The beginning of my first trading bot in the Python programming language with the assistance of AI
In light of the entrance of ChatGPT and soon to be others, my goal of creating an incredibly successful, automated trading bot will be marked by three milestones:
1) The creation of a simple, Python-based trading bot that can turn a profit in both paper and live trading.
2) The integration of additional Python-based strategies, signals, trade secrets, etc. for greater profit potential.
3) The conversion of strategies from other programming languages (e.g., Pine Script) into viable Python code for a trading bot that is both strategic and comprehensive in scope and design for maximum profitability in live trading, specifically.
We’re going to be using the following library: backtrader.pyBacktrader.py will allow us to do backtesting, as well as live trading.
In ChatGPT, we must start with the following prompt: Do you know what backtrader.py is?
It has an obvious response and I won’t bore you w/ that here.
For the next prompt, I asked: Can you please create a trading strategy in Python by using backtrader.py to trade Apple stock, based on 5 years historical data?
ChatGPT generated the following code and response:
import backtrader as bt
import backtrader.indicators as btind
class SmaCross(bt.Strategy):
params = (("fast", 50), ("slow", 200),)
def __init__(self):
sma_fast = btind.SimpleMovingAverage(self.data.close, period=self.params.fast)
sma_slow = btind.SimpleMovingAverage(self.data.close, period=self.params.slow)
self.crossover = btind.CrossOver(sma_fast, sma_slow)
def next(self):
if not self.position:
if self.crossover > 0:
self.buy()
else:
if self.crossover < 0:
self.sell()
cerebro = bt.Cerebro()
data = bt.feeds.YahooFinanceData(dataname='AAPL', fromdate='2018-01-01', todate='2023-01-01')
cerebro.adddata(data)
cerebro.addstrategy(SmaCross)
cerebro.run()
In this example, the SmaCross strategy buys when the fast simple moving average crosses above the slow simple moving average and sells when the fast simple moving average crosses below the slow simple moving average. The params class attribute is used to specify the values of the fast and slow moving average periods. The next method implements the logic for making trades based on the crossover of the fast and slow moving averages. The Cerebro class is used to manage the trading strategy and data, and the YahooFinanceData class is used to import the data.
The code (and written response) generated above differs in a few ways from the video at the YouTube URL, however I will continue on w/ the basic strategy given, and will adjust, accordingly.
Next prompt: YahooFinanceData is not available anymore, do you have other options for historical data sources for Apple stock?
We were offered Quandl, as one of the sources, but a code was generated for another source. I asked ChatGPT to change the data source to Quadl. It generated the following code:
import backtrader as bt
import backtrader.indicators as btind
class SmaCross(bt.Strategy):
params = (("fast", 50), ("slow", 200),)
def __init__(self):
sma_fast = btind.SimpleMovingAverage(self.data.close, period=self.params.fast)
sma_slow = btind.SimpleMovingAverage(self.data.close, period=self.params.slow)
self.crossover = btind.CrossOver(sma_fast, sma_slow)
def next(self):
if not self.position:
if self.crossover > 0:
self.buy()
else:
if self.crossover < 0:
self.sell()
cerebro = bt.Cerebro()
data = bt.feeds.Quandl(dataname='EOD/AAPL',
fromdate='2018-01-01',
todate='2023-01-01',
api_key='<YOUR_API_KEY>',
compression=1)
cerebro.adddata(data)
cerebro.addstrategy(SmaCross)
cerebro.run()
Note: You'll need to replace with your own API key obtained from Quandl.
At this point, we must open account w/ Quandl, which was recently acquired by NASDAQ.
5:29 Mark in the Video: Signup for an account at: https://data.nasdaq.com/
I will be provided w/ my API key immediately upon account creation.
6:50 Mark in the Video: Get Visual Studio Code and let’s run the code there.
7:33 Mark in the Video: Copy/paste my API key into Visual Studio Code, as directed.
Be advised, the video says to add the Python extension to our version of Visual Studio Code. The latest version of Visual Studio Code, instead, will prompt us to be installed the moment we add the main.py file to our folder.
With the Python extension successfully installed into VS Code, a play-button appear towards the top-right corner of the VS Code platform. It makes it more convenient for us to test code.
8:26 Mark: Press the PLAY button.
8:35 Mark: We need to install backtrader module, but before we do…
8:40 Mark: We must create the virtual environment.
In VS Code at the prompt, type “python3 –m venv chatgpt_env”.
Note: We can use any name for the environment. Now we have our environment.
And this last command is where I ran into a problem.
See directly below:
According to my research, I had to restart Visual Studio Code to let the updates take effect. It worked and I got past a significant series of problems.
After also adding the requirements.txt file in the root directory at your instruction, I am now at an impasse at the 10:53 mark in the video.
The snapshot below is what I see when I run the latest version of the code:
Here is a copy of the Python code as it stands at the moment:
import pip
pip.__version__ = "10.0.1"
import backtrader as bt
import backtrader.indicators as btind
class SmaCross(bt.Strategy):
params = (("fast", 50), ("slow", 200),)
def __init__(self):
sma_fast = btind.SimpleMovingAverage(self.data.close, period=self.params.fast)
sma_slow = btind.SimpleMovingAverage(self.data.close, period=self.params.slow)
self.crossover = btind.CrossOver(sma_fast, sma_slow)
def next(self):
if not self.position:
if self.crossover > 0:
self.buy()
else:
if self.crossover < 0:
self.sell()
cerebro = bt.Cerebro()
data = bt.feeds.Quandl(dataname='EOD/AAPL',
fromdate='2018-01-01',
todate='2023-01-01',
api_key='Ue5ZXwiprG4dVywqsSxf',
compression=1)
cerebro.adddata(data)
cerebro.addstrategy(SmaCross)
cerebro.run()
Python has issues with this code and it did NOT work.
OK, a VERY cool development! It looks like I got the code in VS Studio to work and there are no more issues showing up there!
However, when I run the program, I get a message that ends like this:
quandl.errors.quandl_error.NotFoundError: (Status 404) (Quandl Error QECx02) You have submitted an incorrect Dataset code. Please check your Dataset codes and try again.
I ran it through ChatGPT and it said I should check my Quandl code to make sure it is still correct or valid. (I'm presuming this is the API code, which I checked on the website. It's correct.)
I then saw a portion of the NASDAQ site (which acquired Quandl) and it says that only a subset of the API data is available and that I need a subscription to get the full data.
For a running Trading Bot, it would totally be worth it to me, within reason of course!
Is subscribing to this service on the NASDAQ site the next step in your opinion? I don't mind spending some money to get a step closer to passive, exponential income!
Here is the URL to the site which pitches the subscription: https://data.nasdaq.com/databases/RTAT/usage/quickstart/api
2-24-23 Latest news:
I did it! I slept on it and the answer came to me as I was dozing. I made one little change in the dataname and hit Play. It returned a Final Portfolio Value and I even made a profit! (It wasn't much, but it is a profit nonetheless. Most importantly, I created something that actually works!) :) <---------- First Time Software Developer
2-26-23 Latest news:
The good news - I think I successfully pushed my Python code to Github under the project named "TradingBot2".
Is this bad news? - (See copied/pasted pic directly below.)
Took this snapshot below of GitBash also for your perusal after pushing my project (successfully, I hope!)
3-1-23 Latest news:
I tried to push my code tonight and um, might have messed something up.
During my attempt, I got this message below. And now I'm stuck.
I asked The New Bing what Git Bash was trying to tell me and this is what it said:
Thank you for sharing the text. Here is what it means:
3-6-23 Latest news:
I (think I) attempted to push my files into my local py-trader directory today. (Insert smiley face here) However during Step 6 as outlined in your latest message to me on Discord, I got the following response from Git Bash after trying to push AND trying to use GPT3 to assist me after that:
We're almost there! I continue to study some neat strategies to consider for the creation of my first Python-coded Trading Bot also. Any thoughts on this new problem as depicted in the pic above?
The beginning of my first trading bot in the Python programming language with the assistance of AI
In light of the entrance of ChatGPT and soon to be others, my goal of creating an incredibly successful, automated trading bot will be marked by three milestones: 1) The creation of a simple, Python-based trading bot that can turn a profit in both paper and live trading. 2) The integration of additional Python-based strategies, signals, trade secrets, etc. for greater profit potential. 3) The conversion of strategies from other programming languages (e.g., Pine Script) into viable Python code for a trading bot that is both strategic and comprehensive in scope and design for maximum profitability in live trading, specifically.
Milestone 1:
I will initially utilize this YouTube video: https://www.youtube.com/watch?v=-1B2cxSjeGw
How to use ChatGPT to get started building my trading bot:
ChatGPT generated the following code and response:
In this example, the SmaCross strategy buys when the fast simple moving average crosses above the slow simple moving average and sells when the fast simple moving average crosses below the slow simple moving average. The params class attribute is used to specify the values of the fast and slow moving average periods. The next method implements the logic for making trades based on the crossover of the fast and slow moving averages. The Cerebro class is used to manage the trading strategy and data, and the YahooFinanceData class is used to import the data.
