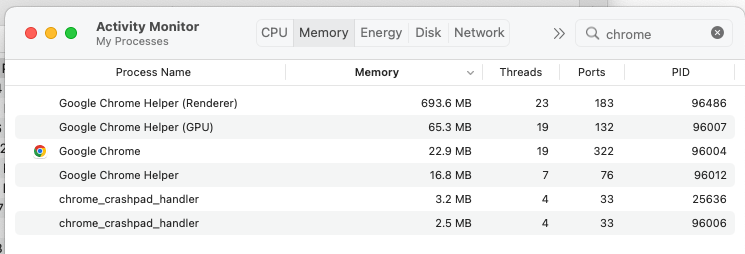
About four minutes after starting: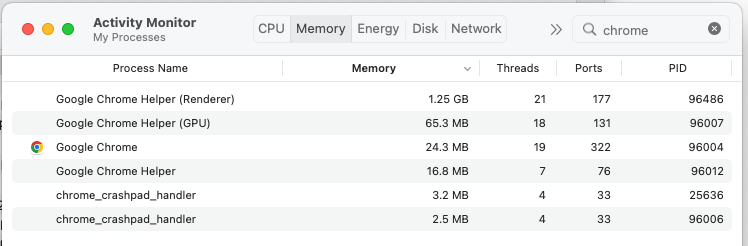