Closed 0rangeFox closed 2 years ago
The generated bytecode from LuaJIT is not compatible with Lua. From https://luajit.org/extensions.html#string_dump Foreign bytecode (e.g. from Lua 5.1) is incompatible and cannot be loaded. To generate bytecode for Lua as luac does one needs to use the PUC Lua library instead of the LuaJIT one.
we usually use luajit -bg x.lua x.ljbc
to convert Lua to bytecode.
I think luajit can not generate bytecode compatible with luac.
The generated bytecode from LuaJIT is not compatible with Lua. From https://luajit.org/extensions.html#string_dump Foreign bytecode (e.g. from Lua 5.1) is incompatible and cannot be loaded. To generate bytecode for Lua as luac does one needs to use the PUC Lua library instead of the LuaJIT one.
Hey guys, as he said, LuaJIT bytecode isn't compatible with Lua and won't compile for Lua. But I'm disabling LuaJIT so it's suppose to use Lua, right? Could you tell me more about PUC Lua Library? Thanks.
There are two totally different Lua implementations. LuaJIT and PUC Lua (can be found here https://www.lua.org/versions.html). These are two libraries both implementing Lua and the Lua C API but use different internals. This means that when disabling the JIT in LuaJIT one still uses LuaJIT but with the JIT disabled. Disabling the JIT will not switch to a different library (PUC Lua). To use PUC Lua one would need to link against this different library instead of LuaJIT.
Hey guys, I would like to know how I can compile to bytecode via C, but I don't want to with JIT. I have tried several ways to disable JIT, but it still seems to be always on.
I have this snippet code to work with Lua C.
And the bytecode result I get is:
And if I do with the command luac, I get this: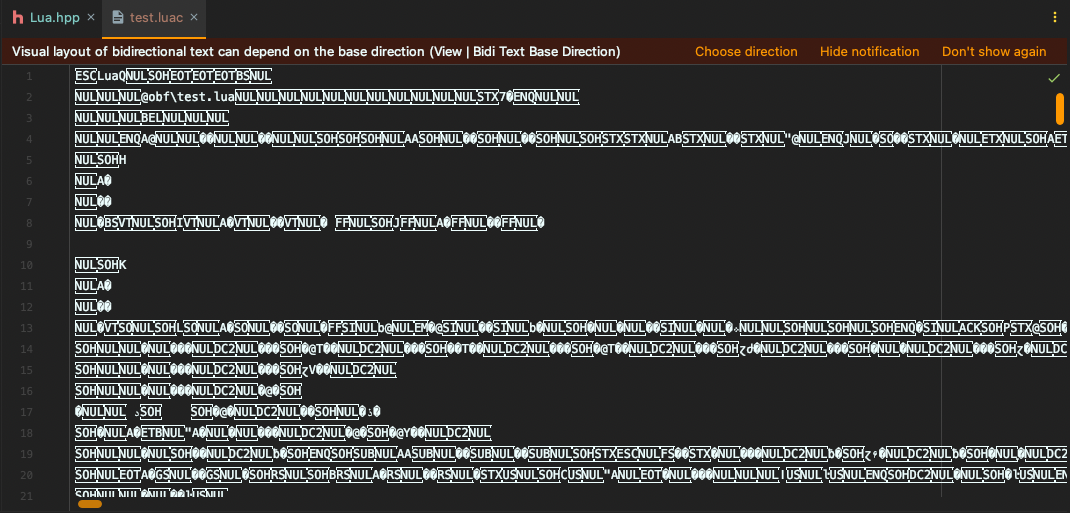
Maybe this is related to JIT, or not? Please correct me if I am wrong, I want to understand what I am doing wrong to get those results.