Closed trusktr closed 9 months ago
const material = new THREE.MeshPhysicalMaterial({ color: 'royalblue' }) points = new THREE.Points(geometry, material) // iOS Safari glitches
MeshPhysicalMaterial is not designed to be used with Points - you must use "PointsMaterial".
Ah, indeed. I was going for that shimmering effect. I can fallback to PointsMaterial on iOS.
I see this is marked as "Help (please use the forum)". Should people post in the forum first, then if something is actually a bug post it here?
Just curious because it wasn't obvious if the above was a bug or not. I figured switching to gl.POINTS
would effectively not make any difference (merely change the fragments on screen), and it worked great everywhere except specifically iOS Safari.
Should people post in the forum first, then if something is actually a bug post it here?
Yes
The issue arise when not specifying gl_PointSize
in the vertex shader on some GPUs @trusktr.
If you wanted to be consistant with your visual on iOS you could also use onBeforeCompile
and specify gl_PointSize
with the same code as in points.glsl.js
.
Description
iOS Safari not rendering
Points
properly. I have a feeling this is a bug in Safari.Reproduction steps
It looks like this:
Click to view screenshot
It looks like this:
Click to view screenshot
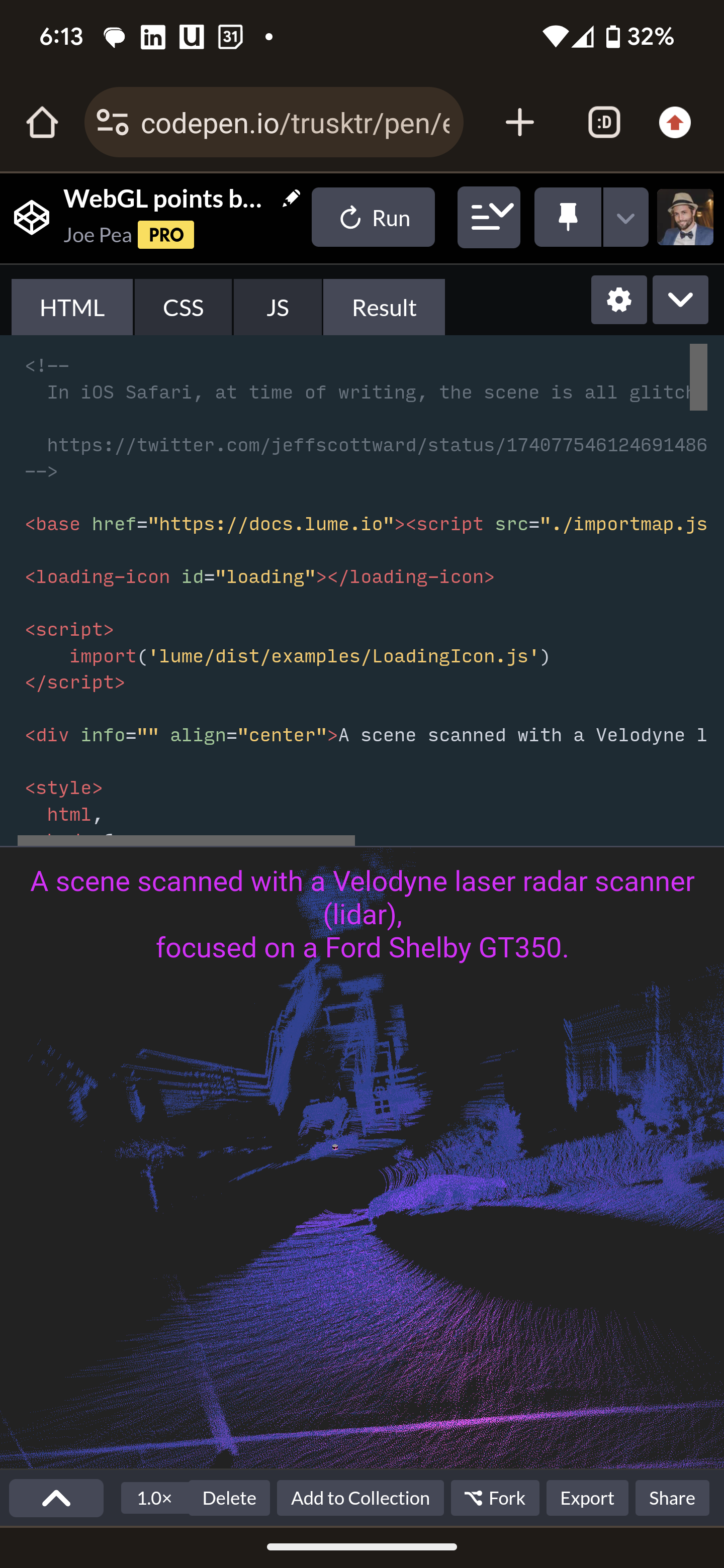Code
Live example
Screenshots
See reproduction
Version
r158 (at time of writing, see https://docs.lume.io/modules/three/package.json)
Device
Mobile
Browser
Safari
OS
iOS