Closed innopreneur closed 5 years ago
Issue Status: 1. Open 2. Started 3. Submitted 4. Done
This issue now has a funding of 5000.0 PROCN attached to it as part of the Ocean Protocol fund.
Issue Status: 1. Open 2. Started 3. Submitted 4. Done
Workers have applied to start work.
These users each claimed they can complete the work by 2 months, 4 weeks from now. Please review their action plans below:
1) Superjo149 has applied to start work _(Funders only: approve worker | reject worker)_.
Action plan:
Questions:
Learn more on the Gitcoin Issue Details page.
Issue Status: 1. Open 2. Started 3. Submitted 4. Done
Work has been started.
These users each claimed they can complete the work by 1 month, 3 weeks ago. Please review their action plans below:
1) superjo149 has been approved to start work.
Action plan:
Questions:
Learn more on the Gitcoin Issue Details page.
2) proy24 has been approved to start work.
I propose to use Amcharts library for implementing this project. Amcharts is used to create beatiful and dynamic graphs and is free under a linkware license. https://www.amcharts.com/javascript-charts/
I propose a 7 day timeline from the date of getting confirmed. Timeline-- Build a prototype with basic functionality. i,e, render the data for bonding-curves. Add advanced querying mechanism . Filter day, month, year etc. Create unit tests for functionality. Create final version.
Questions-- Does this project expect state management via Redux, Is it ok to use a library like react-drizzle for web3 integration?
Learn more on the Gitcoin Issue Details page.
@Superjo149, welcome! Happy to have you on board! Please have a look to this tweet. There might be a code base that you could utilize or learn from. https://twitter.com/oceanprotocol/status/1052209922933436416
Hey @Superjo149, Welcome Onboard! To answer your questions -
Any specific date format for the charts?
Ideally we are looking for something like this. Minimal requirement is "max" period timeline (user can see data for the entire lifetime of particular asset.). Also, "3 months" timeline would be nice to have because initially we don't want to wait till whole year to plot data. And as you see on hover, user can also see data for a particular day.
Does the timeline chart need to have both buy and sell drop events data?
No timeline should have price -> timeline
(timeline could be hours, day, months, year etc depending on time period we plot. For (e.g.) in above image, for "max" timeline, we plot price -> year
.) plotting. Its explained in the bounty description above how to get price for a given time (independent of buy and sell operations). Think of this like a chart of price of google shares at any given time. In that users are not concerned about what is buy/sell price, they just see the price of google shares at any given point in time.
Is the price shown using calculation "price / scale" or the raw value? If so, is scale a fixed value.
What do you mean by scale? Scale of the chart (zoomed in or zoomed out) or did you mean supply
.
And feel free to ask many more questions. I will be your contact person. And to reduce latencyh in question-answer cycle, please join our Gitter channel. Its meant for technical discussions. And you will have access to many more developers (incl. me) to help you with your queries.
Issue Status: 1. Open 2. Started 3. Submitted 4. Done
Work for 5000.0 PROCN has been submitted by:
@chalidbdb please take a look at the submitted work:
Closing this bounty as it is completed.
⚡️ A tip worth 5000.00000 PROCN has been granted to @Superjo149 for this issue from @chalidbdb. ⚡️
Nice work @Superjo149! Your tip has automatically been deposited in the ETH address we have on file.
Issue Status: 1. Open 2. Started 3. Submitted 4. Done
The funding of 5000.0 PROCN attached to this issue has been approved & issued.
Bonding Curves UX bounty
How can you help?
We are looking for some help to create a React-based UX for Bonding Curves. Main aim is create a reusable React component named BondingCurve.js which can be plugged to any react application in Ocean Protocol Ecosystem. This component will interact with Ethereum Smart Contract (to read data) and will plot graphs/charts based on the data read from the Smart Contract.
What needs to be done?
Create a React standalone application (preferably using Create-React-App v2 starter kit) that has one reusable component - BondingCurve.js (apart from bootstrapped App.js).
Specifications about BondingCurve.js :
Smart Contract address (to read data from) is passed as a prop from parent (App.js).
