The upper part is the English version, and the lower part is the Chinese version, with the same content. If there are any wrong, or you have anything hard to understand, pls feel free to let me know.many thx.
React does little at compile stage, only converts jsx into createElement method. So it is mainly a runtime framework, it needs to optimize performance from both CPU and IO sides to achieve the purpose of fast response
This article is based on React 17.0.2React official websiteReact source code
scripts: git、jest、prettier、eslint etc.
Most important modules are react react-dom react-reconciler scheduler
Different platforms use different modules, such as react-dom for browsers, react-art for canvas, and react-native-renderer for mobile
This is why React 17 no longer needs to import React, as it was changed to jsx()
3 How to debug
git clone https://github.com/facebook/react.git
npm install
npm run build react/index,react/jsx,react-dom/index,scheduler --type=NODE
Type the command npm link in build/node_modules/react and build/node_modules/react-dom, then type npm link react, npm link react-dom in business projects
Or directly debug by chrome devtool
Or set react, react-dom, shared, and react-reconciler pointing to the source code in webpack.config.js. The advantage of using this method is that it can directly debug the source code instead of the compiled code. The disadvantage is that some issues caused by uncomplied need to be fixed, such as the configuration of environment variables.
4 Core issues
Async interruptible updates are the goal of React performance optimizations. Scheduler (requestIdleCallback polyfill) and Fiber are key technologies to achieve it. Fiber maps every React node, it is the unit of work.
For the CPU issue, I personally think that using Web Worker + MessageChannel to optimize React rendering, in particularly at multi-core CPU environment.
What is Suspense
What is useDeferredValue
It like debounce, but it can only be used in React 18
5 Explanation of Architecture Diagram
Explain every point for the architecture diagram on the top
5.1 jsx
After jsx is compiled by babel, it is converted to createElement() or jsx(), then it returns a json object that describe dom structure. That json object is also called virtual dom.
The more bits of 1, the lower the priority
Schedule priority is used in Scheduler, Lane priority is used in React
5.2.3 Other
time slice: In Concurrent Mode, multiple tasks run in the same JS thread, and every Task can be switched between interrupted or running states, giving users the illusion of task concurrency. This is similar to the concurrency of a single-core CPU, so it is called time slicing
task scheduling: means that the tasks can be interrupted and resumed, and every task is setted a corresponding priority.
interruptible rendering: there are 2 Fiber trees in a rendering task. A Fiber tree has n Fiber nodes. One Fiber corresponds to a React node. When rendering to a Fiber node, it can be stopped, and in the future it can continue rendering from this Fiber node. That means interrupting and resuming rendering.
5.3 Reconciler
5.3.1 Fiber
workInProgress(Fiber)
//source code: https://github.com/facebook/react/blob/17.0.2/packages/react-reconciler/src/ReactInternalTypes.js
Fiber = {
// Consistent with vitual dom
tag:WorkTag, // the type of component
Key:null | string, // key property of component
elementType: any, // element type, ReactElement.type, is also the first parameter of `createElement`
type: any, // usually `function` or `class` or an element name such as 'p'
// used to build fiber tree, linked list structure
return: Fiber | null, // point to the parent node
child: Fiber | null, // points to the first child node
sibling: Fiber | null, // point to the next sibling node
index: number,
ref:null,
//Save the state, calculate the state
pendingProps: any, // new props brought by the update
memoizedProps:any //last rendered props
updateQueue:UpdateQueue<any> | null; //update queue of the Fiber
memoizedState: any // last rendered state
dependencies: Dependencies | null; //contexts and events
mode: TypeOfMode; // ConcurrentMode or LegacyMode
//effect related, linked list structure
flags: Flags, // effect Tag
nextEffect: Fiber | null, // Used to quickly find the next effect
firstEffect: Fiber | null, // first effect in subtree
lastEffect: Fiber | null, // last sibling effect in the subtree
// Priority related properties
lanes: Lanes,
childLanes: Lanes,
//Pointer to current or workInProgress
//The fiber node in the WorkInProgress tree will switch positions after rendering is complete
this.alternate = null;
}
5.3.2 Fiber tree
Fiber maps every react node and maintains node information, formed based on virtual node processing. Fiber mainly saves the properties, dom, type, etc. of this node, and using child (child node), sibling (sibling node), return (parent node), and updateQueue (for updating state), effect tag (for committing to dom) etc. properties to builds a Fiber tree
5.3.3 Two Fiber trees
React uses Double Buffering to maintain two Fiber trees. WorkInProgress Fiber is the Fiber tree that is being updated, both updated Fiber nodes and unupdated Fiber nodes in it. After workInProgress Fiber constructed, it will be switch to current Fiber, and finally committed to dom. WorkInProgress Fiber is just an intermediate variable in memory.current Fiber and workInProgress Fiber refer to each other by alertnate. The switch is performed by a property named 'current' of fiberRootNode, when current points to workInProgress Fiber, which means the switch is completed.
