Closed I-Own-You closed 1 year ago
this error mean you installed it failed. check the install dir i think it no dashboard folder
I have the same issue. The dashboard is installed and also works after this error message.
The error happens when setup is called outside use -> config
It works fine when all configuration is done in the use block.
this error mean you installed it failed. check the install dir i think it no dashboard folder
I have the same issue. The dashboard is installed and also works after this error message. The error happens when setup is called outside use -> config
It works fine when all configuration is done in the use block.
it doesnt work if its in config file any way
this error mean you installed it failed. check the install dir i think it no dashboard folder
no, it is installed, i reinstalled it a bunch of time, it just doesnt work
I have the same issue with external coniguration
I noticed similar problems with other plugins and I suspect this is a issue with packer (however I'm not a developer of packer and I only draw my conclusions from trial/error and running nvim --startuptime profile.log
so I might be wrong).
Use the config
key of packer to run your plugin related config like this
init.lua
require('packer').startup(
function(use)
...
use {
'glepnir/dashboard-nvim',
config = function() require('mydir/dashboard') end,
event = 'VimEnter'
}
end
)
lua/mydir/dashboard.lua
require('dashboard').setup {
-- your config here
}
Note: run :PackerSync
after making these changes.
Usually, packer executes all use instructions in order and before anything else. For a config like this
require('packer').startup(
function(use)
use 'plugin1'
use 'plugin2'
use 'plugin3'
end
)
require('subdir/plugin1conf')
require('subdir/plugin2conf')
require('subdir/plugin3conf')
the order would be
When you now include more options to the use statement that change load order, packer defers the execution of further use statements for better performance (lazy loading).
For a config like this
require('packer').startup(
function(use)
use 'plugin1'
use {
'plugin2',
after = 'plugin3' -- also happens with e.g. event = 'VimEnter'
}
use 'plugin3'
end
)
require('subdir/plugin1conf')
require('subdir/plugin2conf')
require('subdir/plugin3conf')
the order would be sth. like
This is the problem that happens with dashboard. The config is loaded before the plugin is loaded. But this can happen to all plugins that specify a custom load order and have their config after the packer startup. I had this a lot with nvim-cmp.
Packer supports the config
option to specify a command that is run after the plugin is loaded. This can be used to ensure the config is executed AFTER the package is loaded.
For a config like this
require('packer').startup(
function(use)
use {
'plugin1',
config = function() require('subdir/plugin1conf') end
}
use {
'plugin2',
after = 'plugin3',
config = function() require('subdir/plugin2conf') end
}
use {
'plugin3',
config = function() require('subdir/plugin3conf') end
}
end
)
the order would be sth. like
Using this config structure, i encountered the following problem:
plugin1
, plugin2
and plugin3
are configured at once in subdir/plugin123
The following would not work
require('packer').startup(
function(use)
use {
'plugin1',
config = function() require('subdir/plugin123') end
}
use {
'plugin2',
after = 'plugin3',
config = function() require('subdir/plugin123') end
}
use {
'plugin3',
config = function() require('subdir/plugin123') end
}
end
)
The load order would be
Instead, load order can be forced by using the after
key
require('packer').startup(
function(use)
use {
'plugin1',
after = {'plugin2', 'plugin3'},
config = function() require('subdir/plugin123') end
},
use 'plugin2'
use 'plugin3'
end
)
Here the load order would be
I noticed similar problems with other plugins and I suspect this is a issue with packer (however I'm not a developer of packer and I only draw my conclusions from trial/error and running
nvim --startuptime profile.log
so I might be wrong).TLDR
Use the
config
key of packer to run your plugin related config like thisinit.lua
require('packer').startup( function(use) ... use { 'glepnir/dashboard-nvim', config = function() require('mydir/dashboard') end, event = 'VimEnter' } end )
lua/mydir/dashboard.lua
require('dashboard').setup { -- your config here }
Note: run
:PackerSync
after making these changes.Long version
Execution in order
Usually, packer executes all use instructions in order and before anything else. For a config like this
require('packer').startup( function(use) use 'plugin1' use 'plugin2' use 'plugin3' end ) require('subdir/plugin1conf') require('subdir/plugin2conf') require('subdir/plugin3conf')
the order would be
- load plugin1
- load plugin2
- load plugin3
- source plugin1conf
- source plugin2conf
- source plugin3conf
Problem with lazy loading
When you now include more options to the use statement that change load order, packer defers the execution of further use statements for better performance (lazy loading).
For a config like this
require('packer').startup( function(use) use 'plugin1' use { 'plugin2', after = 'plugin3' -- also happens with e.g. event = 'VimEnter' } use 'plugin3' end ) require('subdir/plugin1conf') require('subdir/plugin2conf') require('subdir/plugin3conf')
the order would be sth. like
- load plugin1
- defer loading of plugin2
- source plugin1conf
- source plugin2conf (error because plugin2 has not been loaded yet)
- continue with loading plugin2
- load plugin3
- source plugin3conf
This is the problem that happens with dashboard. The config is loaded before the plugin is loaded. But this can happen to all plugins that specify a custom load order and have their config after the packer startup. I had this a lot with nvim-cmp.