Next prompt: YahooFinanceData is not available anymore, do you have other options for historical data sources for Apple stock? We were offered Quandl, as one of the sources, but a code was generated for another source. I asked ChatGPT to change the data source to Quadl. It generated the following code:
Note: You'll need to replace with your own API key obtained from Quandl.
8:35 Mark: We need to install backtrader module, but before we do… 8:40 Mark: We must create the virtual environment.
Note: We can use any name for the environment. Now we have our environment.
And this last command is where I ran into a problem.
See directly below:
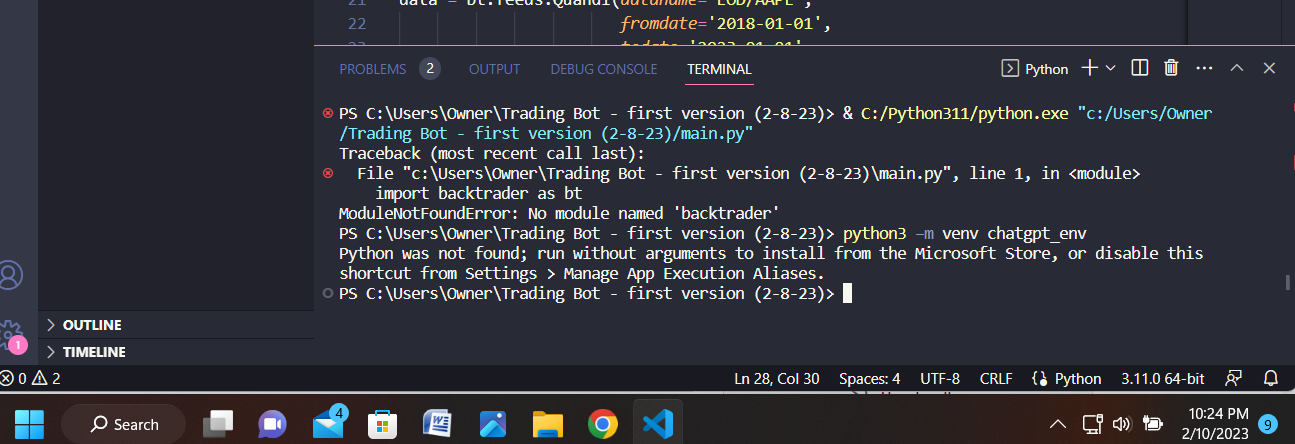
In VS Code at the prompt, type “python3 –m venv chatgpt_env”.
According to my research, I had to restart Visual Studio Code to let the updates take effect. It worked and I got past a significant series of problems. After also adding the requirements.txt file in the root directory at your instruction, I am now at an impasse at the 10:53 mark in the video. The snapshot below is what I see when I run the latest version of the code: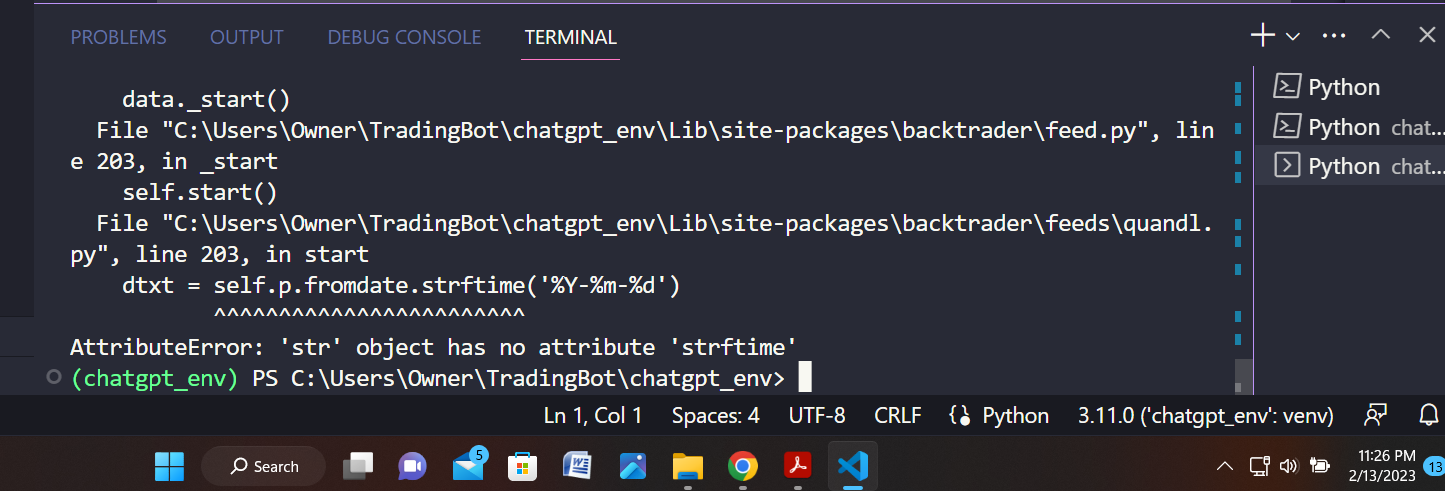
Here is a copy of the Python code as it stands at the moment:
Latest news: I tried integrating Gitbash with VS Code and I found the instructions at this URL:
https://www.geeksforgeeks.org/how-to-integrate-git-bash-with-visual-studio-code/
Python has issues with this code and it did NOT work.