During mount, build a workInProgress Fiber based on jsx object, then switch workInProgress Fiber to current Fiber, finally commit to dom; during update, build a new workInProgress Fiber based on state-changed jsx object and current Fiber, and then switch it to current Fiber, finally commit to dom
5.4 Renderer
commit to dom: Iterate effect list built on render stage, every Fiber node in effect list saves the own changes of properties, then commit to real dom.
The following is the Chinese version, the same content as above
The upper part is the English version, and the lower part is the Chinese version, with the same content. If there are any wrong, or you have anything hard to understand, pls feel free to let me know.many thx.
1 Directory
Export API for react directory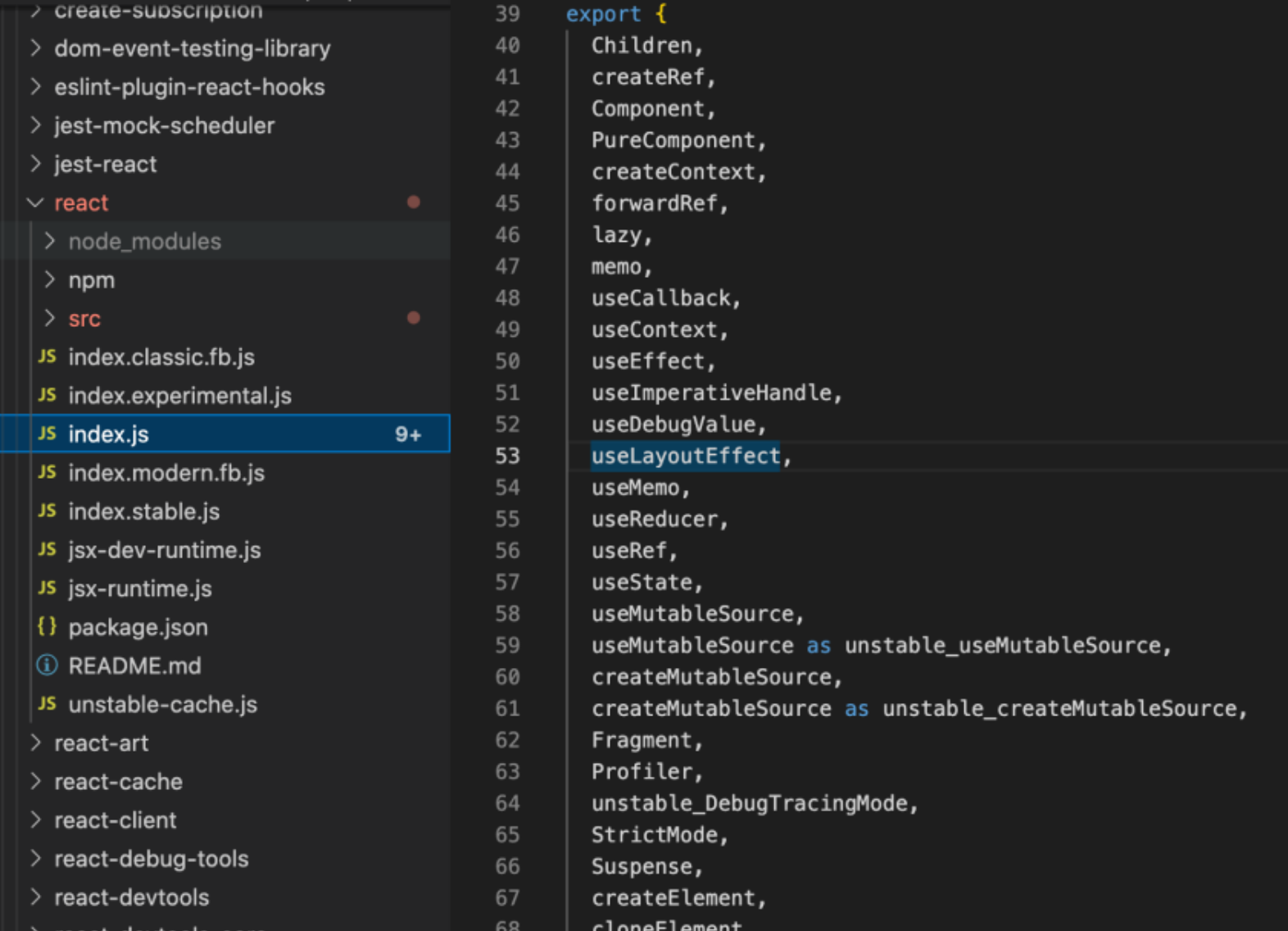
For web side, it requires the following 3 modules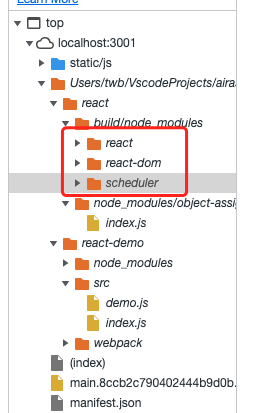
2 Entrance
2.1 Entrance
2.2 Compilation of JSX
2.2.1 Old compilation
2.2.2 New compilation
3 How to debug
4 Core issues
