Open danieltuzes opened 3 years ago
Do you have python installed in your MinGW installation? GDB uses python for pretty printing.
If you don't have python GDB, open a shell/command and use MinGW-get.exe to 'install' Python-enabled GDB e.g. MinGw-get.exe install gdb-python 1a) Get Python 2.7.x from http://python.org/download/ and install 1b) Make sure PYTHONPATH and PYTHONHOME are set in your environment: PYTHONPATH should be C:\Python27\Lib (or similar) PYTHONHOME should be C:\Python27 1c) Add PYTHONHOME to your PATH %PYTHONHOME%;... 2a) Open a text enter, enter the following statements. Notice the 3rd line is pointing to where the python scripts are located. See notes below about this! python import sys sys.path.insert(0, 'C:/MinGW/share/gcc-4.6.1/python') from libstdcxx.v6.printers import register_libstdcxx_printers register_libstdcxx_printers (None) end 2b) Save as '.gdbinit' NOTE: Windows explorer will not let you name a file that starts with with a period from explorer. Most text edits (including Notepad) will let you. GDB init files are like 'scripts' of GDB commands that GBD will execute upon loading. 2c) The '.gdbinit' file needs to be in the working directory of GDB (most likely this is your projects root directory but your IDE can tell you.
@WardenGnaw I don't know if I have, but I have no share
nor python
folder in the MinGW folder obtained from the link mentioned above. In the corporate environment I working with, I have very limited software I am allowed to use and I am afraid that MinGW-get and its network access request are not allowed. I installed python 2.7 from an Anaconda prompt with
conda create --name py2 python=2.7
And then python 2 can be found:
(base) C:\Users\tuzes>conda activate py2
(py2) C:\Users\tuzes>where python
C:\Users\tuzes\miniconda3\envs\py2\python.exe
I prefer not to pollute my path. I'd like to add lookup directories only for the relevant environments.
Could you please give further helping hand? It is also fine if you improve the user manual of this extension and I will try to follow that.
I also found the description on GDB's Wiki how to add it, but it is not comprehensive and refers to a 12 years old e-mail. Debugging is a key priority for a language support pack like vscode-cpptools, and inspecting STL containers is widely used, so it is in the inerest of vscode-cpptools to provide a useful guide.
For Windows: If you only see memory address for STL containers when debugging, you probably used x64 Windows.
I found one valid solution, when installing MinGW in x64 Windows, install i686 (win32)
version (the bottom of this comment gives its official download link) of MinGW instead of x86_64
version, see below:
win32 version of MinGW download: i686-posix-dwarf
More details, see: https://stackoverflow.com/a/66238128/6075331
Initially posted on https://github.com/microsoft/vscode-cpptools/issues/3921#issuecomment-780379258.
For Windows: If you only see memory address for STL containers when debugging, you probably used x64 Windows.
I found one valid solution, when installing MinGW in x64 Windows, install
i686 (win32)
version (the bottom of this comment gives its official download link) of MinGW instead ofx86_64
version, see below:
win32 version of MinGW download: i686-posix-dwarf
More details, see: https://stackoverflow.com/a/66238128/6075331
Initially posted on #3921 (comment).
As I said in the section Versions: OS and Version: Windows 10 pro x64
. What you offer is a workaround at most and not in line with the official recommendations.
Do you have python installed in your MinGW installation? GDB uses python for pretty printing.
If you don't have python GDB, open a shell/command and use MinGW-get.exe to 'install' Python-enabled GDB e.g. MinGw-get.exe install gdb-python 1a) Get Python 2.7.x from http://python.org/download/ and install 1b) Make sure PYTHONPATH and PYTHONHOME are set in your environment: PYTHONPATH should be C:\Python27\Lib (or similar) PYTHONHOME should be C:\Python27 1c) Add PYTHONHOME to your PATH %PYTHONHOME%;... 2a) Open a text enter, enter the following statements. Notice the 3rd line is pointing to where the python scripts are located. See notes below about this! python import sys sys.path.insert(0, 'C:/MinGW/share/gcc-4.6.1/python') from libstdcxx.v6.printers import register_libstdcxx_printers register_libstdcxx_printers (None) end 2b) Save as '.gdbinit' NOTE: Windows explorer will not let you name a file that starts with with a period from explorer. Most text edits (including Notepad) will let you. GDB init files are like 'scripts' of GDB commands that GBD will execute upon loading. 2c) The '.gdbinit' file needs to be in the working directory of GDB (most likely this is your projects root directory but your IDE can tell you.
@WardenGnaw Did I provide the extra info you needed? You labeled this as "more info is needed". Please let me know if I can give more details.
Type: Debugger
I installed VSCode with the newest C/C++ extension, downloaded MinGW 17.1 from https://nuwen.net/mingw.html and tried to debug my CPP code, but there is either a bug, a wrong setting, or a missing feature in the debugger.
I also have MSVC but I tried to use g++ and gdb, and without installation, simply by copying them into my home directory, because in a corporate environment, one cannot use an online installer nor can install files into program files, for which one would need admin rights.
What is wrong
I cannot inspect the element of an stl container. In the example, I create a
vector<int>
, put a breakpoint into the code, and try to see its element. The debugger does not show it.Versions
Code
Use the following code and put a breakpoint within the loop:
Actual behavior
The debugger stops at the breakpoint but doesn't show the values of the vector in the VARIABLES (or Watch) tab. It only shows: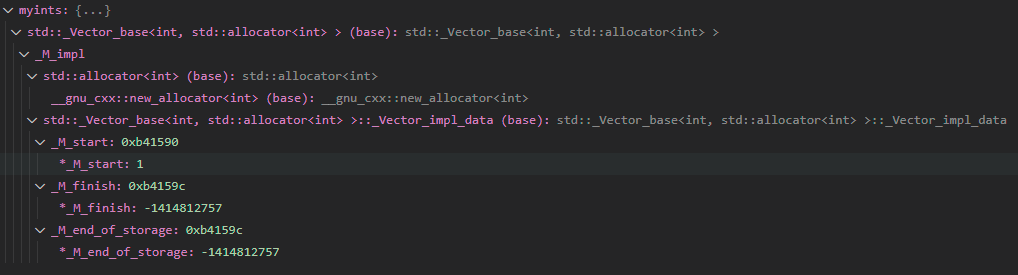
json files
lunch.json:
tasks.json:
c_cpp_properties.json: