Open valdeua opened 1 year ago
Take a look at this project: https://github.com/gid-oss/dataui-nestjs-crud
@valdeua I think this fix is wha you want - https://github.com/rewiko/crud/pull/20
I'm having the same issue. Is there any plan to update this library? Or should be switching to the forked projects?
I have same issue when use limit
in query:
@Crud({
model: {
type: TransactionEntity,
},
routes: {
only: ['getManyBase', 'getOneBase'],
},
query: {
alwaysPaginate: true,
limit: 25,
join: {
deposit: {
eager: true,
},
},
})
// QueryFailedError: ER_DUP_FIELDNAME: Duplicate column name 'TransactionEntity_id'
same issue here , anyone has a solution ?
Bug Report
Current behavior
When I try to join ('eager' type) related entity I received 'Duplicate column name'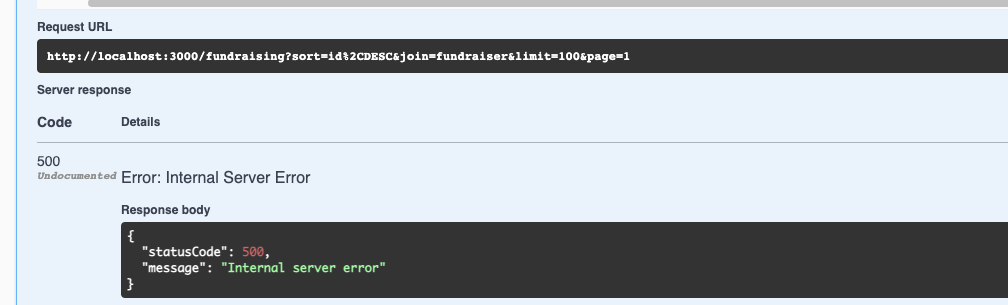
[Nest] 39063 - 11/30/2022, 12:11:42 PM ERROR [ExceptionsHandler] Duplicate column name 'Fundraising_id'
Here is an exception:
I think an important precondition here is: I am trying to get data with ?limit When I tried to get data without limit I didn't have error: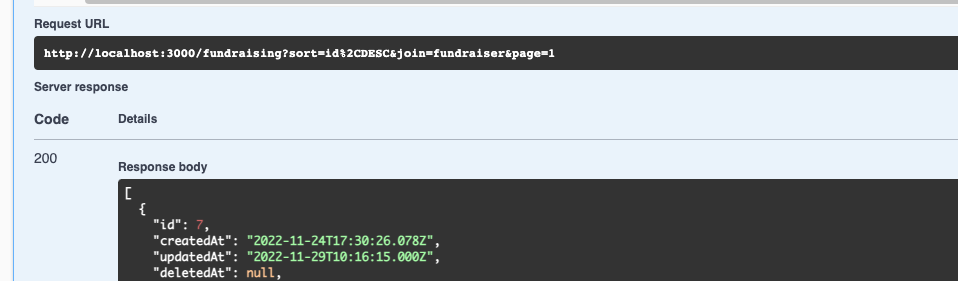
Expected behavior
Eagerly joined column without duplication error
What I have tried
I tried aliases but it doesn't help https://github.com/nestjsx/crud/issues/281#issuecomment-566964787 relation field renaming also wasn't helpful
My CRUD example:
Models:
Generated Query example
For Tooling issues:
Packages: