Closed kiredewit closed 2 years ago
This sounds like a power problem. The L1117 datasheet recommends tantalum capacitors (1 on input and 1 on output). Its hard to tell from the picture what you're using in the circuit.
If you're using the 3-wire approach, then you need to drop the SPI speed to 4MHz. This can be done either in platformio.ini
[env...]
board="..."
build_flags=
-DRF24_SPI_SPEED=4000000
or in RF24 c'tor
RF24 radio(CE_PIN, CSN_PIN, 4000000);
This sounds like a power problem. The L1117 datasheet recommends tantalum capacitors (1 on input and 1 on output). Its hard to tell from the picture what you're using in the circuit.
JM15RP LM1117T https://www.tinytronics.nl/shop/image/cache/data/product-1262/LM1117T-1500x1500.jpg.
800mAh output.
I got the same result when I feed the circuit with 3.3V.(without the regulator)
If you're using the 3-wire approach, then you need to drop the SPI speed to 4MHz. This can be done either in platformio.ini
[env...] board="..." build_flags= -DRF24_SPI_SPEED=4000000
or in RF24 c'tor
RF24 radio(CE_PIN, CSN_PIN, 4000000);
I try this before.
The communication part is working with and without setting the SPI speed. When I only disable the code for communications there are still bad results. I don`t think that the SPI speed is the problem.
I was trying to focus on the capacitors and resistors you're using, not the regulator. BJTs (like your regulator) often require capacitors on each end to function properly. If the regulator is adjustable, then there are required resistors in addition to the capacitors. This is all detailed by the L1117 datasheet (see the link I posted). When I say "power", I'm talking about the voltage multiplied by the current (P = V * I). If 800mA is the specification maximum, then that heavily depends on the circuit (made of resistors and capacitors) you're using. Note that it helps to measure the actual voltage and current rather than telling me what you've read in the specifications or tutorials.
With only the sensor connected to the bread board, the values are correct.
This is hard to diagnose since you only describe the problem with very little about the circuit. An actual schematic would help.
Thanks for the advice and tips for so far. You are right about missing components when using a L1117. To limit the possibility for more mistakes I removed the regulator and use a 3.3v power source(from the FTDI).
When I use 3.3v from the FTDI and remove the regulator, I still get the same problem with analog read on PB3.
The actual scheme is now almost the same as in the manual. I removed the led and 5v, because the power source is 3.3V.
I connected the sensor AOUT to PB3.
^^
+-\/-+ nRF24L01 CE, pin3 ------| //
PB5 1|o |8 Vcc --- nRF24L01 VCC, pin2 ------x----------x--|<|-- 5V
NC PB3 2| |7 PB2 --- nRF24L01 SCK, pin5 --|<|---x-[22k]--| LED
NC PB4 3| |6 PB1 --- nRF24L01 MOSI, pin6 1n4148 |
nRF24L01 GND, pin1 -x- GND 4| |5 PB0 --- nRF24L01 MISO, pin7 |
| +----+ |
|-----------------------------------------------||----x-- nRF24L01 CSN, pin4
10nF
NerdRalph vision:
The only difference I see is a capacitor value from C1. 0.1uF is 100nF where the RF24 doc recommends 10nF. ~This value is non-negotiable.~ Unless the value in ~your~ nerdRalph's schematic is a typo or something.
It is possible that you caused damage to the soil sensor (via current inrush spikes) when not properly using the regulator. The radio may have been protected by the diode but that's not a guarantee because it is an LED (not a power diode). I've always been against nerdRalph having used an LED in his blog post. Power stability is very important (especially with the ICs).
Original RC combination was 1K/100nF. 22K/10nF combination worked better.
I use the 22k/10nF combination. (ceramic capacitor 103))
It is possible that you caused damage to the soil sensor (via current inrush spikes) when not properly using the regulator.
Uhmm I didn`t think about that. I will try directly with a new sensor.
The radio may have been protected by the diode but that's not a guarantee because it is an LED (not a power diode). I've always been against nerdRalph having used an LED in his blog post. Power stability is very important (especially with the ICs)
Good to know. Thanks.
@kiredewit use >
to denote a blockquote 😉
> a blockquote ends in a blank line
this a reply to the quoted text
@kiredewit use
>
to denote a blockquote wink> a blockquote ends in a blank line this a reply to the quoted text
:) thanks. That`s more readable.
With a new sensor and 3.3v power source, i got the same result :(.
well, I guess that's good news for the sensor.
I'm not sure if this sounds obvious to you. but a golden rule of DC electricity is make sure the ground is always shared by all devices in a given circuit.
I'm really stretching here because I'm running out of ideas for possible problems. It can't be a software problem because you've commented out the RF24 code. That's why I'm focused on hunting electrical problems.
Have you tried using an Arduino Nano/Uno instead of an ATTiny85? That might help narrow things down.
I'm not sure if this sounds obvious to you. but a golden rule of DC electricity is make sure the ground is always shared by all devices in a given circuit.
They all use the same ground :).
Have you tried using an Arduino Nano/Uno instead of an ATTiny85? That might help narrow things down.
I have tried this on a wemos D1 and attiny85 with only the sensor connected. With the attiny85, I used a different pin. With both devices the sensor give the correct values.
I just tested the last circuit again(attiny85 with only the sensor connected). It`s looks like the sensor is only giving good values when it is connected to PB2. PB3 give different values then the circuit with NR24 connected, still the values are not correct.
Uhmm I think I need first to find out why the sensor is only working with PB2.
Seems like a ATTiny problem to me. Glad you were able to narrow it down. I don't remember all the specific reserved functionality for the ATTiny85 pins, but I think a couple are reserved for Serial emulation.
Worth noting that the platformIO core you're using isn't something we test for. I have tested the SpenceKonde core for ATTiny85 because that's what we officially recommend for the Arduino IDE.
closing this since there doesn't seem to be any need for action on our end.
Feel free to report your findings about why PB2 works and why PB3 doesn't.
I have a problem with reading analog input from the ATTINY85(PB3) when using the setup described in the ATTiny manual/instructables/source.
when I connect a capacitive soil moisture level 1.2 to PB3, I expect a value around the 600.
With only the sensor connected to the bread board, the values are correct.
When I use the setup as described in the manuals, the analog read value is building up to 1023.(the code is without communication with the nrf2401, just a test code for reading the analog input on PB3).
There is no problem with sending and receiving data. In this case the sensor is only transmitting data.(receiver is a wemos D1 mini)
Setup: FT232RL (for power and serial communication, power to circuit 3.3V, original power source will be a 9V battery) ATTiny85 LM1117T 3.3V (removed to get better focus on the problem) nrf24l01
Mine: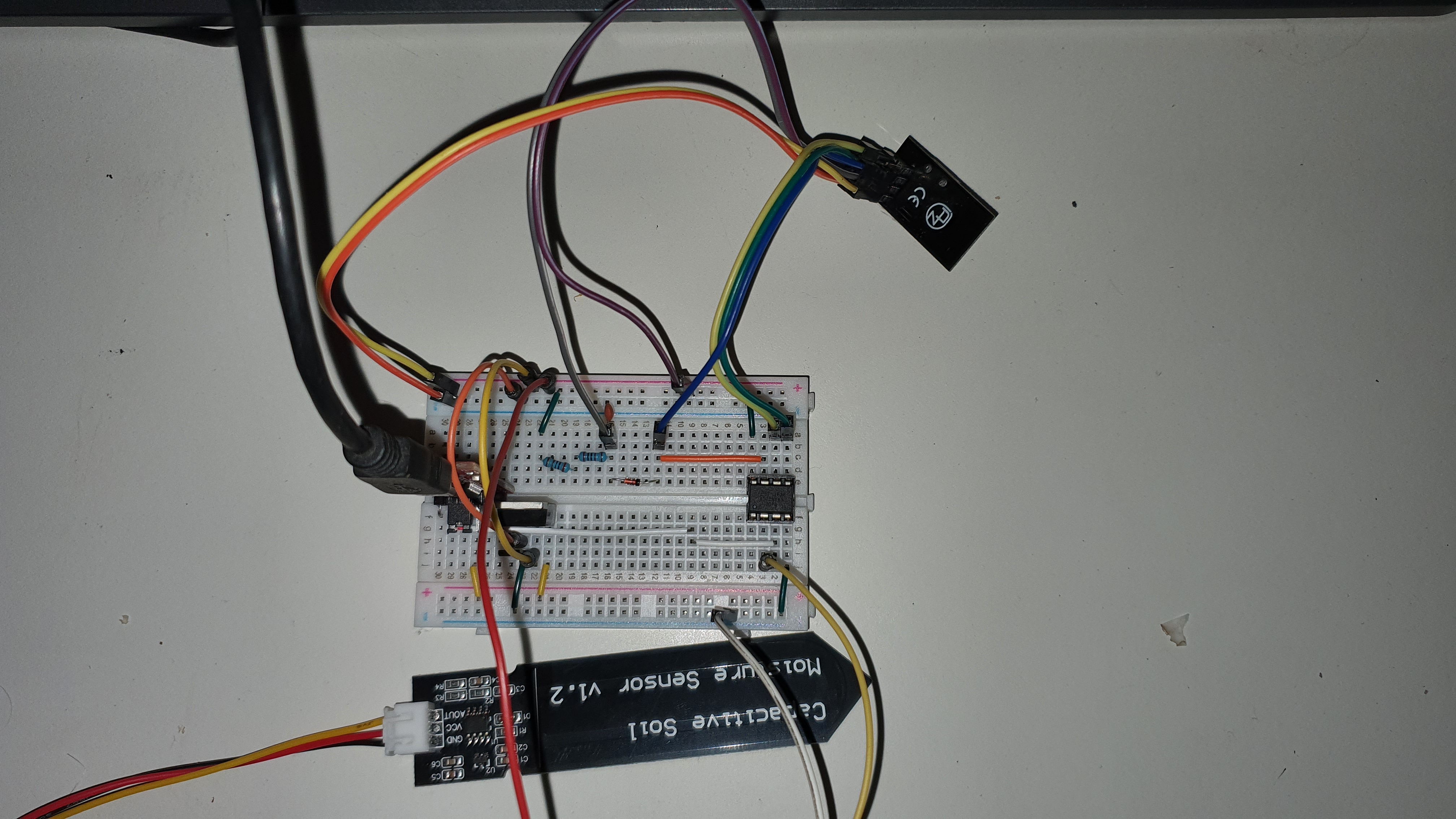
used example
Transmitter code
platform.ini:
main.cpp: (only sensorread is active)
Some Serial output(i was expecting a value around the 600/700): 1022 --[0---100---2] 1015 --[0---100---2] 1013 --[0---100---2] 1011 --[0---100---2] 1008 --[0---100---2] 1010 --[0---100---2] 1010 --[0---100---2] 1016 --[0---100---2] 1021 --[0---100---2] 1023 --[0---100---2]
If I remove the ceramic capacitor and the VCC for nrf2401 the analog value for PB3 is changing to a lower number, still not in the correct range. Can someone explain why the analog read on PB3 give me not the expecting value with the full setup?