Closed SahilMahadwar closed 1 year ago
I have tried everything at this point even solutions from here: vercel/next.js#15965 but nothing seems to work
here is my code
import React, { useState, useRef, useEffect } from 'react'; import RecordRTC, { invokeSaveAsDialog } from 'recordrtc'; export default function Test() { const [stream, setStream] = useState(null); const [blob, setBlob] = useState(null); const refVideo = useRef(null); const recorderRef = useRef(null); const handleRecording = async () => { const mediaStream = await navigator.mediaDevices.getDisplayMedia({ video: { width: 1920, height: 1080, frameRate: 30, }, audio: false, }); setStream(mediaStream); recorderRef.current = new RecordRTC(mediaStream, { type: 'video' }); recorderRef.current.startRecording(); }; const handleStop = () => { recorderRef.current.stopRecording(() => { setBlob(recorderRef.current.getBlob()); }); }; const handleSave = () => { invokeSaveAsDialog(blob); }; useEffect(() => { if (!refVideo.current) { return; } // refVideo.current.srcObject = stream; }, [stream, refVideo]); return ( <div className="App"> <header className="App-header"> <button onClick={handleRecording}>start</button> <button onClick={handleStop}>stop</button> <button onClick={handleSave}>save</button> {blob && ( <video src={URL.createObjectURL(blob)} controls autoPlay ref={refVideo} style={{ width: '700px', margin: '1em' }} /> )} </header> </div> ); }
error
@SahilMahadwar This error is because Next.js is a SSR framework and URL only construct on Client side. That means URL is undefined on Server. The simple hack is that wrap your video logic into a component and import that component with Next/dynamic with {ssr: false} that means component will only import on client side nor on Nextjs Server.
In my case it helps me to resolve this blocker.
const VideoRecordingDialog = dynamic(() => import('../VideoRecordingDialog'), { ssr: false });
@ahmedraza11 It worked thanks :)
I have tried everything at this point even solutions from here: https://github.com/vercel/next.js/discussions/15965 but nothing seems to work
here is my code
error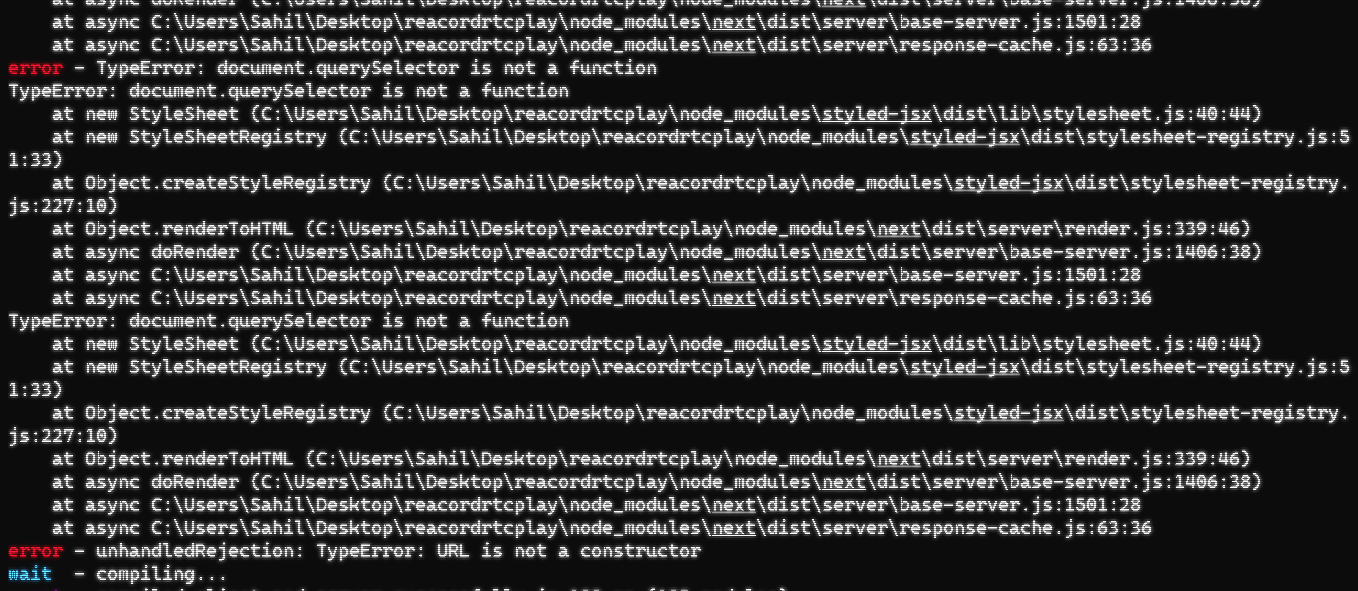