What is Suspense
What is useDeferredValue
It like debounce, but it can only be used in React 18
5 Explanation of Architecture Diagram
5.1 jsx
5.2 Scheduler
5.2.1 Schedule
5.2.1.1 requestIdleCallback polyfill
5.2.1.2 Defects of requestIdleCallback
FPS out of sync
It's browser compatibility is not as good as requestAnimationFrame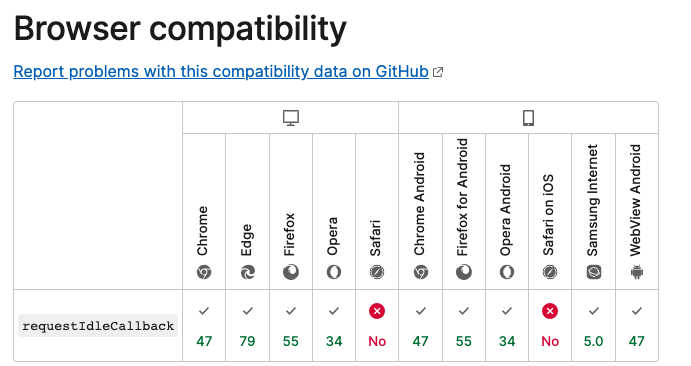
5.2.2 Priority
Schedule priorities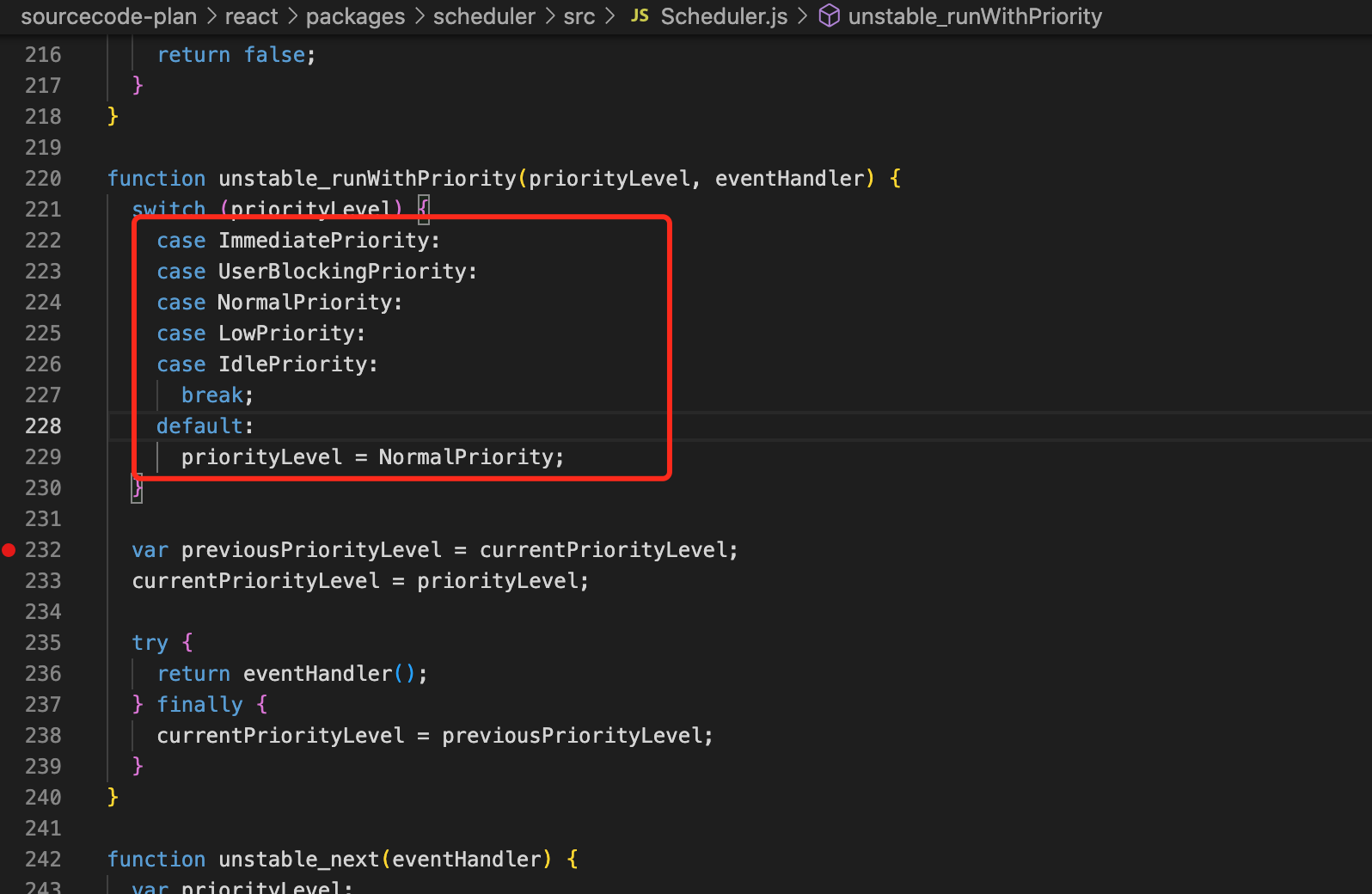
Lane priorities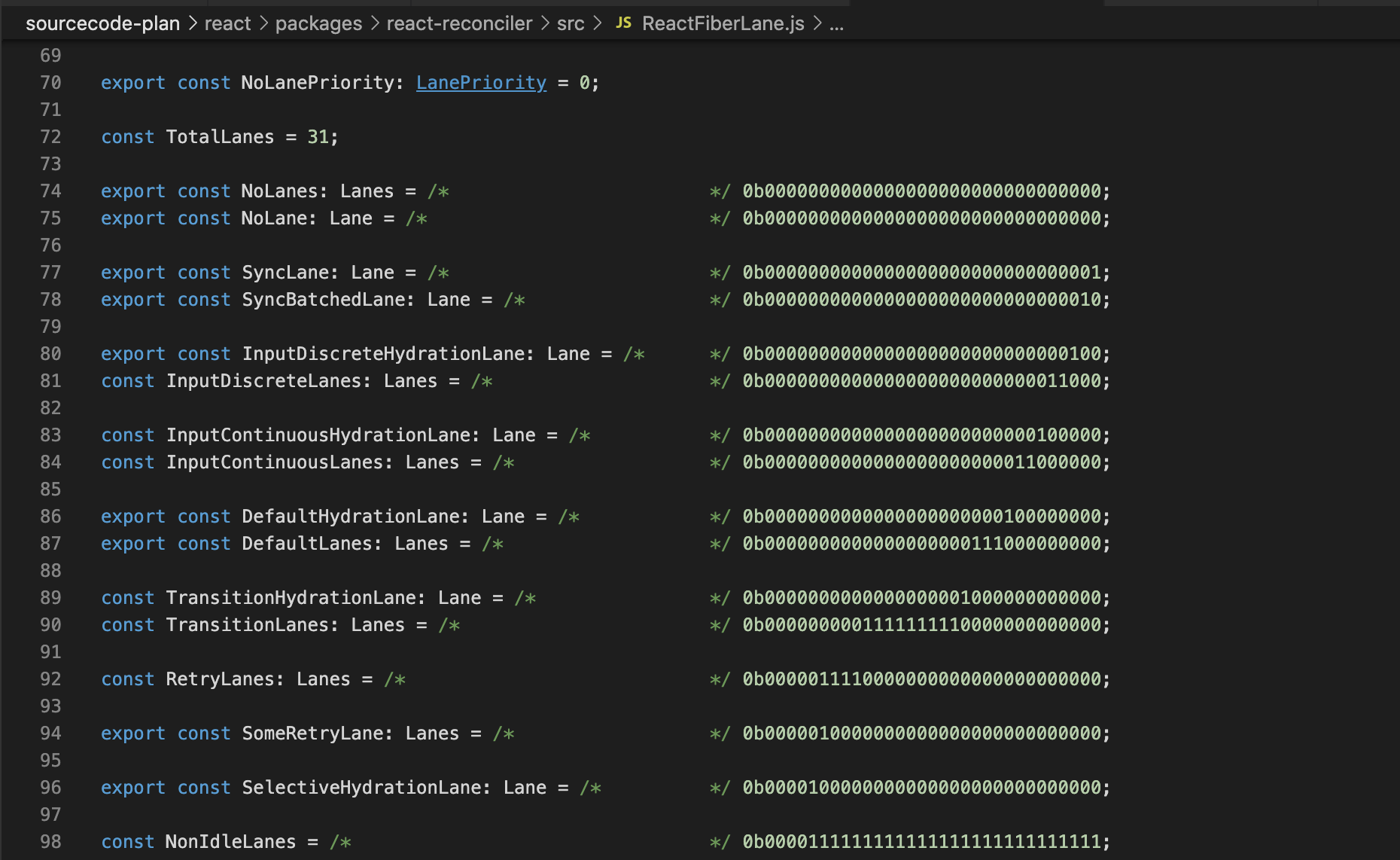
5.2.3 Other
time slicing
5.3 Reconciler
5.3.1 Fiber
workInProgress(Fiber)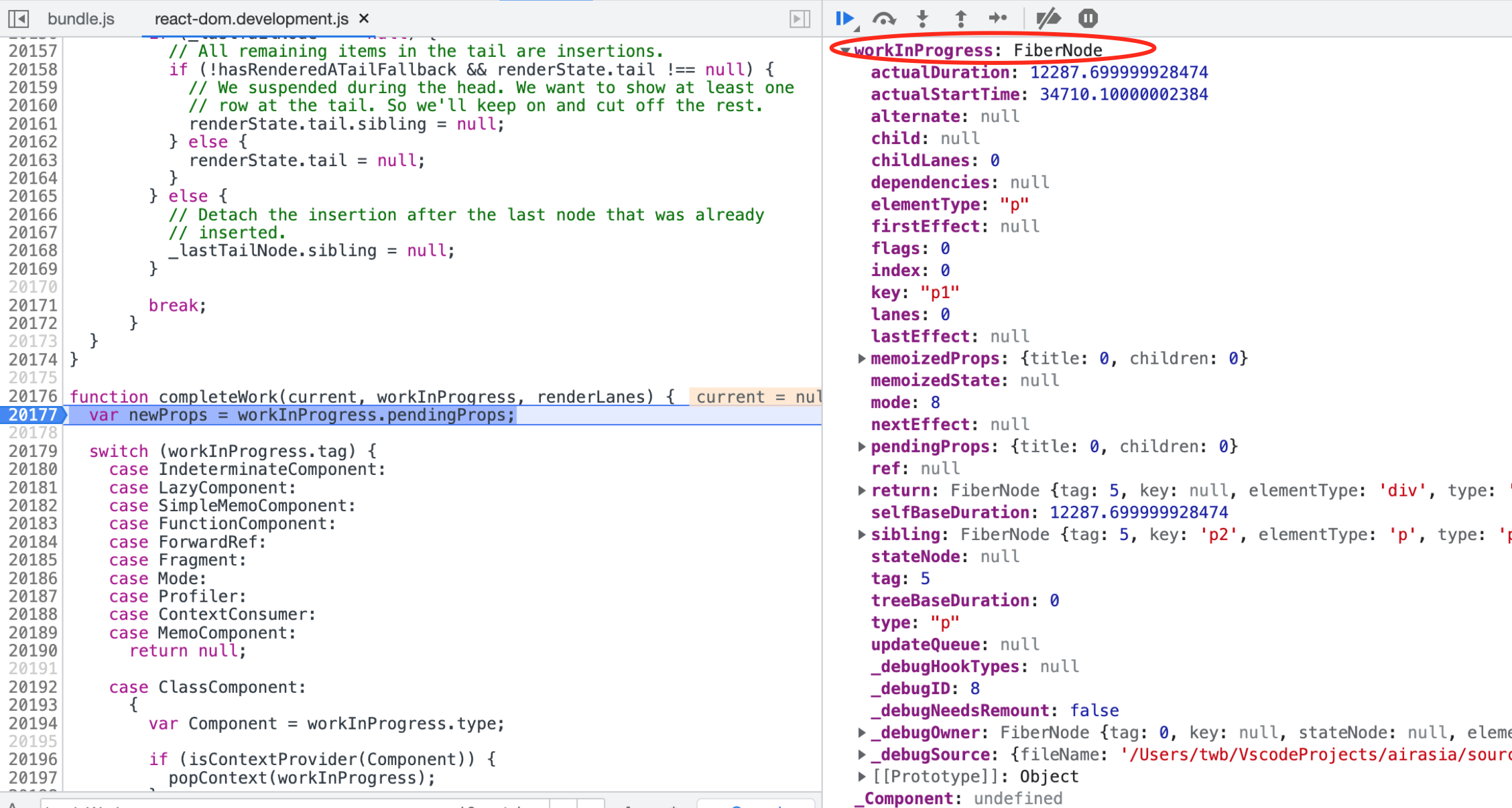
5.3.2 Fiber tree
5.3.3 Two Fiber trees
5.4 Renderer
