Open name27 opened 1 year ago
// ignore_for_file: prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:flutter/src/widgets/container.dart';
import 'package:flutter/src/widgets/framework.dart';
class DrinkTile extends StatelessWidget {
const DrinkTile(this.nameK, this.nameE, this.price, this.imgUrl);
final String nameK;
final String nameE;
final String price;
final String imgUrl;
@override
Widget build(BuildContext context) {
return ListTile(
title: Text(
nameK,
style: TextStyle(
fontSize: 16,
fontWeight: FontWeight.bold,
),
),
subtitle: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
nameE,
style: TextStyle(
fontSize: 14,
fontWeight: FontWeight.w200,
),
),
Text(
price,
style: TextStyle(
fontSize: 16,
color: Colors.black,
fontWeight: FontWeight.bold,
),
),
],
),
isThreeLine: true,
leading: CircleAvatar(
backgroundImage: AssetImage(imgUrl),
radius: 48,
),
);
}
}
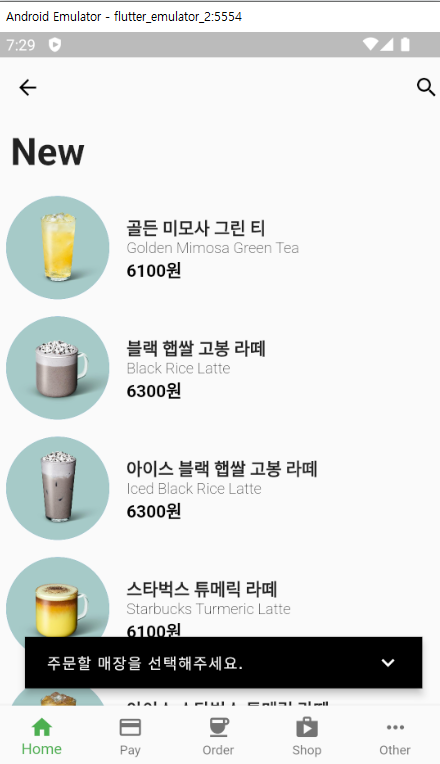
// ignore_for_file: prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:flutter/src/widgets/container.dart';
import 'package:flutter/src/widgets/framework.dart';
class DrinkTile extends StatelessWidget {
const DrinkTile(this.nameK, this.nameE, this.price, this.imgUrl);
final String nameK;
final String nameE;
final String price;
final String imgUrl;
@override
Widget build(BuildContext context) {
return Row(
children: [
Padding(
padding: const EdgeInsets.all(8.0),
child: CircleAvatar(
backgroundImage: AssetImage(imgUrl),
radius: 48,
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
nameK,
style: TextStyle(
fontSize: 16,
fontWeight: FontWeight.bold,
),
),
Text(
nameE,
style: TextStyle(
fontSize: 14,
fontWeight: FontWeight.w200,
),
),
Text(
price,
style: TextStyle(
fontSize: 16,
color: Colors.black,
fontWeight: FontWeight.bold,
),
),
],
),
),
],
);
}
}
// ignore_for_file: prefer_const_constructors
// ignore_for_file: prefer_const_literals_to_create_immutables
import 'package:contact_app/ContactFile.dart';
import 'package:contact_app/DrinkTile.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false, //debug 배너 제거
home: Scaffold(
appBar: AppBar(
backgroundColor: Colors.transparent,
foregroundColor: Colors.black,
elevation: 0,
leading: Icon(Icons.arrow_back),
actions: [
Icon(Icons.search),
],
),
body: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Padding(
padding: const EdgeInsets.all(12.0),
child: Text(
'New',
style: TextStyle(
fontSize: 35,
fontWeight: FontWeight.bold,
),
),
),
Expanded(child: const SnackBarPage()),
],
),
bottomNavigationBar: BottomNavigationBar(
type: BottomNavigationBarType.fixed,
selectedItemColor: Colors.green,
items: [
BottomNavigationBarItem(icon: Icon(Icons.home), label: 'Home'),
BottomNavigationBarItem(icon: Icon(Icons.payment), label: 'Pay'),
BottomNavigationBarItem(icon: Icon(Icons.coffee), label: 'Order'),
BottomNavigationBarItem(icon: Icon(Icons.shop), label: 'Shop'),
BottomNavigationBarItem(
icon: Icon(Icons.more_horiz), label: 'Other'),
],
),
),
);
}
}
class SnackBarPage extends StatelessWidget {
const SnackBarPage({super.key});
@override
Widget build(BuildContext context) {
return Center(
child: ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.white
),
onPressed: () {
final snackBar = SnackBar(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('주문할 매장을 선택해주세요'),
Icon(Icons.keyboard_arrow_down, color: Colors.white,),
],
),
);
ScaffoldMessenger.of(context).showSnackBar(snackBar);
},
child: ListView(
children: [
DrinkTile(
'골든 미모사 그린 티',
'Golden Mimosa Green Tea',
'6100원',
'assets/images/item_drink1.jpeg'
),
DrinkTile(
'블랙 햅쌀 고봉 라떼',
'Black Rice Latte',
'6300원',
'assets/images/item_drink2.jpeg'
),
DrinkTile(
'아이스 블랙 햅쌀 고봉 라떼',
'Iced Black Rice Latte',
'6300원',
'assets/images/item_drink3.jpeg'
),
DrinkTile(
'스타벅스 튜메릭 라떼',
'Starbucks Turmeric Latte',
'6100원',
'assets/images/item_drink4.jpeg'
),
DrinkTile(
'아이스 스타벅스 튜메릭 라떼',
'Iced Starbucks Turmeric Latte',
'6100원',
'assets/images/item_drink5.jpeg'
),
],
),
),
);
}
}