The upper part is the English version, and the lower part is the Chinese version, with the same content. If there are any wrong, or you have anything hard to understand, pls feel free to let me know.many thx.
This document discusses a suitable state management solution for WeChat Mini Programs. After comparing various options, the Proxy solution is recommended, and a detailed analysis of the Proxy solution is provided.
2 Comparison of Different Solutions
Solution
Advantages
Disadvantages
Redux
Clear state management
Large library size, increases the app size Complex configuration Suitable for medium to large projects
MobX
Simple reactive programming
Large library size, increases app size Suitable for medium and large projects, especially those needing a lot of reactive updates
Global Data
Built-in support in WeChat Mini Programs Easy to use
Does not support asynchronous updates
Event Bus
Loose coupling between components, flexible event-based communication
Complex state management, hard to maintain Suitable for small projects with complex event flows
Proxy
Automatic response to state changes Simple code implementation
A large number of proxy objects may affect performance Suitable for small applications
Since most WeChat Mini Programs are small applications, the Proxy solution is recommended. However, if there are too many proxy objects, consider other solutions like MobX.
3 Proxy Solution Design
3.1 Core Source Code Reference
class Store {
instance = null;
callBackList = {};
constructor() {
this.createInstance();
}
createInstance() {
if (!this.instance) {
// init dataSource
const dataSource = {};
this.instance = new Proxy(dataSource, {
set: (target, key, value, receiver) => {
const result = Reflect.set(target, key, value, receiver);
if (this.callBackList[key]) {
// execute every callback for the corresponding field
Object.values(this.callBackList[key]).flat().forEach(callback => callback(value));
}
return result;
}
});
}
return this.instance;
}
get(key) {
return this.instance[key];
}
set(key, value) {
this.instance[key] = value;
}
subscribe(key, callback, componentInstance) {
const id = componentInstance.is;
if (!this.callBackList[key]) {
this.callBackList[key] = {};
}
if (!this.callBackList[key][id]) {
this.callBackList[key][id] = [];
}
this.callBackList[key][id].push(callback);
}
unsubscribe(key, componentInstance) {
const id = componentInstance.is;
if (this.callBackList[key] && this.callBackList[key][id]) {
delete this.callBackList[key][id];
}
}
}
export default new Store();
Note: The above solution uses the Proxy API, which requires the WeChat Mini Program version to be >= 2.11.0.
If you need to support lower versions of the Mini Program, please use the source code below.
class Store {
callBackList = {}; // save callbacks
data = {}; // save states
constructor() {}
get(key) {
return this.data[key];
}
set(key, value) {
this.data[key] = value;
if (this.callBackList[key]) {
const callbacks = Object.values(this.callBackList[key]).reduce(
(acc, cur) => acc.concat(cur),
[]
);
callbacks.forEach(callback => callback(value));
}
}
subscribe(key, callback, componentInstance) {
// mark every component
const id = componentInstance.is;
if (!this.callBackList[key]) {
this.callBackList[key] = {};
}
if (!this.callBackList[key][id]) {
this.callBackList[key][id] = [];
}
this.callBackList[key][id].push(callback);
}
unsubscribe(key, componentInstance) {
const id = componentInstance.is;
if (this.callBackList[key] && this.callBackList[key][id]) {
delete this.callBackList[key][id];
}
}
}
export default new Store();
// Set value synchronously
Store.set('userInfo', newUserInfo);
// Update synchronously
this.setData({
userInfo: Store.get('userInfo')
});
3.4 Asynchronous Updates
// Asynchronous update
// Subscribe to changes in userInfo
Store.subscribe('userInfo', (newUserInfo) => {
this.setData({
userInfo: newUserInfo
});
}, this);
// Unsubscribe when the page is unloaded
onUnload() {
Store.unsubscribe('userInfo', this);
}
3.5 Modularization
Consider creating state modules, especially for information frequently used throughout the project, such as user profile information or i18n.
For example, user profile information:
// Subscribe
ProfileStore.subscribe(this.updateProfile, this)
// Unsubscribe
ProfileStore.unsubscribe(this)
// Set value
ProfileStore.set(profile)
// Get value
ProfileStore.get()
The following is the Chinese version, the same content as above
The upper part is the English version, and the lower part is the Chinese version, with the same content. If there are any wrong, or you have anything hard to understand, pls feel free to let me know.many thx.
1 Overview
This document discusses a suitable state management solution for WeChat Mini Programs. After comparing various options, the Proxy solution is recommended, and a detailed analysis of the Proxy solution is provided.
2 Comparison of Different Solutions
Complex configuration
Suitable for medium to large projects
Suitable for medium and large projects, especially those needing a lot of reactive updates
Easy to use
Suitable for small projects with complex event flows
Simple code implementation
Suitable for small applications
Since most WeChat Mini Programs are small applications, the Proxy solution is recommended. However, if there are too many proxy objects, consider other solutions like MobX.
