Closed DusterTheFirst closed 4 years ago
From your image it's fairly obvious that your code is miss-interpreting the y position and/or height. This is an example of that happening (Note the similarity to your issue):
And here it is when interpreting the y/height from fontdue correctly:
Note: The y coordinate is the bottom edge of the glyph, and height expands upwards. These are the relevant docs:
pub struct GlyphPosition {
/// Hashable key that can be used to uniquely identify a rasterized glyph.
pub key: GlyphRasterConfig,
/// The left side of the glyph bounding box. Dimensions are in pixels, and are alawys whole
/// numebrs.
pub x: f32,
/// The bottom side of the glyph bounding box. Dimensions are in pixels and are always whole
/// numbers.
pub y: f32,
/// The width of the glyph. Dimensions are in pixels.
pub width: usize,
/// The height of the glyph. Dimensions are in pixels.
pub height: usize,
}
Feel free to comment on this issue if you have more questions.
&GlyphPosition
s and rasterizing them withrasterize_config
.Sample code:
produces: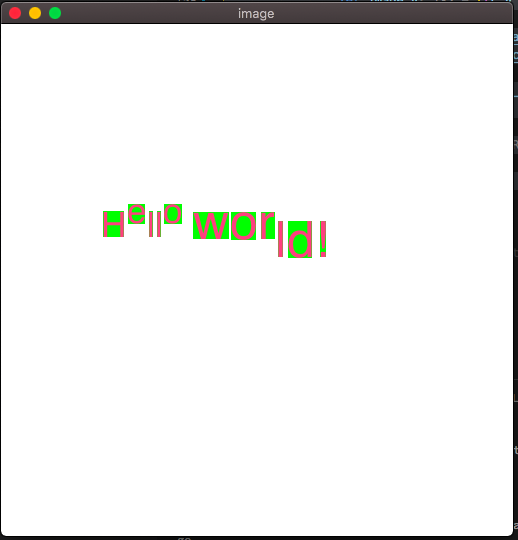