The upper part is the English version, and the lower part is the Chinese version, with the same content. If there are any wrong, or you have anything hard to understand, pls feel free to let me know.many thx.
Overview
Analyze the principle and source code of Redux-thunk
1. Invoke flow
2 How to use
in store.js
import thunk from 'redux-thunk';
const store = () => createStore(rootReducer, applyMiddleware(thunk));
function createThunkMiddleware<
State = any,
BasicAction extends Action = AnyAction,
ExtraThunkArg = undefined
(extraArgument?: ExtraThunkArg) {
// Standard Redux middleware definition pattern:
// See: https://redux.js.org/tutorials/fundamentals/part-4-store#writing-custom-middleware
const middleware: ThunkMiddleware<State, BasicAction, ExtraThunkArg> =
({ dispatch, getState }) =>
next =>
action => {
// The thunk middleware looks for any functions that were passed to `store.dispatch`.
// If this "action" is really a function, call it and return the result.
if (typeof action === 'function') {
// Inject the store's `dispatch` and `getState` methods, as well as any "extra arg"
return action(dispatch, getState, extraArgument)
}
// Otherwise, pass the action down the middleware chain as usual
return next(action)
}
return middleware
}
> The key is the line if (typeof action === 'function')
> If action is a function, invoke this function, otherwise go into the next middleware
### 4 Simplified version
> Without redux-thunk, there are only two ways to deal with the side effects of the request: 1. The View layer (ui component) sends the request first, and invoke store.dispath after getting the response data. 2. send the request in the reducer, and invoke the store.dispatch after getting the response data.
> Use redux-thunk, you can build actions that support functions
_The following is the Chinese version, the same content as above_
### Overview
> 分析redux-thunk的原理和源码
### 1. Invoke flow
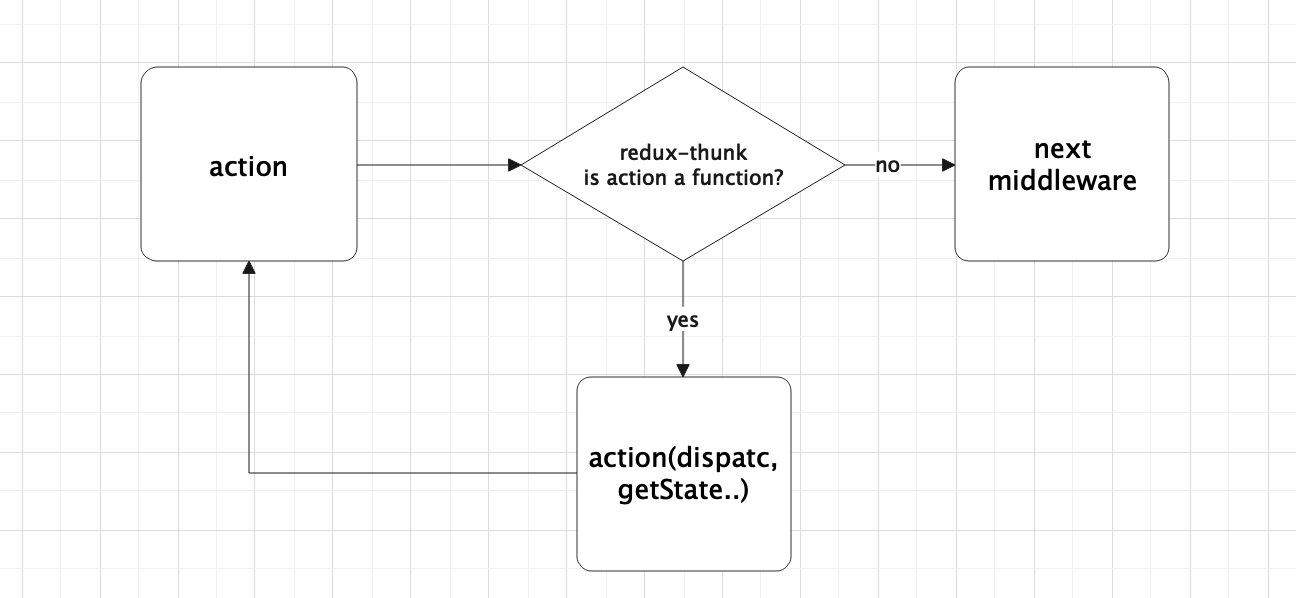
### 2 如何使用
in store.js
import thunk from 'redux-thunk';
const store = () => createStore(rootReducer, applyMiddleware(thunk));
function createThunkMiddleware<
State = any,
BasicAction extends Action = AnyAction,
ExtraThunkArg = undefined
(extraArgument?: ExtraThunkArg) {
// Standard Redux middleware definition pattern:
// See: https://redux.js.org/tutorials/fundamentals/part-4-store#writing-custom-middleware
const middleware: ThunkMiddleware<State, BasicAction, ExtraThunkArg> =
({ dispatch, getState }) =>
next =>
action => {
// The thunk middleware looks for any functions that were passed to store.dispatch.
// If this "action" is really a function, call it and return the result.
if (typeof action === 'function') {
// Inject the store's dispatch and getState methods, as well as any "extra arg"
return action(dispatch, getState, extraArgument)
}
// Otherwise, pass the action down the middleware chain as usual
return next(action)
}
The upper part is the English version, and the lower part is the Chinese version, with the same content. If there are any wrong, or you have anything hard to understand, pls feel free to let me know.many thx.
Overview
1. Invoke flow
2 How to use
in store.js
in action.js
in ui component
3. Source code
return middleware }
const thunk = ({ dispatch, getState }) => (next) => (action) => { if (typeof action === 'function') { return action(dispatch, getState); }
return next(action); }; export default thunk
import thunk from 'redux-thunk'; const store = () => createStore(rootReducer, applyMiddleware(thunk));
export const fetchTodos = () => dispatch => ( fetchTodos().then(todos => dispatch({ type: GET_TODOS, todos })) );
store.dispatch(fetchTodos())
function createThunkMiddleware< State = any, BasicAction extends Action = AnyAction, ExtraThunkArg = undefined
return middleware }
const thunk = ({ dispatch, getState }) => (next) => (action) => { if (typeof action === 'function') { return action(dispatch, getState); }
return next(action); }; export default thunk