Closed devzakir closed 2 years ago
I'm also struggling with these warnings. I think the LogRocket article describes setting this up for Apollo Client 2, while Apollo Client 3 suggests a slightly different setup:
Apollo 3: https://www.apollographql.com/docs/react/data/fragments/#defining-possibletypes-manually
My Apollo config looks more or less like this:
import { InMemoryCache } from '@apollo/client'
import { possibleTypes } from '../fragmentTypes.json' // exported from codegen
export default function () {
return {
httpEndpoint: process.env.GRAPHQL_ENDPOINT,
cache: new InMemoryCache({ possibleTypes })
}
}
And I'm fetching data in my Vue component like so. I'm actually using Nuxt in static mode so I mostly want to use queries to pre-render my views. I think prefetch
is supposed to do this?
export default {
apollo: {
myQuery: {
prefetch: true,
query: gql`...`
}
}
}
However, I'm having other issues. It seems like the component initially mounts without any data. Also, generating a static site seems to work fine, but when I visit the site it doesn't work at all. However, if I disable the cache, everything works fine except for these warnings during development. I'm going to proceed without a cache and hope for the best. Any downside in doing this?
I also admit I'm quite new to Apollo – perhaps supplying a cache implicitly tells Apollo I want to fetch data client-side? That could explain some of my issues.
@maximilliangeorge I am using this config for Apollo Client 3. config works fine.
import { InMemoryCache } from 'apollo-cache-inmemory'
import dataTypes from './fragmentTypes.json'
export default function(ctx) {
return {
httpEndpoint: `http://localhost:1337/graphql`,
cache: new InMemoryCache({ possibleTypes: dataTypes.possibleTypes })
}
}
Hey @devzakir
I get the same behaviour as you when I use InMemoryCache
as imported from apollo-cache-inmemory
import { InMemoryCache } from 'apollo-cache-inmemory'
However, when I use it from @apollo/client
I get the errors as I described above.
import { InMemoryCache } from '@apollo/client'
Not sure which one I'm supposed to use... I see a lot of conflicting documentation on this. Here's from the Apollo docs:
As of Apollo Client 3.0, the InMemoryCache class is provided by the @apollo/client package. No additional libraries are required.
https://www.apollographql.com/docs/react/caching/cache-configuration/
I got it to work like this. Seems like Nuxt Apollo is still on the old version.
import { InMemoryCache, IntrospectionFragmentMatcher } from 'apollo-cache-inmemory'
import schema from '../graphql/schema.json'
const fragmentMatcher = new IntrospectionFragmentMatcher({
introspectionQueryResultData: schema
})
export default function () {
return {
cache: new InMemoryCache({ fragmentMatcher })
}
}
My codegen.yml
config had issues with apollo version. I set apolloClientVersion
to 2
. Now it works fine!
Here is the updated config
schema: "http://localhost:1337/graphql"
generates:
./graphql/fragmentTypes.json:
plugins:
- "fragment-matcher"
config:
apolloClientVersion: 2
Helllo! I need help with a graphql error. #help #needed
I am using @strapijs with @apollographql and @nuxt_js (frontend).
Getting "(heuristic) fragment matcher" error after caching possibleTypes following a article on LogRocket (https://blog.logrocket.com/using-code-gen-to-avoid-heuristic-graphql-queries/)
Here is the Github Repo -> https://github.com/devzakir/strapi-graphql-nuxt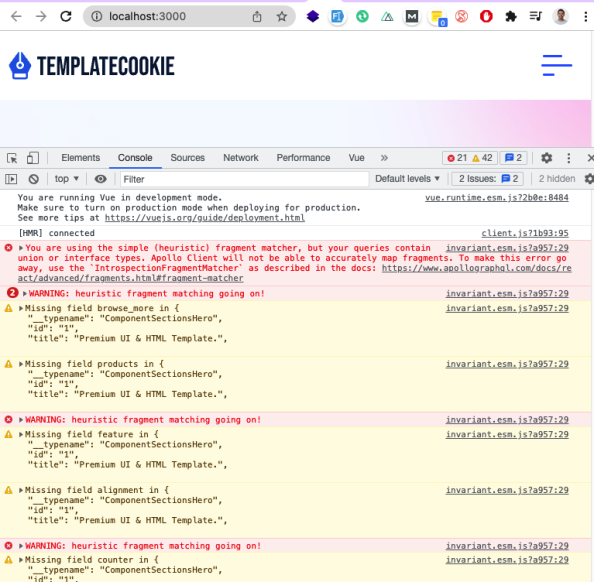