The following is the Chinese version, the same content as above
1 目录
react目录的API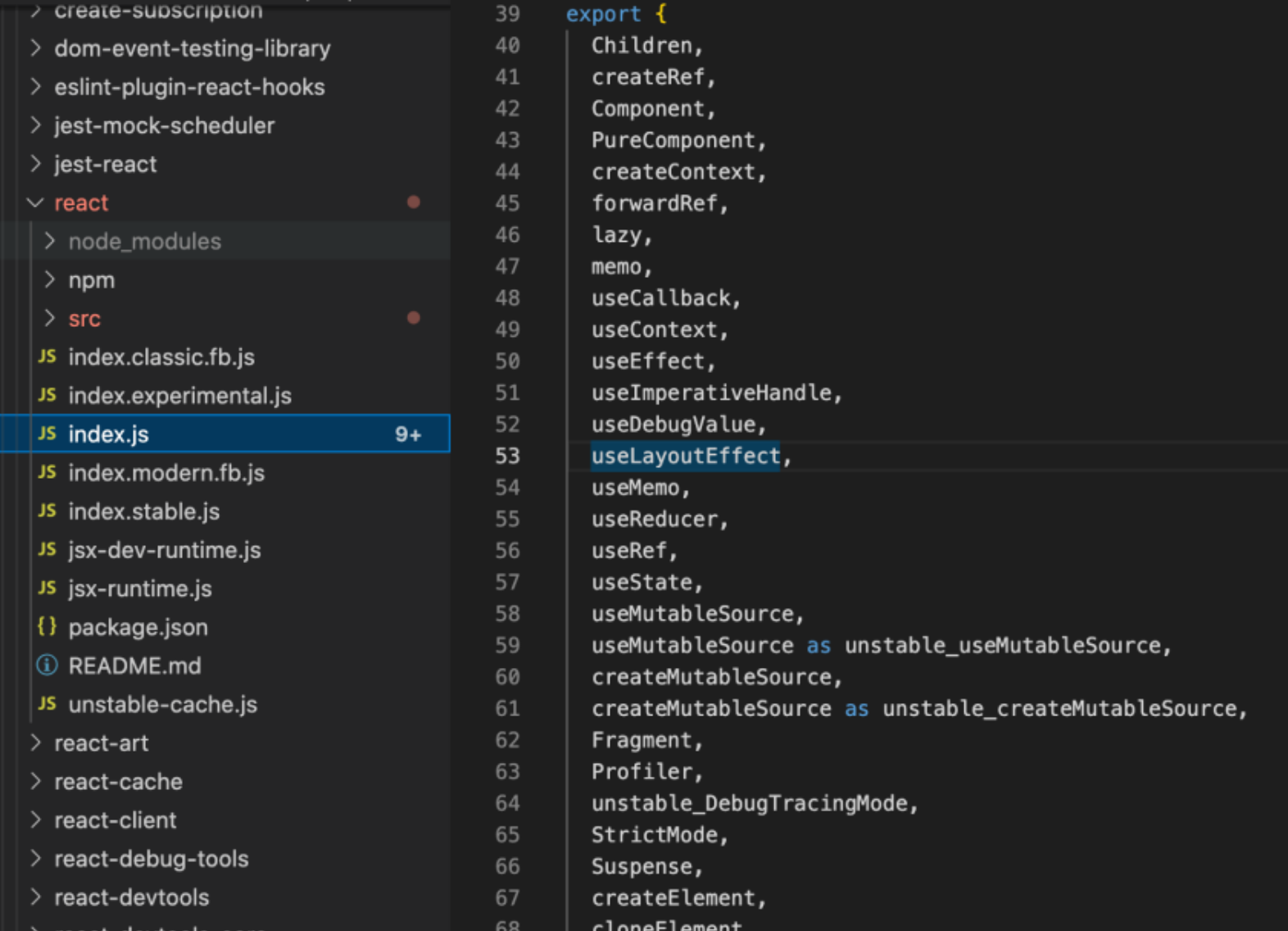
web端需要的核心就3个包
2 入口
2.1 入口
2.2 JSX的编译
2.2.1 旧的编译
2.2.2 新的编译
3 如何调试
4 核心问题
What is Suspense
What is useDeferredValue
It like debounce, but it can only be used in React 18
5 架构图的解释
5.1 jsx
5.2 Scheduler
5.2.1 调度
5.2.1.1 requestIdleCallback polyfill
5.2.1.2 requestIdleCallback 的缺陷
FPS不同步
浏览器兼容性不如requestAnimationFrame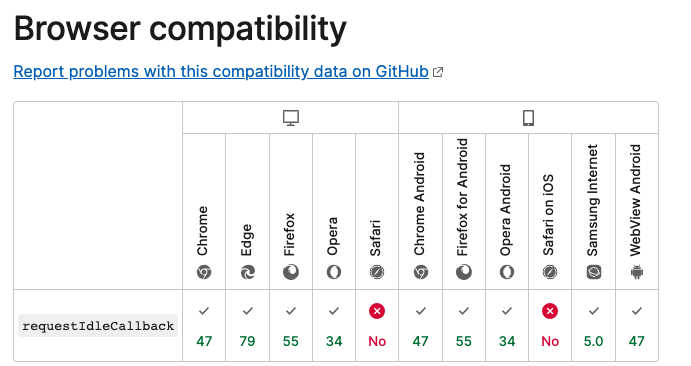
5.2.2 优先级
Schedule优先级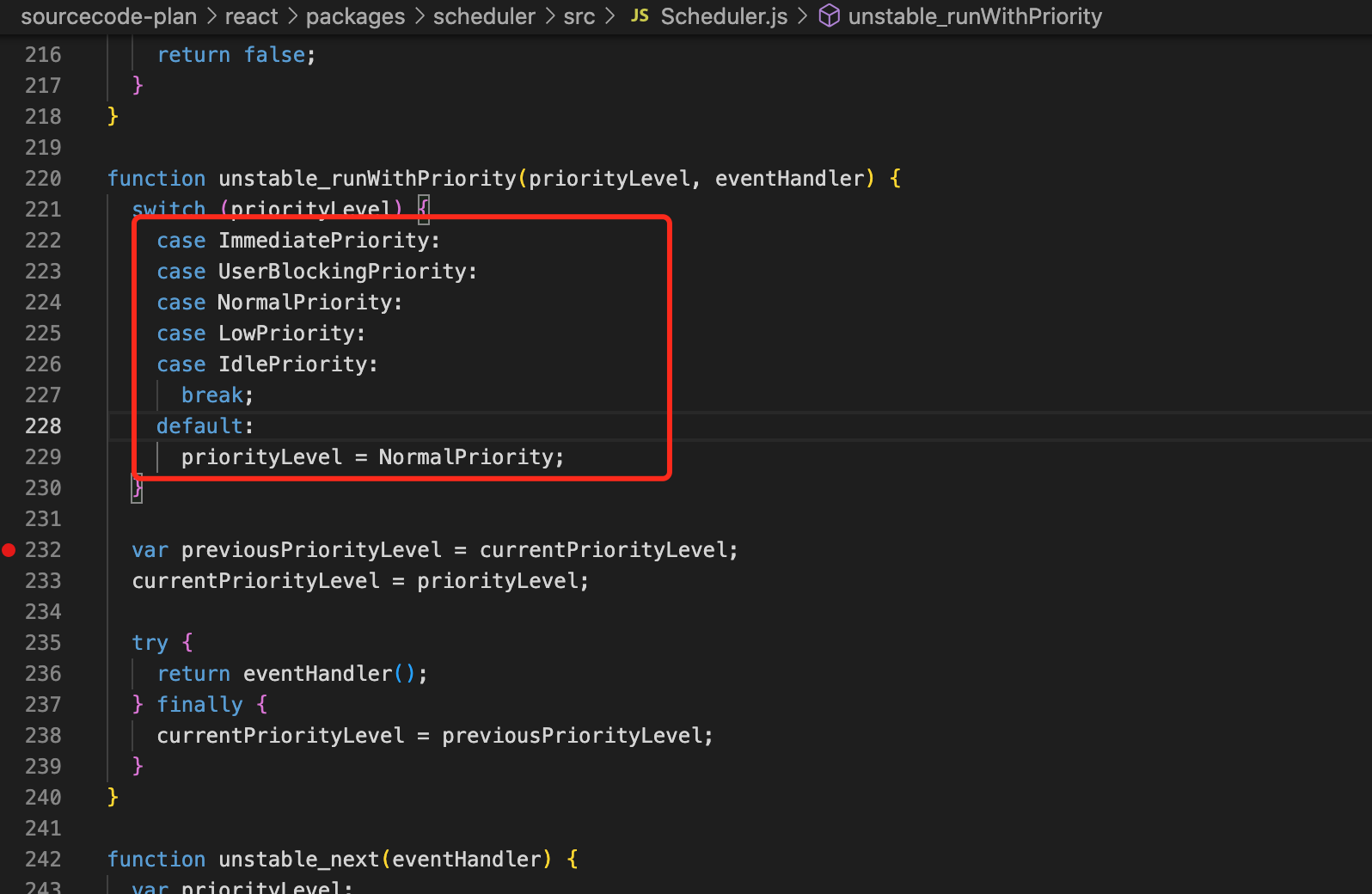
Lane优先级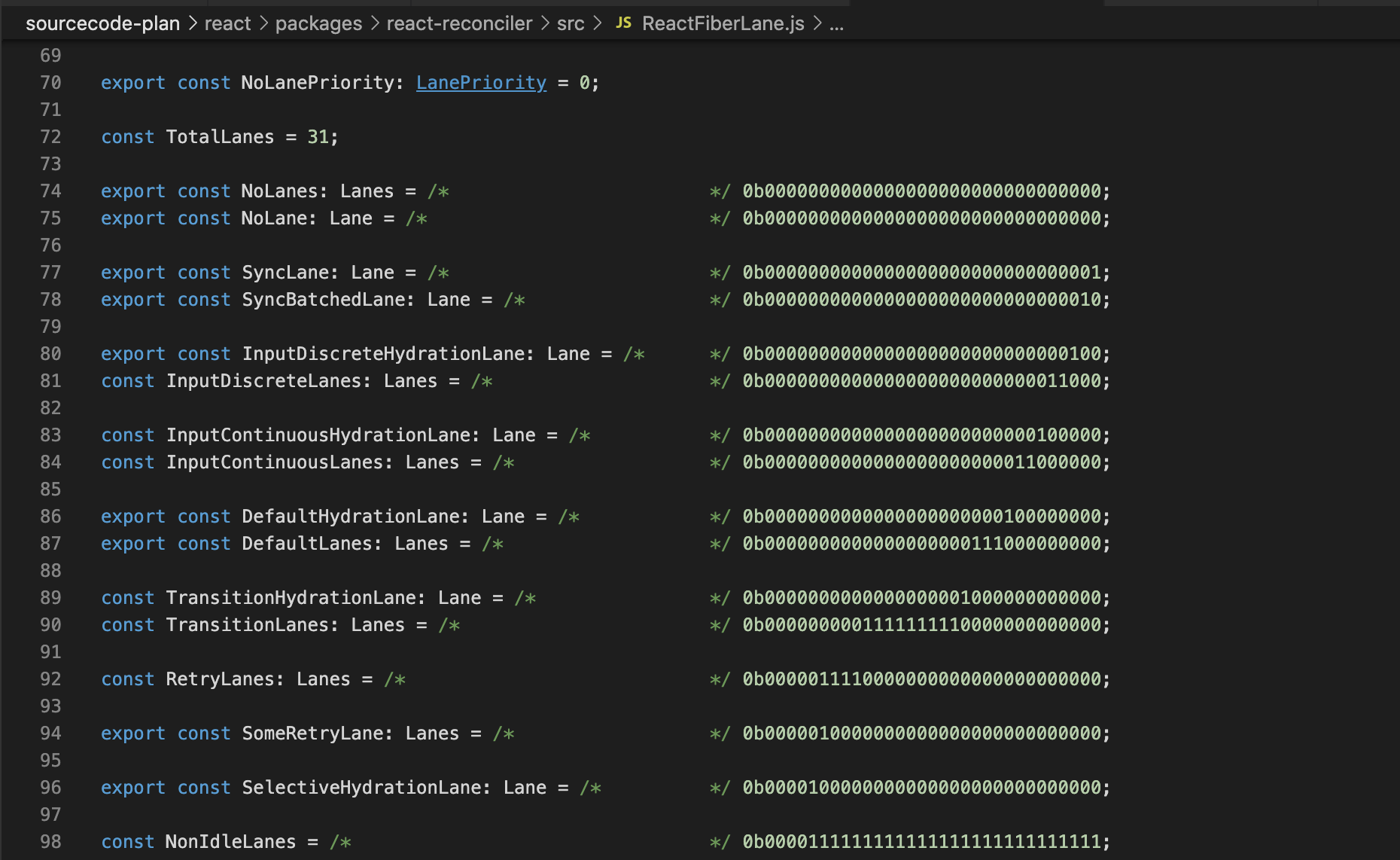
5.2.3 其他
5.3 Reconciler
5.3.1 Fiber
workInProgress(Fiber)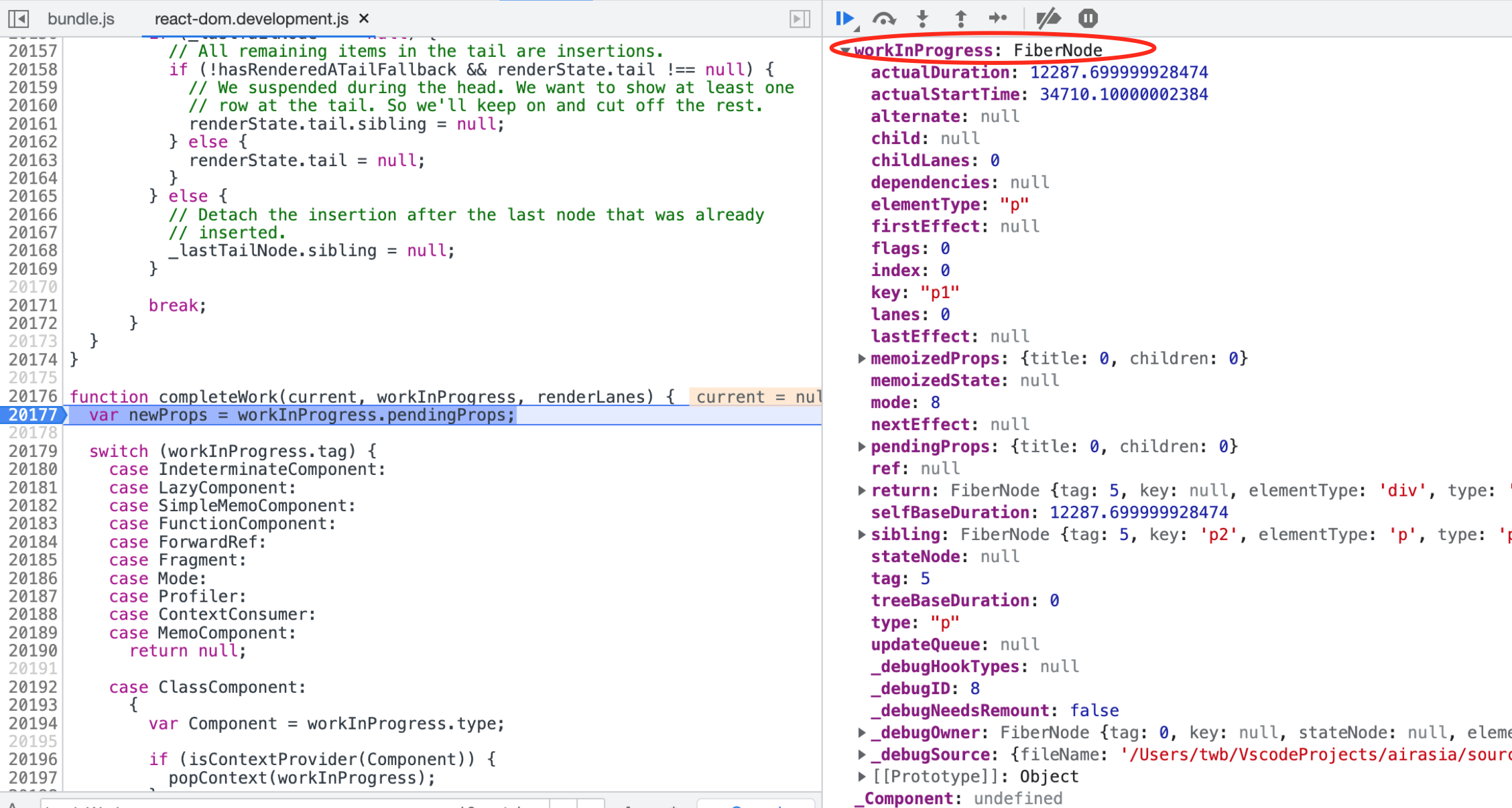
5.3.2 Fiber tree
5.4 Renderer