Closed talalz94 closed 3 years ago
Yes, this appears to be an issue in the simple-websocket package. I'll look into it.
Can I ask you to install simple-websocket from the main branch and confirm the fix? Thanks!
@miguelgrinberg Thank you for the response brother. I have a few more questions if you can kindly address them:
1) I was never using simple-websocket in the first place, I was only using flask-socketio. So why are you asking me to use simple-websocket if the issue was in this library?
2) So if I use simple-websocket instead of Flask-socketio, is the client-browser supported like the use case I have mentioned above?
I was never using simple-websocket in the first place
I see references to simple-websocket in your error stack traces, so you are most definitely using simple-websocket. This is a package that provides the websocket server to Flask-SocketIO, to you it is an indirect dependency. You don't have to change anything, just upgrade simple-websocket (which you already have installed) to the version that's on the main branch here on GitHub.
I was never using simple-websocket in the first place
I see references to simple-websocket in your error stack traces, so you are most definitely using simple-websocket. This is a package that provides the websocket server to Flask-SocketIO, to you it is an indirect dependency. You don't have to change anything, just upgrade simple-websocket (which you already have installed) to the version that's on the main branch here on GitHub.
Yes, this has fixed the problem that I was having. Many thanks :)
Just one more thing. If you dont mind commenting on my approach towards building a multi-client app using Flask-SocketIO that essentially takes in web-cam stream from multiple users simultaneoulsy and processes them. Do you think the multi-session functionality would suffice?
Do you think the multi-session functionality would suffice?
What do you mean by multi-session?
Do you think the multi-session functionality would suffice?
What do you mean by multi-session?
Sorry its not exactly multi-session, I meant I want multiple clients to connect to the same server and I just wanted to confirm my existing approach and Flask-SocketIO is compatible with that>
@talalz94 it should be fine as long as you don't store anything in the server. It seems you just accept a frame, process it and return the results, without retaining anything, correct? That is safe, as your server is stateless.
Description
I am creating a simple application that takes in web cam-stream from an in-browser client side script, converts each frame into a string format and sends it over the backend server consisting of Flask-SocketIO. The issue is that when the string length exceeds a certain length, the messages are not recieved at the backend and Im talking upto 60 kb max string and at a rate of 1 message per 5 seconds, just to test out the connection. Kindly have a look below for further details.
index.html (Client Side)
app.py (Server side)
Server Logs
Client logs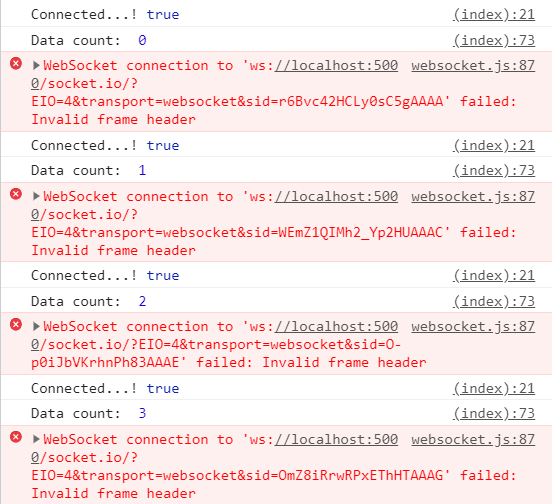
Flask-SocketIO Versions Flask-SocketIO 5.1.0 python-engineio 4.2.0 python-socketio 5.3.0
Additional Context I am running a windows machine and as I have mentioned earlier, the string length seems to be causing the issue as if I send in any string that is greater than x bytes, I get the same errors.
Looking forward to hearing from you soon. Thanks in advance :)