Open GreenRover opened 2 years ago
Assuming this example:
Node a1 = node("A1"); Node b1 = node("B1"); Node c1 = node("C1"); Node c2 = node("C2"); List<LinkSource> linkSourcesB = new ArrayList<>(); linkSourcesB.add(a1); linkSourcesB.add(b1); linkSourcesB.add(c1); linkSourcesB.add(c2); linkSourcesB.add( graph().graphAttr() .with(Rank.inSubgraph(Rank.RankType.SAME), Rank.dir(TOP_TO_BOTTOM)) .with(a1, b1, c1) ); linkSourcesB.add( graph().graphAttr() .with(Rank.inSubgraph(Rank.RankType.SAME), Rank.dir(LEFT_TO_RIGHT)) .with(c1, c2) ); linkSourcesB.add(a1.link(b1)); linkSourcesB.add(b1.link(c1)); linkSourcesB.add(c1.link(c2)); Graph g = graph("broker2concentrator").directed() .graphAttr().with(Rank.dir(LEFT_TO_RIGHT)) .nodeAttr().with(Font.name("arial")) .with(linkSourcesB); ByteArrayOutputStream outputStream = new ByteArrayOutputStream(); Graphviz .fromGraph(g) .render(Format.PNG) .toOutputStream(outputStream); return outputStream.toByteArray();
The current result:
The wanted result:
Is the wanted example possible and how?
Here a playground with full complexity: https://dreampuf.github.io/GraphvizOnline/#digraph%20G%20%7B%0A%20%20%20%20%0A%20%20rankdir%20%3D%20TD%3B%0A%0A%20%20A1%20-%3E%20B1%20-%3E%20C1%20-%3E%20C2%0A%20%20%0A%20%20subgraph%20%7B%20%0A%20%20%20%20%20rank%20%3D%20sink%20%3B%20rankdir%20%3D%20TB%20%3B%20A1%3B%20B1%3B%20C1%3B%20%0A%20%20%7D%20%0A%20%20%0A%20%20subgraph%20%7B%20%0A%20%20%20%20%20rank%20%3D%20same%20%3B%20rankdir%20%3D%20LR%20%3B%20C1%3B%20C2%3B%20%0A%20%20%7D%20%0A%0A%20%20%0A%20%20A1_x1%20-%3E%20A1%0A%20%20A1_x2%20-%3E%20A1%0A%20%20A1_x3%20-%3E%20A1%0A%20%20A1_x4%20-%3E%20A1%0A%20%20A1_x99%20-%3E%20A1%0A%0A%20%20B1_x1%20-%3E%20B1%0A%20%20B1_x2%20-%3E%20B1%0A%20%20B1_x3%20-%3E%20B1%0A%20%20B1_x4%20-%3E%20B1%0A%20%20B1_x99%20-%3E%20B1%0A%0A%20%20C1_x1%20-%3E%20C1%0A%20%20C1_x2%20-%3E%20C1%0A%20%20C1_x3%20-%3E%20C1%0A%20%20C1_x4%20-%3E%20C1%0A%20%20C1_x99%20-%3E%20C1%0A%20%20%0A%20%20C2_x1%20-%3E%20C2%0A%20%20C2_x2%20-%3E%20C2%0A%20%20C2_x3%20-%3E%20C2%0A%20%20C2_x4%20-%3E%20C2%0A%20%20C2_x99%20-%3E%20C2%0A%7D
here a possible solution. How is it possible to do this with the java api?
https://stackoverflow.com/questions/69080171/graphiz-direction-nodes-as-an-l/69081229?noredirect=1#comment122095835_69081229
Assuming this example:
The current result: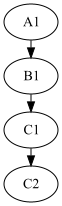
The wanted result: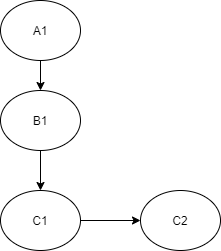
Is the wanted example possible and how?
Here a playground with full complexity: https://dreampuf.github.io/GraphvizOnline/#digraph%20G%20%7B%0A%20%20%20%20%0A%20%20rankdir%20%3D%20TD%3B%0A%0A%20%20A1%20-%3E%20B1%20-%3E%20C1%20-%3E%20C2%0A%20%20%0A%20%20subgraph%20%7B%20%0A%20%20%20%20%20rank%20%3D%20sink%20%3B%20rankdir%20%3D%20TB%20%3B%20A1%3B%20B1%3B%20C1%3B%20%0A%20%20%7D%20%0A%20%20%0A%20%20subgraph%20%7B%20%0A%20%20%20%20%20rank%20%3D%20same%20%3B%20rankdir%20%3D%20LR%20%3B%20C1%3B%20C2%3B%20%0A%20%20%7D%20%0A%0A%20%20%0A%20%20A1_x1%20-%3E%20A1%0A%20%20A1_x2%20-%3E%20A1%0A%20%20A1_x3%20-%3E%20A1%0A%20%20A1_x4%20-%3E%20A1%0A%20%20A1_x99%20-%3E%20A1%0A%0A%20%20B1_x1%20-%3E%20B1%0A%20%20B1_x2%20-%3E%20B1%0A%20%20B1_x3%20-%3E%20B1%0A%20%20B1_x4%20-%3E%20B1%0A%20%20B1_x99%20-%3E%20B1%0A%0A%20%20C1_x1%20-%3E%20C1%0A%20%20C1_x2%20-%3E%20C1%0A%20%20C1_x3%20-%3E%20C1%0A%20%20C1_x4%20-%3E%20C1%0A%20%20C1_x99%20-%3E%20C1%0A%20%20%0A%20%20C2_x1%20-%3E%20C2%0A%20%20C2_x2%20-%3E%20C2%0A%20%20C2_x3%20-%3E%20C2%0A%20%20C2_x4%20-%3E%20C2%0A%20%20C2_x99%20-%3E%20C2%0A%7D