Closed binarytracer closed 5 years ago
You should close your Nest application in either after
or afterEach
hook (app.close()
)
@kamilmysliwiec its working and more simple way than i thought.
@binarytracer How did you do it? can you share your piece of code solving this problem?
@Kronhyx
You should close your Nest application in either
after
orafterEach
hook (app.close()
)
Closing the application in afterAll
did not work for me, and VSCode could not find after
. I had to use afterEach
:
afterEach(async () => {
// If you don't call this, then the tests will hang
await app.close();
});
I'm submitting a...
Current behavior
file: src/app/app.controller.spec.ts
result:
Expected behavior
Minimal reproduction of the problem with instructions
running npm script again,
with adjustments
result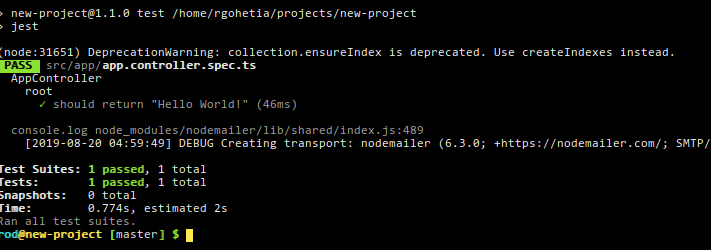
What is the motivation / use case for changing the behavior?
Environment