Solution
Packer supports the
config
option to specify a command that is run after the plugin is loaded. This can be used to ensure the config is executed AFTER the package is loaded.For a config like this
require('packer').startup( function(use) use { 'plugin1', config = function() require('subdir/plugin1conf') end } use { 'plugin2', after = 'plugin3', config = function() require('subdir/plugin2conf') end } use { 'plugin3', config = function() require('subdir/plugin3conf') end } end )
the order would be sth. like
- load plugin1
- source plugin1conf
- load plugin2
- source plugin2conf
- load plugin3
- source plugin3conf
Bonus: Multi-package configs
Using this config structure, i encountered the following problem:
- plugin
plugin1
,plugin2
andplugin3
are configured at once insubdir/plugin123
- All 3 plugins must be loaded before the config should be executed
- The config should not be executed multiple times
The following would not work
require('packer').startup( function(use) use { 'plugin1', config = function() require('subdir/plugin123') end } use { 'plugin2', after = 'plugin3', config = function() require('subdir/plugin123') end } use { 'plugin3', config = function() require('subdir/plugin123') end } end )
The load order would be
- load plugin1
- source plugin123conf (error)
- load plugin2
- skip sourcing of plugin123conf because already loaded
- load plugin3
- skip sourcing of plugin123conf because already loaded
Instead, load order can be forced by using the
after
keyrequire('packer').startup( function(use) use { 'plugin1', after = {'plugin2', 'plugin3'}, config = function() require('subdir/plugin123') end }, use 'plugin2' use 'plugin3' end )
Here the load order would be
- load plugin2
- load plugin3
- load plugin1
- source plugin123conf
This works for me. Thanks~~ 👍
This is till an issue when using packer
during the installation and trying to use external configurations like examples show in README
@Ultimator14, what path did you specify as mydir
in use
block in your example above?
I tried the full and shortened path to the place where nvim installed the plugin but none worked:
shortened: ~/.local/share/nvim/site/pack/packer/opt/dashboard-nvim/lua/dashboard
full: /Users/leikoilja/.local/share/nvim/site/pack/packer/opt/dashboard-nvim/lua/dashboard
The path is relative to the lua folder, without the .lua extension. My config structure is like this
~/.config/nvim
|__init.lua
|__lua
|__plugins.lua <-- I have packer config in here
|__plugins
|__dashboard.lua
|__otherplugin.lua
~/.config/nvim/init.lua
...
require("plugins")
...
~.config/nvim/lua/plugins.lua
...
-- Dashboard
use {
'glepnir/dashboard-nvim',
event = 'VimEnter',
requires = {
'nvim-tree/nvim-web-devicons'
},
config = function() require('plugins/dashboard') end
}
...
~.config/nvim/lua/plugins/dashboard.lua
require('dashboard').setup {}
Well, I figured that if there was trouble trying to configure the module externally, but still the dashboard would show right away after the error message, there was no need for this line in the use statement:
event = 'VimEnter',
I removed it, run PackerInstall and relaunch… the dashboard showed correctly without error!
Seems to me like the event was forcing a premature initialization of the module.
Go ahead and try if you still have this problem. I kept my config in the after
directory, with the rest of configs.
Describe the bug I try to source the file, i get the error, i try to start neovim and i get the error
To Reproduce Steps to reproduce the behavior:
Expected behavior to work
Screenshots
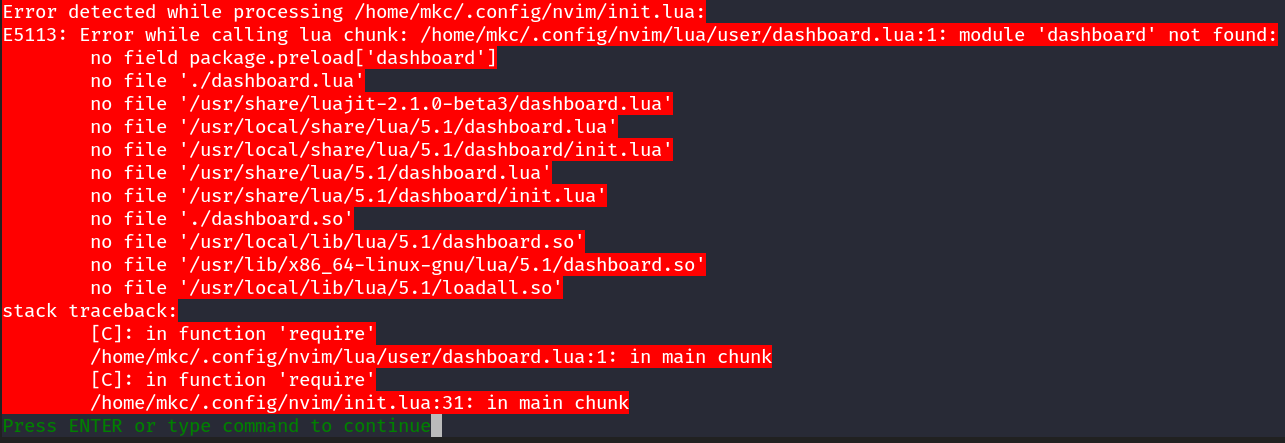