Closed zhucan closed 4 years ago
Incorrect usage of Go modules use import github.com/minio/minio-go/v6
@harshavardhana I had updated the deps to v6, the new test case: package main
import ( "bytes" "fmt"
"github.com/minio/minio-go/v6"
)
func main() { // Use a secure connection. ssl := false
// Initialize minio client object.
minioClient, err := minio.NewWithRegion("10.0.76.240:8060", "LGDUL089742B3WAGG3DL", "FilsJxgSOu3qFZlEHj16AC1xrCdSZCGCYn7ghwiF", ssl, "us-east-1")
if err != nil {
fmt.Println(err)
return
}
for i:=0; i<10;i++ {
err := minioClient.MakeBucket(fmt.Sprintf("pvc-%d", i), "us-east-1")
if err != nil {
fmt.Println(err)
continue
}
n, err := minioClient.PutObject(fmt.Sprintf("pvc-%d", i), "csi-fs"+"/", bytes.NewReader([]byte("")), 0, minio.PutObjectOptions{})
if err != nil {
fmt.Println(err)
continue
}
fmt.Println("Successfully uploaded bytes: ", n)
}
}
Result:
Ran this with AWS S3 no issues, looks like an issue Ceph server implementation
go run ~/test.go
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
Successfully uploaded bytes: 0
With the following code
package main
import (
"bytes"
"fmt"
"github.com/minio/minio-go/v6"
)
func main() {
// Use a secure connection.
ssl := false
// Initialize minio client object.
minioClient, err := minio.NewWithRegion("play.min.io", "minio", "minio123", ssl, "us-east-1")
if err != nil {
fmt.Println(err)
return
}
for i:=0; i<10;i++ {
err := minioClient.MakeBucket(fmt.Sprintf("pvc-%d", i), "us-east-1")
if err != nil {
fmt.Println(err)
continue
}
n, err := minioClient.PutObject(fmt.Sprintf("pvc-%d", i), "csi-fs"+"/", bytes.NewReader([]byte("")), 0, minio.PutObjectOptions{})
if err != nil {
fmt.Println(err)
continue
}
fmt.Println("Successfully uploaded bytes: ", n)
}
}
The backend can be returning error in the case of either MakeBucket
or PutObject
. You can add something extra when you print to distinguish which API is returning this error and debug further.
@harshavardhana @koolhead17 Thanks,the errors was returned by the PutObject.
@harshavardhana @kannappanr Take look at my new test case. package main
import ( "bytes" "fmt"
"github.com/minio/minio-go/v6"
)
func main() { // Use a secure connection. ssl := false
// Initialize minio client object.
minioClient, err := minio.NewWithRegion("10.0.76.240:8060", "LGDUL089742B3WAGG3DL", "FilsJxgSOu3qFZlEHj16AC1xrCdSZCGCYn7ghwiF", ssl, "us-east-1")
if err != nil {
fmt.Println(err)
return
}
for i:=0; i<10;i++ {
err := minioClient.MakeBucket(fmt.Sprintf("pvc-%d", i), "us-east-1")
if err != nil {
fmt.Println(err)
continue
}
n, err := minioClient.PutObject(fmt.Sprintf("pvc-%d", i), "csi-fs"+"/", bytes.NewReader([]byte("")), 0, minio.PutObjectOptions{})
if err != nil {
fmt.Println("put object + ", err)
continue
}
fmt.Println("Successfully uploaded bytes: ", n)
}
}
With the following code:
@zhucan this is not our bug - this looks like a bug in ceph-rgw implementation
@harshavardhana I had used s3cmd to test on it,but it's successful.
Notice you are creating a prefix /
with 0bytes here, so it's not the same reproducer.
I shall close this issue for now, please report this to Ceph tracker.
@harshavardhana hello,v4 authentication can be used "Signed payload option" ?
`When transferring payload in a single chunk, you can optionally choose to include the payload hash in the signature calculations, referred as signed payload (if you don't include it, the payload is considered unsigned). The signing procedure discussed in the following section applies to both, but note the following differences:
Signed payload option – You include the payload hash when constructing the canonical request (that then becomes part of StringToSign, as explained in the signature calculation section). You also specify the same value as the x-amz-content-sha256 header value when sending the request to S3.
Unsigned payload option – You include the literal string UNSIGNED-PAYLOAD when constructing a canonical request, and set the same value as the x-amz-content-sha256 header value when sending the request to Amazon S3.
When you send your request to Amazon S3, the x-amz-content-sha256 header value informs Amazon S3 whether the payload is signed or not. Amazon S3 can then create signature accordingly for verification.`
@harshavardhana hello,v4 authentication can be used "Signed payload option" ?
`When transferring payload in a single chunk, you can optionally choose to include the payload hash in the signature calculations, referred as signed payload (if you don't include it, the payload is considered unsigned). The signing procedure discussed in the following section applies to both, but note the following differences:
Signed payload option – You include the payload hash when constructing the canonical request (that then becomes part of StringToSign, as explained in the signature calculation section). You also specify the same value as the x-amz-content-sha256 header value when sending the request to S3.
Unsigned payload option – You include the literal string UNSIGNED-PAYLOAD when constructing a canonical request, and set the same value as the x-amz-content-sha256 header value when sending the request to Amazon S3.
When you send your request to Amazon S3, the x-amz-content-sha256 header value informs Amazon S3 whether the payload is signed or not. Amazon S3 can then create signature accordingly for verification.`
minio-go automatically does this @lsw214 https://docs.aws.amazon.com/AmazonS3/latest/API/sigv4-streaming.html
Hi @harshavardhana I am also facing this kind of error when I added minio api url as backend in terraform configs. Here is the error,
Error writing state file: failed to upload state: operation error S3: PutObject, https response error StatusCode: 400, RequestID: 17D45EE7F4D481FB, HostID: e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855, api error XAmzContentSHA256Mismatch: The provided 'x-amz-content-sha256' header does not match what was computed.
@harshavardhana
this is not our bug - this looks like a bug in ceph-rgw implementation
if the size of the object is zero, only disable it, can uploader the object with 0 size.
/open
My test case: package main
import ( "bytes" "fmt"
)
func main() { // Use a secure connection. ssl := false
}
Result: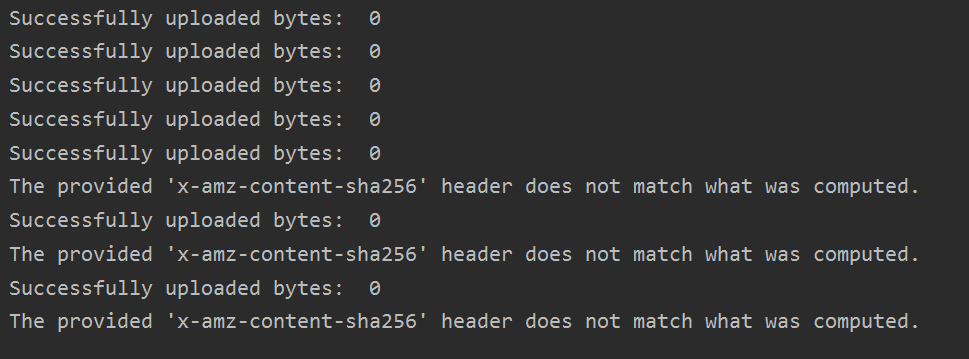
Sometimes, It's failed.