Closed fatihgvn closed 4 years ago
Even i'm facing the same problem
The same issue
happy 9999 on all MQ7, MQ131, and MQ135. This library is promising but after implementing it i just got 9999
For this, i will be implement a new table in the next release, i will share you the demo: |ADC_In | Ecuacion_V_ADC | Voltaje_ADC | Ecuacion_R | Resistencia_RS | EQ_Ratio | Ratio (RS/R0) | Ecuacion_PPM | PPM |
Calibrations issues was fixed on new versions 1.9.9, 1.10, 2.0.
Thank you :).
Please update your library and it will solved! 👯♂
Describe the bug code in arduino
Serial Monitor
A0-A0
A0-GND
A0-VCC
Circuit Picture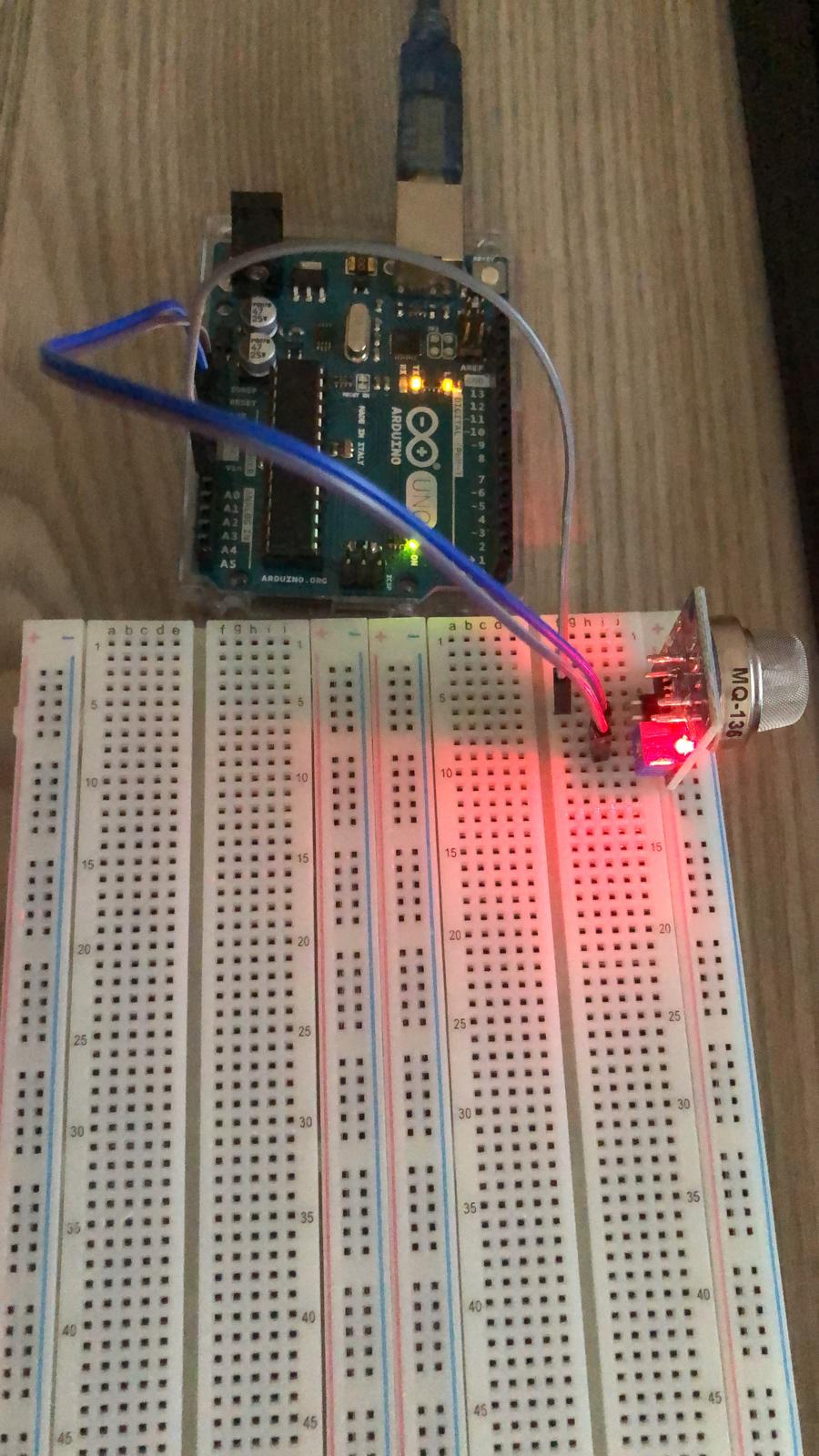
Desktop (please complete the following information):
Explanation Pin connections:
I've been trying for a few days. Where am I wrong? Only 9999.00 ppm data comes from the serial.