Closed takezoe closed 6 years ago
This pull request makes possible to trace local actor calls from Play. Here are example of traceable actors:
import jp.co.bizreach.trace.akka.actor.ActorTraceSupport._ // Message extends TraceMessage and take TraceData case class HelloActorMessage(message: String) (implicit val traceData: ActorTraceData) extends TraceMessage // Actors extend TraceableActor and inject ZipkinTraceServiceLike class HelloActor @Inject()(@Named("child-hello-actor") child: ActorRef, val tracer: ZipkinTraceServiceLike) extends TraceableActor { def receive = { case m: HelloActorMessage => { println(m.message) // Decorate actorRef with TraceableActorRef() TraceableActorRef(child) ! HelloActorMessage("This is a child actor call!") } } } // It's possible to trace nested actor calls class ChildHelloActor @Inject()(val tracer: ZipkinTraceServiceLike) extends TraceableActor { def receive = { case m: HelloActorMessage => { println(m.message) } } }
Result:
For remote actors, a message class must extend RemoteTraceMessage instead of TraceMessage.
RemoteTraceMessage
TraceMessage
case class HelloActorMessage(message: String) (implicit val traceData: RemoteActorTraceData) extends RemoteTraceMessage
In this case, the span data is converted to java.util.HashMap[String, String] automatically to serialize.
java.util.HashMap[String, String]
This pull request makes possible to trace local actor calls from Play. Here are example of traceable actors:
Result: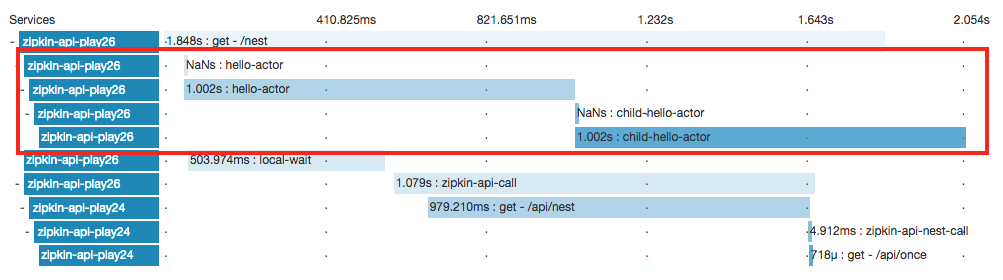
For remote actors, a message class must extend
RemoteTraceMessage
instead ofTraceMessage
.In this case, the span data is converted to
java.util.HashMap[String, String]
automatically to serialize.