Open Hebilicious opened 1 year ago
Currently this is implemented as a module : https://github.com/Hebilicious/form-actions-nuxt
is this a stable module ?
Currently this is implemented as a module : https://github.com/Hebilicious/form-actions-nuxt
is this a stable module ?
Currently this is implemented as a module : Hebilicious/form-actions-nuxt
I would say it is still experimental. I'm looking for feedback from users to see if some things will need to be changed so if you're interested give it a try. We're working with @pi0 on getting direct nitro support https://github.com/unjs/nitro/pull/1286, which will make this module much easier to use
Edit: Starting from Nuxt 3.7.0 the module works without modification to Nitro/H3.
From a DX perspective, I think it's logical to incorporate this directly into Nuxt. Currently, being new to Nuxt, I'm finding it challenging to understand the correct approach to handle form submissions, especially since I've previously worked with Remix and have lost touch with the methods used before.
From a DX perspective, I think it's logical to incorporate this directly into Nuxt. Currently, being new to Nuxt, I'm finding it challenging to understand the correct approach to handle form submissions, especially since I've previously worked with Remix and have lost touch with the methods used before.
While I do agree with you, the module itself has very low popularity and usage at this point, so I'm not sure this is on the table at this point :/
Maybe a bit off topic but server part in nuxt is not loved on nuxt documentation. (Though a recent pull request added server section to homepage)
Nuxt has everything for building a complete server,infact great things. I would love to see Nuxt actually be recommended/ marketed as full stack framework where form actions or Astro like forms are available (btw forms in astro looks great: https://docs.astro.build/en/recipes/build-forms/).
From a DX perspective, I think it's logical to incorporate this directly into Nuxt. Currently, being new to Nuxt, I'm finding it challenging to understand the correct approach to handle form submissions, especially since I've previously worked with Remix and have lost touch with the methods used before.
While I do agree with you, the module itself has very low popularity and usage at this point, so I'm not sure this is on the table at this point :/
In that case, I'm curious about how people handle progressive enhancement or even form submissions in a seamless way. If one still needs to bind the input fields and use an e.preventDefault() function handler, then I'm not convinced..
I couldn't find comprehensive documentation on the topic, nor any convincing examples.
In the meantime, please use and recommend the module and let me know if you have any issues with it 🙏
I think that moving this into Nuxt core would be very smart. Nuxt maintainers—If you have not already read this article, please check it out for why this feature is arguably such a significant steps forward:
The Web’s Next Transition — Kent C. Dodds Progressively Enhanced Single Page Apps (PESPAs)
TLDR: Form actions not only provide the benefit of a built-in way to allow a website to work even in the absence of JavaScript (see this commonly linked flowchart), but more importantly, they allow for a dramatically simpler mental model by simplifying state management and colocating related client and server code.
Also, interest in this approach is gaining traction with both Next.js 14 getting Server Actions as of Oct 26 which can be used with forms for progressive enhancement and Remix getting Vite support as of Oct 31 which should help it's adoption. Notably, SvelteKit mentions in its docs that although API routes can be used to send data to the server, form actions "are a better way to submit data".
Sematically, Nuxt already does the equivalent of this for <a>
elements. Before JS loads, the browser default behavior simply works as a "fallback", but after JavaScript loads (or potentially if it loads) then client-side navigation is used and the links are "progressively enhanced". This feature would just extends that philosophy to <form>
elements, which is extremely powerful for DX and accessibility.
Describe the feature
Edit : Form actions have been released as a Nuxt module 🎉 Please try it and leave feedback.
Progressively enhanced patterns relies on using native forms to make it easier to have apps that works without JavaScript. This can work well with Nuxt Server components too, as it could allow client-server communication with 0client side javascript. While this is not desirable in all scenarios, and having this as the default to do everything is very opinionated, it would be nice to support these patterns at the framework level :
Multiple frameworks already support this pattern (Remix, SvelteKit, SolidStart ...), and Next is adding it :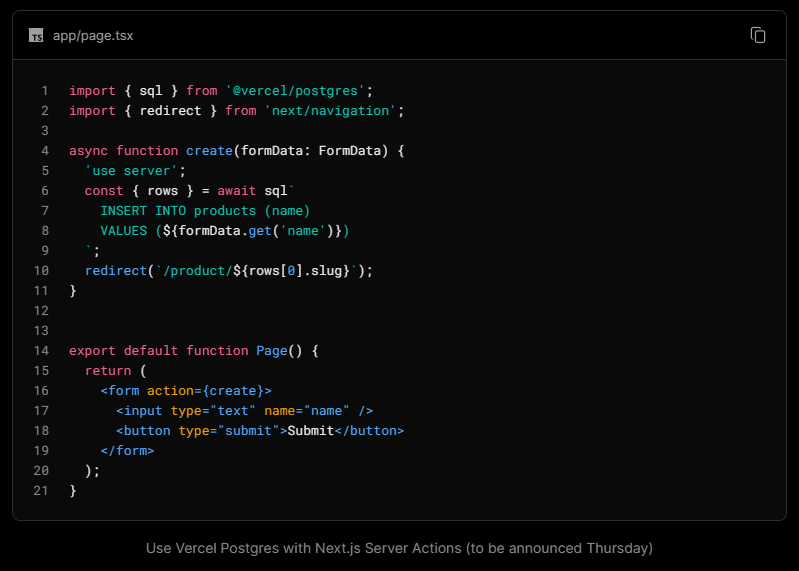
For Nuxt, it's (almost) possible already to implement this patterns by doing something like this (in /server/api/pespa.ts) :
Then creating a page at /pespa ...
However here we have to use "/api" for the action attribute, and the DX isn't the best for multiple reasons.
It would be nice to have a
/actions
directory (in/server
for nuxt) so that we can define form actions more easily. (This probably needs a discussion/RFC somewhere so that the Nuxt/Nitro/H3 side can be updated together, let me know if I should repost this somewhere else)For the nitro syntax, the
defineFormAction
I proposed above could be integrated in nitro, as a replacement for defineEventHandler.I'm not sure if manually redirecting in the action handler is the best way to do things, perhaps what would need to happen for Nuxt specifically is to generate POST only handlers for these form actions, while these GET methods to the same URL are handled by the corresponding route or nuxt page.
If I'm not mistaken, for Nuxt we also need a way to easily pass data back and forth, perhaps the existing ways can be used, but a new composable feels appropriate.
There's also a need for something similar to sveltekit use:enhance / applyAction to make it easier to progressively enhance forms (altough, e.preventDefault() could be enough, the DX is a little barebones)
Having something like
getNativeRequest
from H3 would be really useful too:This might need some change in @vue/core, see React here.
Proposed API :
I'm suggesting an API here to illustrate what we could do.
pages/signIn.vue
server/signIn.vue
To recap here :
v-enhance
to use on forms to enhance themdefineFormAction
. Could accept a function or an object of functions/server/actions
to define server actions.getNativeRequest()
andactionResponse()
to simplify the action workflowuseAction
oruseEnhance
, to work with actionResponse and v-enhance.Overall I feel like this API is respectful of the standard web semantics, and feels vue-ish. Feel free to suggest any improvements for it.
Reference from other frameworks :
Additional information
Final checks