BondingCurve.js should be able render 2 charts (in different tabs) for any given smart contract address -
(Tab Label : Timeline) Initially, this chart is plotted by going through events of Smart Contract |(hint: inside componentDidMount() lifecycle method) and renders price (from the event) vs timeline (hint: use formatted block.timestamp) (axis labels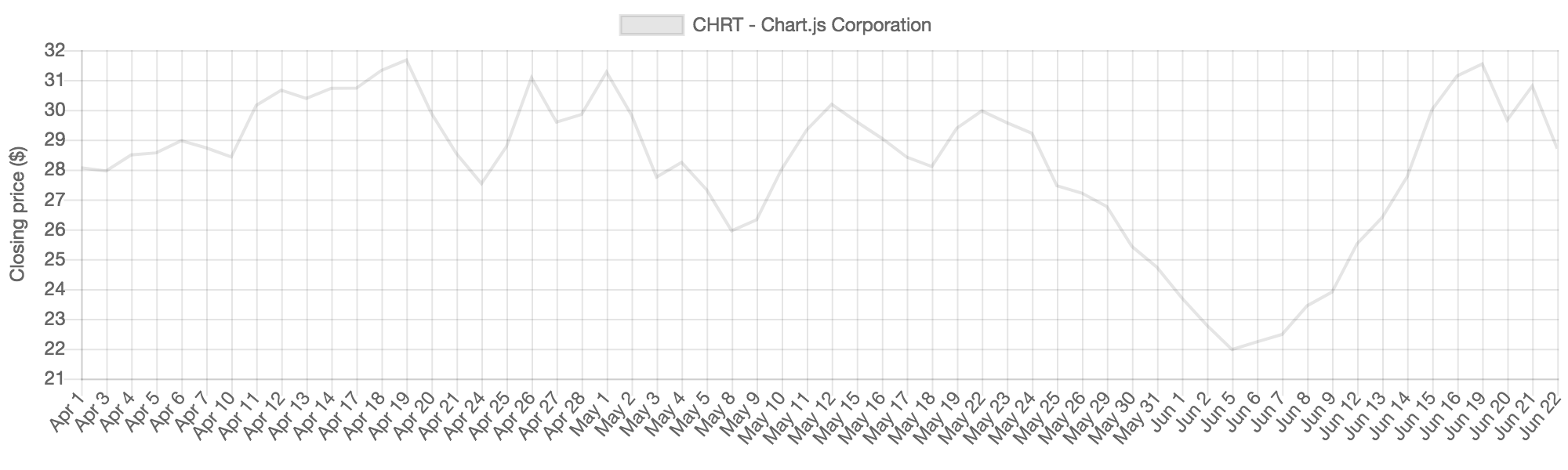
price -> timeline
). Since, events are actively fired, this component should ideally listen to events in real-time as well and dynamically render updated chart with new data points. We would like to see chart view for multiple timelines like 1 day, 1 month, maximum etc. But, atleast 'maximum' time period view should be present (others - 1 day, 1 month, 1 year etc. are optional)(Tab Label : Bonding Curve) This chart is plotted by using
query()
function of Smart Contract. Ideally, chart curve will be same for anyprice -> supply
model. So, using multiple queries enough data can be buffered to display bonding curve chart.Implementation Details
1. plotting BC as "price vs. supply"
Take a look at this test -
The key part is following for-loop, which sweep the supply from 0 to limit (i.e., 10000). It will keep buying bonded tokens with 50 Ocean tokens each time. Then, query the current supply amount and print it out. You can keep them into two arrays for plotting.
2. plot BC as "price vs. time"
In this case, you only need to listen to the event of "buyDrops" and "sellDrops" and log the price at each time of the transaction. Then plot them out in the graph with corresponding timestamp.
Tech stack should include following -
The Pull Requests must be sent to this repository: https://github.com/oceanprotocol/bondingCurvesUX
We are very happy when
When all below changes are merged with master branch
BoundingCurve.js
component which renders 2 types of charts (Timeline, Bonding Curve) based on data points retrieved from corresponding Smart ContractSeen this problem before?
Any help solving this is welcome. Feel free to leave any comments and help someone else to solve it. We might airdrop tokens to someone even if not directly completing bounty.
Questions & Reviews
Pull requests will be reviewed by one of the maintainers or long-term contributors. In case of any additional questions feel free to ask in this thread and we will do our best to add the missing info :)
Reward
This bounty is valued at 5000 PROCN tokens. Once the project was reviewed and merged in the master branch, you will receive the reward. PROCN is a proto-Ocean token. Bounty hunters that earn PROCN will be able to convert them 1:1 to Ocean tokens on network launch (currently Ocean Token is valued at 0.20 EUR). Network launch is expected to happen by Mar 31, 2019. Until then PROCN will be locked and non-transferrable in the ETH wallet to which it is delivered to.