Closed blogdron closed 5 years ago
worked parallax -> https://github.com/fedor-elizarov/parallax_data/tree/master/version2 need calculate Tangent camera_position and Tangent fPosition in vertex shader and after replace camera x to y and y to x and inverse y to -y https://youtu.be/FUYzaYnRJWY . Im close issue
Hi Daniel, can you help me? I do not understand if the problem is related to the engine or the fact that I am an idiot)) I try to make a parallax map, but I have a lot of distortions, if I do the calculations in the vertex shader, and if I do them in fragmentary shaders, then everything is correct only displayed on two sides of the cube. I added a video where everything is visible and the shader code
I have been suffering for a week, I do not understand how to fix it Maybe this is a feature of the data in the engine and I just do not notice?
Click in image to video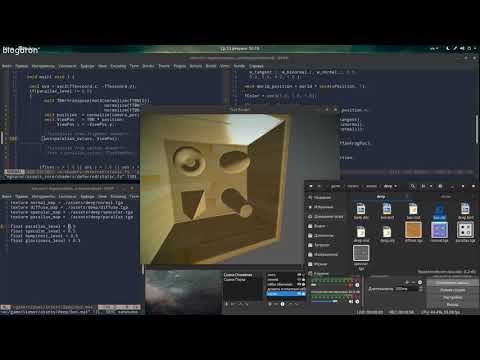
I create repo https://github.com/fedor-elizarov/parallax_data there is a collected work program (linux only)
vertex shader in assets_core/shaders/deffered/static.vs
fragment shader in assets_core/shaders/deffered/static.fs
diff input data in shader in render.c (render_static())