Closed oauramos closed 2 years ago
Everything is working, except missing hash! :cry:
Hi @middlebaws
You just forgot to add the strictDynamic
and strictInlineStyles
middlewares to securityMiddleware
:). They inject the strict CSP features on top of your base csp
configuration. If you add them, it should work:
import { chain, chainMatch,csp, isPageRequest,nextSafe, strictDynamic, strictInlineStyles } from '@next-safe/middleware';
import { NextRequest, NextResponse } from 'next/server';
const PUBLIC_FILE = /\.([a-zA-Z0-9]+$)/;
function routesMiddleware(request: NextRequest) {
...
}
const securityMiddleware = [
nextSafe({ disableCsp: true }),
csp({
// your CSP base configuration with IntelliSense
// single quotes for values like 'self' are automatic
directives: {
'default-src': ['self'],
'script-src': ['self'],
'style-src': ['self', 'https://fonts.googleapis.com', 'unsafe-inline'],
'font-src': ['self', 'https://fonts.gstatic.com', 'data:', ],
'img-src': ['self', 'data:', 'https://*.google-analytics.com', 'https://*.analytics.google.com', 'https://*.googletagmanager.com', 'https://*.g.doubleclick.net', 'https://*.google.com'],
'media-src': ['self', 'data:', 'http://localhost:3000' ],
'connect-src': ['self', 'https://*.google-analytics.com' , 'https://*.analytics.google.com', 'https://*.googletagmanager.com', 'https://*.g.doubleclick.net', 'https://*.google.com']
},
}),
strictDynamic(),
strictInlineStyles(),
];
export default chain(
routesMiddleware,
chainMatch(isPageRequest)(...securityMiddleware)
)
I would also recommend initializing the style server outside of the MyDocument.getInitialProps
closure. The e2e app uses a setup with Mantine (uses emotion under the hood) that works and in Mantine docs it is done outside, like so:
const cache = createEmotionCache();
const { extractCriticalToChunks } = createEmotionServer(cache);
MyDocument.getInitialProps = async (ctx) => {
const originalRenderPage = ctx.renderPage;
...
}
Please let me know if that solved your problem. I am also going to release 0.9.0
soon and drop support for Beta middleware in favor of stable middleware (no more pages/_middleware.ts
, just root-level middleware.ts
), so I also recommend the upgrade to Next 12.2 and 0.9.0
, especially if you host your app on Vercel, I did some stuff to massively decrease CPU usage (see #45).
Okay, reading here some questions related to Mantine. I build my own ServerStyle.tsx and works pretty great at Chrome. (Locally and deployed)
Buuuuuuuuuut, strict-dynamic are not supported by firefox. Have some way to work with that?
I am currently working on #40 and I am experimenting with loading Next by an inline script proxy (transitive trust). I tried that before, but it messed up Next script loading, but I have a new idea that could work. Then it would work with Firefox.
With the current approach (injecting integrity attributes to scripts with src) unfortunately not, because Firefox messes up SRI validation for scripts with src attribute in conjunction with strict-dynamic
: https://bugzilla.mozilla.org/show_bug.cgi?id=1409200
For me its fine, if you want can close this case.
Hi @nibtime , how are you doing?
Im trying to apply next-safe-middleware in Nextjs + Emotion + MUI application, why? Because using emotionCache and use default CSP provided by Vercel not work.
Here my
_document.tsx
Also, follow my _middleware.tsx using chain() (of course)
My huge question is, I already add config into dynamic
staticProps
andstaticPaths
But still suffering from missing hash/nonce alerts in App :/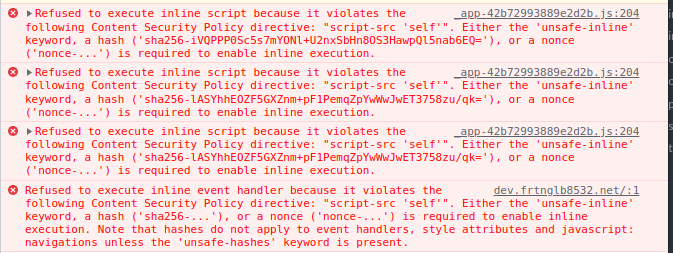
NextJS:
2.1.5
"@emotion/cache":"^11.7.1",
"@emotion/react":"^11.8.1",
"@emotion/server":"^11.4.0",
"@emotion/styled":"^11.8.1",