Closed reallymello closed 1 year ago
it seems like the old callback style doesn't work reliable when using supertest api testing so we're only going to support the async
version for when writing supertest api tests using the @nightwatch/apitesting
plugin.
@reallymello Given the example you provided, this works reliable using nightwatch 2.6.4 and @nightwatch/apitesting v0.2.1:
test('can GET count of sold inventory', async ({ supertest }) => {
await supertest
.request('https://petstore.swagger.io/v2')
.get('/store/inventory/')
.expect(200)
.expect('Content-Type', /json/)
.then((response) => {
expect(response.body.sold).to.be.greaterThan(0);
// console.log(JSON.stringify(response.body, null, 2));
});
});
test('can POST a pet to the store', async ({ supertest }) => {
await supertest
.request('https://petstore.swagger.io/v2')
.post('/pet')
.send({
id: 31337,
category: {
id: 1313,
name: 'owls',
},
name: 'Bitey',
photoUrls: ['https://nightwatchjs.org/images/images1/mini_logo.svg'],
tags: [
{
id: 0,
name: 'replicant',
},
],
status: 'available',
})
.expect(200)
.expect('Content-Type', /json/)
.then((response) => {
expect(response.body.id).to.equal(31337);
expect(response.body.status).to.equal('availablee'); // purposeful typo to test Nightwatch bug
});
});
test('can POST order to the pet store', async ({ supertest }) => {
await supertest
.request('https://petstore.swagger.io/v2')
.post('/store/order')
.send({
id: 0,
petId: 31337,
quantity: 1,
shipDate: '2022-12-30T14:55:04.147Z',
status: 'placed',
complete: true,
})
.expect(200)
.expect('Content-Type', /json/)
.then((response) => {
expect(response.body.id).to.be.greaterThan(0);
expect(response.body.quantity).to.equal(1);
expect(response.body.status).to.equal('placed');
});
});
Just checked in 2.6.4 using async/await instead and it is logging correctly and catching the seeded expect mismatch in the then. Looks good now.
awesome, thanks for your help fixing this!
Description of the bug/issue
When I execute a test suite with several test cases in it leveraging @nightwatch/apitesting I'm seeing log entries from the tests in that suite getting mixed up under the headings for the other tests in the suite. Also, despite an assertion failure being logged, the overall execution reports passing. Perhaps this is related to the use of .then?
Steps to reproduce
nightwatch
in the ./apiTesting/ folder from here https://github.com/reallymello/nightwatchTutorials/tree/master/apiTestingSample test
Command to run
Verbose Output
Nightwatch Configuration
Nightwatch.js Version
2.6.3
Node Version
16.15.0
Browser
Chrome
Operating System
Windows 10
Additional Information
https://github.com/reallymello/nightwatchTutorials/tree/master/apiTesting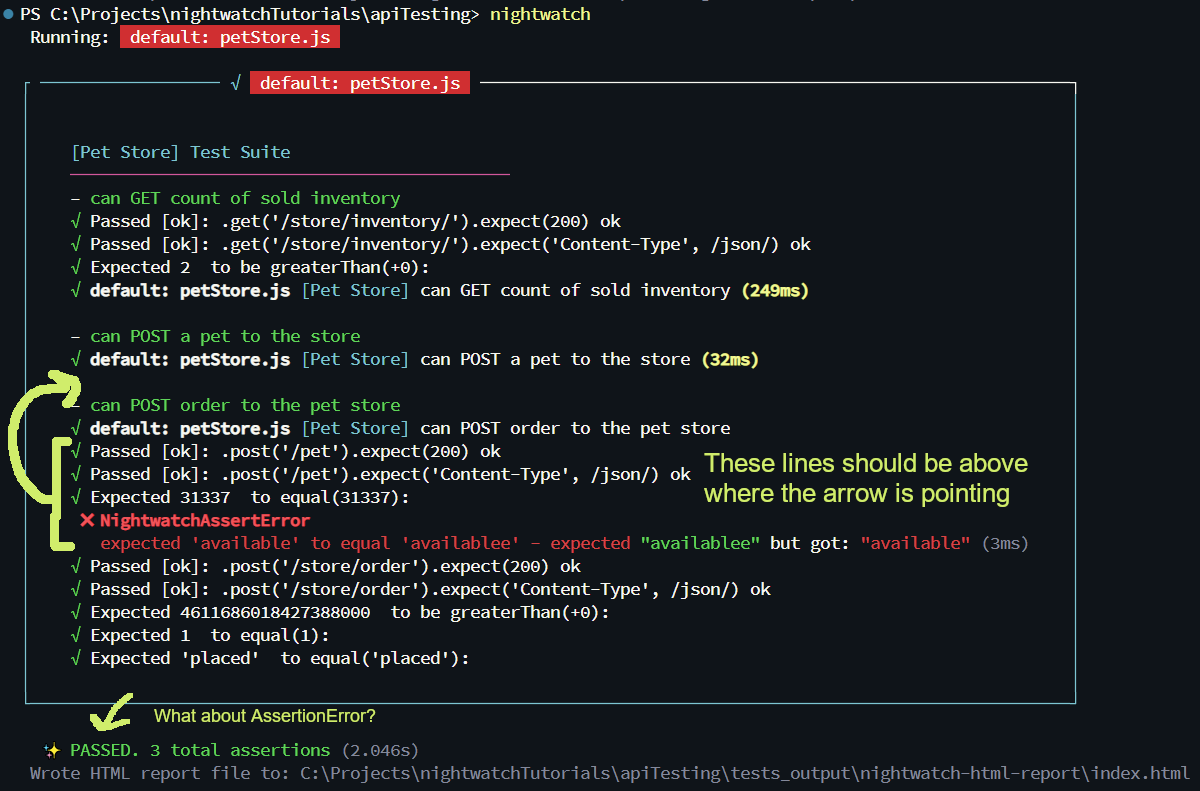