Open rivermoon21 opened 5 years ago
It looks like the problem lies within your composition provider.
It applies to every domainObject which resides in the namespace public.namespace
and is of type folder
.
But all your subsytem domainObjects also reside in the namespace public.namespace
and are of the type folder
. So everytime a new object is added by the objectprovider openmct checks if the compositionprovider applies to it and load all the subsytem domainobjects for it too. And since all of your domainObjects apply to it it will end in an endless loop.
You can fix it tho by changing your appliesTo
function, for example try:
appliesTo: function (domainObject) {
return domainObject.identifier.namespace === 'public.namespace' &&
domainObject.type === 'folder' &&
domainObject.identifier.key === 'my-root-major-key';
}
Also to answer your second question why they are not shown as telemetry types:
folder
to every subsytem domainObject. Thats why its displayed as a folder.values
array.Try to use
return {
identifier: identifier,
name: measurement.name,
type: 'example.telemetry',
telemetry: {
values: measurement.measurements,
},
location: 'public.namespace:my-root-major-key'
};
in your object provider.
Also your root key 'my-root-major-key' and the root key in the json file ('sc') don't match. That is not a problem, but it is definitely confusing.
Thanks for the help. That solved one of the issues I was facing. Now the hierarchy shows the spacecraft and the subsystems. However, it doesn't show the telemetry data point of each subsystem in the hierarchy tree on the left. It only shows it on the main screen (right). [as shown in the image]
Do you have any suggestions for this?
It seems to be pretty close, I just don't see what changes I need to do.
This is what I have as far as types and providers:
` openmct.objects.addRoot({ namespace: 'public.namespace', key: 'my-root-major-key' });
openmct.types.addType('example.my-type', {
name: "My Personal Type",
description: "This is a personal type that I added!",
creatable: true,
cssClass: "icon-plot-resource"
});
openmct.types.addType('example.telemetry', {
name: "My Personal Type",
description: "This is a personal type that I added!",
cssClass: "icon-plot-resource"
});
openmct.types.addType('example.subsytem', {
name: "My subsytem Type",
description: "This is a subsytem type that I added!",
cssClass: "icon-folder"
});
openmct.objects.addProvider('public.namespace', {
get: function (identifier) {
return objectPromise.then(function(data) {
if(identifier.key === 'my-root-major-key')
{
return{
identifier: identifier,
name: data.name,
type: data.type,
location: 'ROOT'
}
}
//fill in data points for each subsytem
else {
var measurement = data.subsystems.filter(function (m) {
return m.key === identifier.key;
})[0];
return {
identifier: identifier,
name: measurement.name,
type: 'example.subsytem',
telemetry: {
values: measurement.measurements,
},
location: 'public.namespace:my-root-major-key'
};
}
});
}
});
openmct.composition.addProvider({
appliesTo: function (domainObject) {
return domainObject.identifier.namespace === 'public.namespace' &&
domainObject.type === 'folder' &&
domainObject.identifier.key === 'my-root-major-key';
},
load: function (domainObject) {
return objectPromise.then(function (data) {
return data.subsystems.map(function (m) {
return {
namespace: 'public.namespace',
key: m.key
};
});
});
}
});`
@mauricerivers - You need to provide values for all measurement types. Please share your repo so I can fully understand the issue you are having
Here is the link to my repo: https://github.com/mauricerivers/openmct-testing-objects/blob/master/index.html
All the code is in the index.html because I am just trying to get this to work. Then I will clean out the repo. Hope you can help. Thanks.
Was there any solution to this? I too am looking to produce a composition provider that supports Root-->Subsystem-->Telemetry object
I published my solution to folder hierarchy here: Discussion: Folders hierarchy #7494
Hello, I am trying to build and display an object that has a root folder, subsystems, and the subsystem's data point. However, when I try in the code, the subsystems appear repeatedly every time [as shown in pic].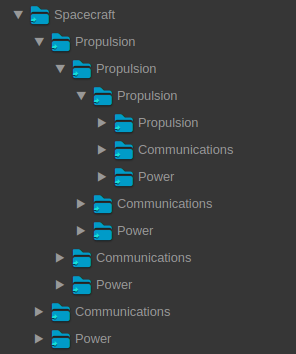
Can you guys help me figure out what I need to add to my Object Provider or Composition provider? I thought it would display it by adding the 'telemetry' argument
here is my json file (similar to tutorial found online):
And Object Provider:
And Composition Provider:
Issue: Help in displaying my objectn correctly with its subsystems and their respective telemetry. Severirty: Trivial.