3 Proxy Solution Design
3.1 Core Source Code Reference
Note: The above solution uses the Proxy API, which requires the WeChat Mini Program version to be >= 2.11.0.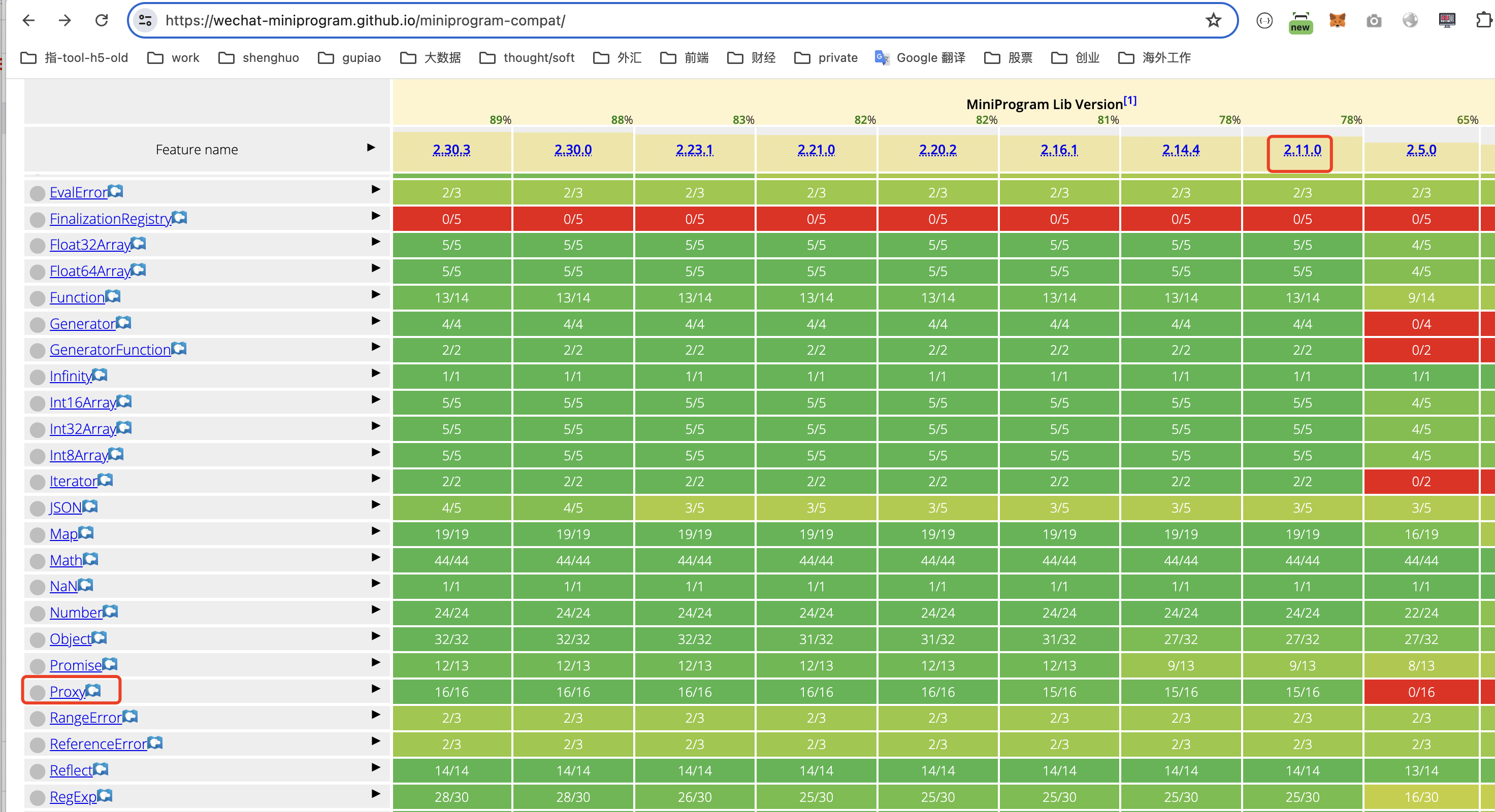
If you need to support lower versions of the Mini Program, please use the source code below.
3.2 Unified Data Source
3.2.1 Data Source Structure
3.3 Synchronous Updates
3.4 Asynchronous Updates
3.5 Modularization
Consider creating state modules, especially for information frequently used throughout the project, such as user profile information or i18n. For example, user profile information:
Usage is simpler:
The following is the Chinese version, the same content as above
1 概述
本文主要针对微信小程序探讨一个合适的状态管理方案,在经过各方案对比后,推荐使用Proxy方案,并对Proxy方案进行详细的分析。
2 不同方案对比
配置繁琐
适合中大型项目
适合中型项目以上,尤其需要大量响应式更新的场景
容易使用
适合小型项目,需要复杂事件流的场景
代码实现简洁
- 适合小型应用
基于大部分微信小程序都是小型应用,推荐采用 Proxy方案。 但如果代理的对象过多,则要考虑其他方案如Mbox等。
3 Proxy 方案设计
3.1 核心源码参考
注意,上面的方案用到Proxy API, 它要求所使用的小程序的版本>=2.11.0
如果要兼容小程序低版本,请采用下面的源码
3.2 统一数据源
3.2.1 数据源结构
3.3 同步更新
3.4 异步更新
3.5 模块化
可以考虑做一些状态模块封装,尤其对整个项目都会用到的用户信息,或国际化等 比如用户信息
使用更简洁