OK, a VERY cool development! It looks like I got the code in VS Studio to work and there are no more issues showing up there! However, when I run the program, I get a message that ends like this:
quandl.errors.quandl_error.NotFoundError: (Status 404) (Quandl Error QECx02) You have submitted an incorrect Dataset code. Please check your Dataset codes and try again.
I ran it through ChatGPT and it said I should check my Quandl code to make sure it is still correct or valid. (I'm presuming this is the API code, which I checked on the website. It's correct.) I then saw a portion of the NASDAQ site (which acquired Quandl) and it says that only a subset of the API data is available and that I need a subscription to get the full data. For a running Trading Bot, it would totally be worth it to me, within reason of course! Is subscribing to this service on the NASDAQ site the next step in your opinion? I don't mind spending some money to get a step closer to passive, exponential income!
Here is the URL to the site which pitches the subscription: https://data.nasdaq.com/databases/RTAT/usage/quickstart/api
2-24-23 Latest news: I did it! I slept on it and the answer came to me as I was dozing. I made one little change in the dataname and hit Play. It returned a Final Portfolio Value and I even made a profit! (It wasn't much, but it is a profit nonetheless. Most importantly, I created something that actually works!) :) <---------- First Time Software Developer
2-26-23 Latest news: The good news - I think I successfully pushed my Python code to Github under the project named "TradingBot2". Is this bad news? - (See copied/pasted pic directly below.)
Took this snapshot below of GitBash also for your perusal after pushing my project (successfully, I hope!)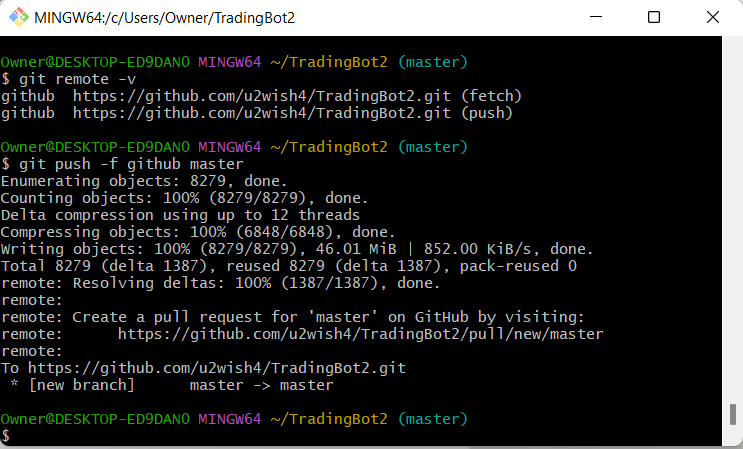
3-1-23 Latest news: I tried to push my code tonight and um, might have messed something up.
During my attempt, I got this message below. And now I'm stuck.
I asked The New Bing what Git Bash was trying to tell me and this is what it said: Thank you for sharing the text. Here is what it means:
git init reinitializes an existing Git repository in your project folder1. git add . adds all the files in your project folder to the staging area, which means they are ready to be committed12. git commit -m "TradingBot2 Push 3-1-23" creates a snapshot of the changes you made and saves it to the Git directory with a message12. On branch master Your branch is up to date with 'github/master'. nothing to commit, working tree clean means that you are on the master branch of your repository, which is synchronized with your Github repository, and that you have no changes to commit since your last commit3.
3-6-23 Latest news: I (think I) attempted to push my files into my local py-trader directory today. (Insert smiley face here) However during Step 6 as outlined in your latest message to me on Discord, I got the following response from Git Bash after trying to push AND trying to use GPT3 to assist me after that:
We're almost there! I continue to study some neat strategies to consider for the creation of my first Python-coded Trading Bot also. Any thoughts on this new problem as depicted in the pic above?