Closed Aldrin-John-Olaer-Manalansan closed 4 years ago
Which version of the library? Latest master?
Also, it seems to me that a bit is stuck sometimes when reading a byte, can you sequentially send every integer until 255 and see how that turns out?
Which version of the library? Latest master?
Hello @Avamander yes I am using the latest version. I just chmod the library using this steps 8 hours ago and I got those results.
Also, it seems to me that a bit is stuck sometimes when reading a byte, can you sequentially send every integer until 255 and see how that turns out?
As advised, I recorded a sample of sending an array of byte from 0 up to 255. and it turns out that the reception of raspberry-pi is really not stable. try watching the video.
I only changed the following esp32 code:
#include <SPI.h>
#include "RF24.h"
RF24 radio(25, 26);
byte __private_channel__[5] = {76, 224, 23, 108, 172};
void setup() {
// put your setup code here, to run once:
Serial.begin(115200);
radio.begin();
radio.openWritingPipe(__private_channel__);
radio.setChannel(97);
radio.setPALevel(RF24_PA_MAX);
radio.printDetails();
radio.stopListening();
}
void loop() {
static byte value=0;
byte message[32];
memset(message,value,32);
if (value>=255)
value=0;
else value++;
if (radio.write(message,32))
{
Serial.println("Message:");
Serial.println((char*)message);
for (byte i=0;i<32;i++)
Serial.print(' '+String(message[i]));
Serial.println();
}
else
Serial.println("failed");
delay(250);
}
Please try the bundled gettingStarted
example and see if the issue still persists.
Generally though I suspect this to be an issue with your wiring on the rPi, by any chance is it connected trough a ribbon cable to the module?
Please try the bundled
gettingStarted
example and see if the issue still persists.Generally though I suspect this to be an issue with your wiring on the rPi, by any chance is it connected trough a ribbon cable to the module?
The issue DOES NOT persist on the gettingStarted example. Only at the pingpair_dyn.py and at my raspberrypi.py script. I suspect that the issue happens when a full 32 byte payload is being recovered. the more unstable the library becomes?
I am using the latest Raspberry-Pi 4B, I used 10cm DuPont connecting wires(ribbon cable) to wire nrf24 to rpi and my wiring was:
NRF24 Raspberry-Pi 4B MOSI - GPIO10(SPI0_MOSI) MISO - GPIO09(SPI0_MISO) CLK - GPIO11(SPI0_CLK) CE - GPIO22(pin no 15) CSN - GPIO07(SPI0_CE1) VCC - 3v3(pin no 17) GND - Ground(pin no 25)
Also, I tested CSN connected to CE0 before and it produces the same wrong values when connecting CSN to CE1.
If the issue doesn't exist in gettingStarted
then it's time to figure out what modification in your code causes the issue. There are quite a few things you can configure, at a quick glance I noticed you changed the channel and pipes, try changing those back and test then.
You can also try experimenting with changing CRC (length), disabling/enabling dynamic payloads, disabling/enabling auto-ACK and adding ceramic capacitor to the power pins of the nRF24. Max power when modules are near each other might also not be a good idea.
There are also clone modules that cause very weird issues in certain configurations, make sure you don't have one of those. There's quite a lot of information about those available online.
If the issue doesn't exist in
gettingStarted
then it's time to figure out what modification in your code causes the issue. There are quite a few things you can configure, at a quick glance I noticed you changed the channel and pipes, try changing those back and test then.
Even with default channel,pipe, ect still no luck, still have this wrong messages
You can also try experimenting with changing CRC (length), disabling/enabling dynamic payloads, disabling/enabling auto-ACK and adding ceramic capacitor to the power pins of the nRF24. Max power when modules are near each other might also not be a good idea.
If you see the pictures on my first post, I used PA_MIN on both Rpi and ESP32. Also, I tried 8-bit CRC and no CRC(using dynamic payloads) on both esp32 and rpi but still receive wrong values
There are also clone modules that cause very weird issues in certain configurations, make sure you don't have one of those. There's quite a lot of information about those available online.
But the NRF24 is the only thing installed on my Rpi after fresh Raspbian Buster install: I do the commands sudo apt update sudo apt upgrade sudo reboot sudo raspi-config enable spi
then I just followed this steps.
What's wrong then?
If the issue doesn't exist in
gettingStarted
then it's time to figure out what modification in your code causes the issue.
I take back my claim about the .cpp files are working. They also have the same bug that the message produces wrong value(I have observed that the gettingstarted.cpp also has slight differences on the number it projects versus the values sent by my ESP32)
Before I conducted the Experiment, I freshly installed this NRF24 library. rebuild,reinstalled, but still the problem still persists
OK, so after a lot of tweaks, I finally got the solution to my problem. it is hardware related with the wiring. I retweaked the wire like this:
~made a twisted pair cable(by twisting two dupont wire), and those two wires are for 3.3v and gnd (noise cancellation)
~made a twisted pair cable(by twisting two dupont wire), and those two wires are for MOSI and MISO (noise cancellation)
set the pin configuration like this: NRF24 Raspberry-Pi 4B MOSI - GPIO10(SPI0_MOSI) MISO - GPIO09(SPI0_MISO) CLK - GPIO11(SPI0_CLK) CE - GPIO22(pin no 15) CSN - GPIO08(SPI0_CE0) VCC - 3v3(pin no 1) GND - Ground(pin no 6)
Advise: Raspberry pi is very sensitive to noise I suspect... That is why it is good to twist the cable of vcc-ground, also the mosi-miso cables to cancel the environmental interference inside and outside the source.
Thank you for describing what you did to solve this issue, it's probably very appreciated whoever stumbles upon this in the future.
I think that adding a decoupling ceramic or electrolytic capacitor soldered directly to the nRF24L01+ could help against noise as well. For many people this solves most issues they have with the modules.
Hello, I have been using this library to communicate multiple microcontrollers using NRF24L01+ . But after months, I decided to integrate a communication between Raspberry-Pi and ESP32 wirelessly using two NRF24L01+.
My first test was, Raspberry-Pi act as transmitter while ESP32 act as Receiver, everything worked fine. ESP32 receives the Message "accurately".
My second test, Raspberry-Pi act as receiver while ESP32 act as transmitter. Yes, raspberry-pi successfully receive the message, but sadly the received message are wrong values. Can somebody explain why? Here are the sample codesI used for testing:
ESP32.ino: Compiled with: IDE: Arduino IDE Board: NodeMCU-32S UploadSpeed: 921600 Flash-Speed: 80MHz
RaspberryPi.py:
see screenshots for details about my case:
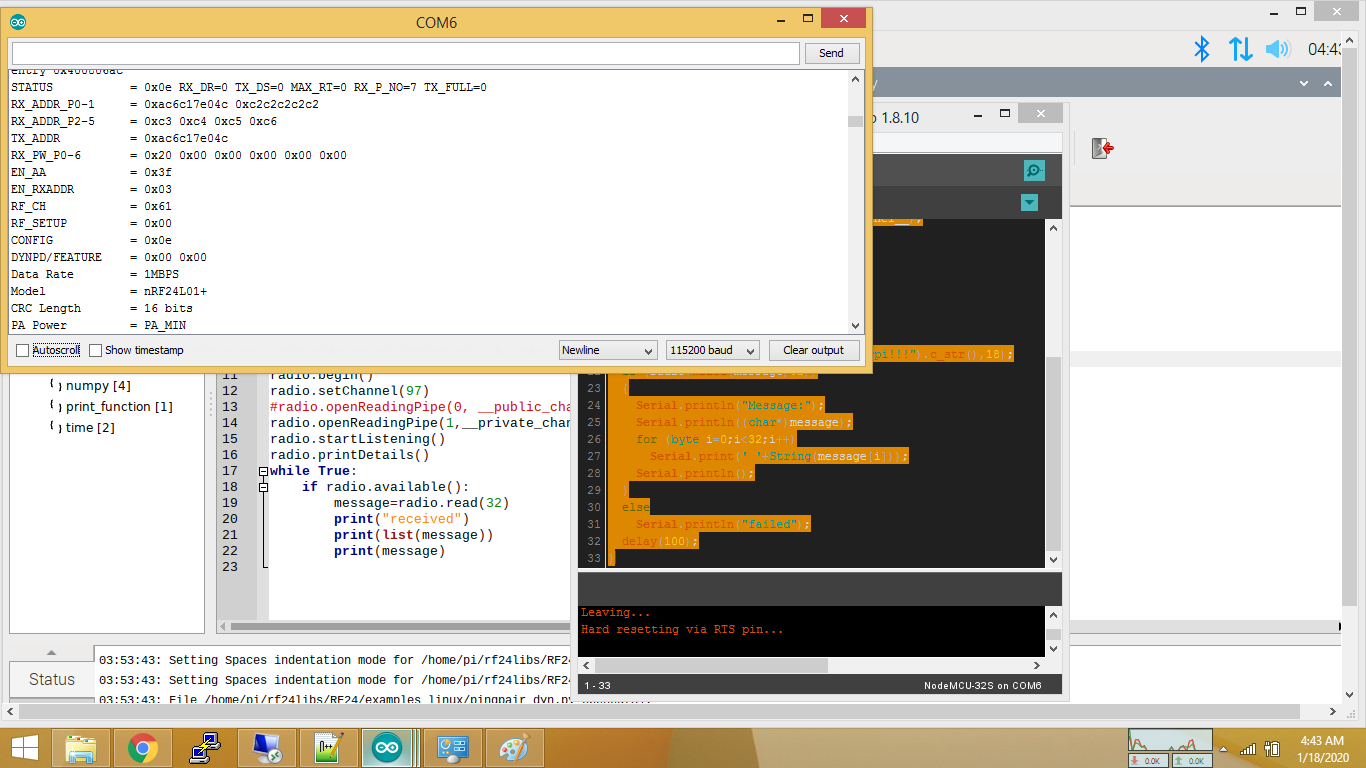
Edit: I Also tried PA_MAX power amplifier level, but still no luck of receiving a good message at the raspberry-pi side:
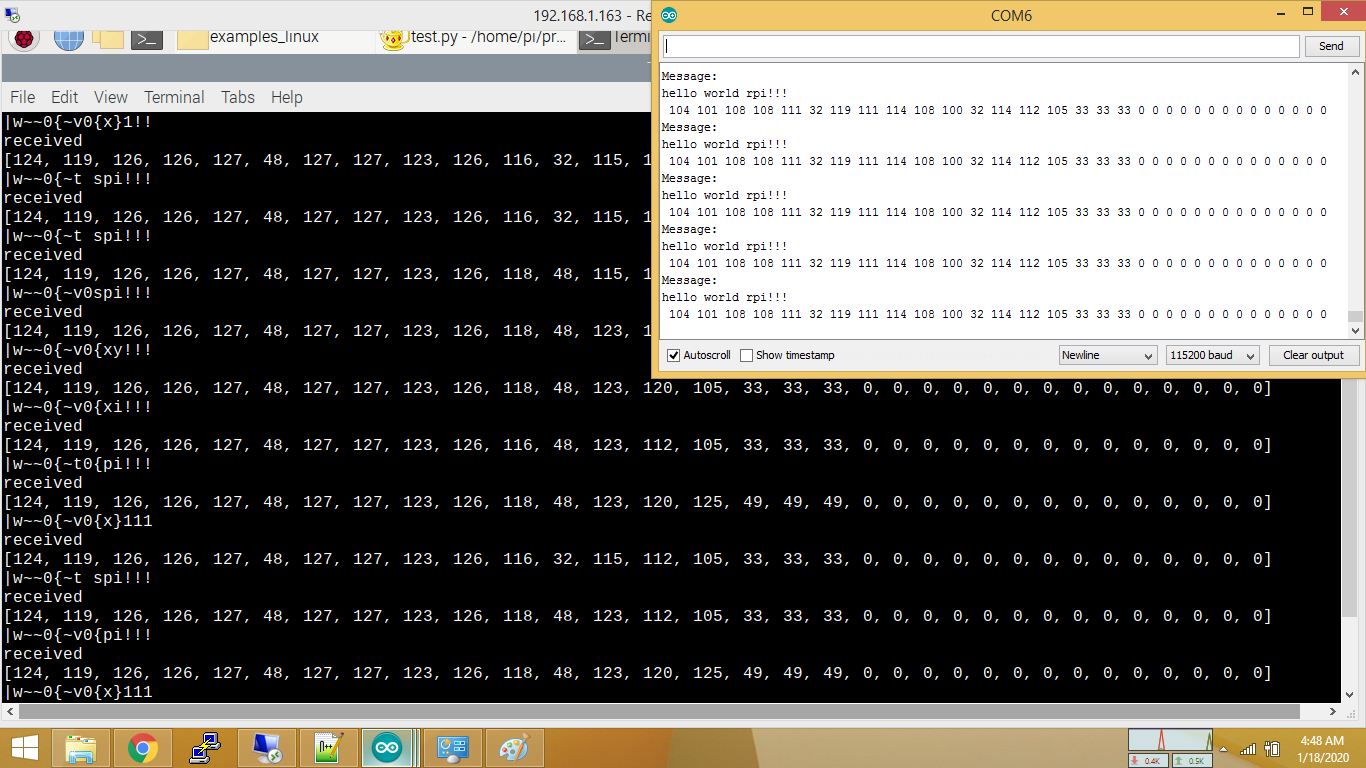