Closed fgrander closed 1 year ago
I appologize, there was a mistake in the Matlab code:
clear;
close all;
clc;
addpath("nurbs_toolbox"); % https://de.mathworks.com/matlabcentral/fileexchange/26390-nurbs-toolbox-by-d-m-spink
load("export.mat");
ctrlpts = permute(ctrlpts, [3,1,2]);
srf = nrbmak(ctrlpts,{knotvector_u knotvector_v});
dsrf = nrbderiv(srf);
u = linspace(0, 1);
v = linspace(0, 1);
[UU, VV] = meshgrid(u, v);
[srf_eval, deriv_uv] = nrbdeval(srf, dsrf, {u,v});
deriv = deriv_uv{1,1};
nrbplot(srf, [50 50]);
figure();
surf(UU, VV, reshape(deriv(1,:,:), numel(u), numel(v)));
xlabel('u'); ylabel('v'), zlabel('dSdu0');
figure();
surf(UU, VV, reshape(deriv(2,:,:), numel(u), numel(v)));
xlabel('u'); ylabel('v'), zlabel('dSdu1');
figure();
surf(UU, VV, reshape(deriv(3,:,:), numel(u), numel(v)));
xlabel('u'); ylabel('v'), zlabel('dSdu2');
Describe the bug I did a NURBS approximation of a 3D model in Rhino resulting in several NURBS surfaces. I calculated the derivatives for one of the resulting surfaces and figured out some strange effects. The following image shows the surface at the top left. The other 3 plots shows the x-, y- and z-part of the derivative of the surface with respect to u. In each part of the derivative there are some jumps across the surface which are obviosly wrong.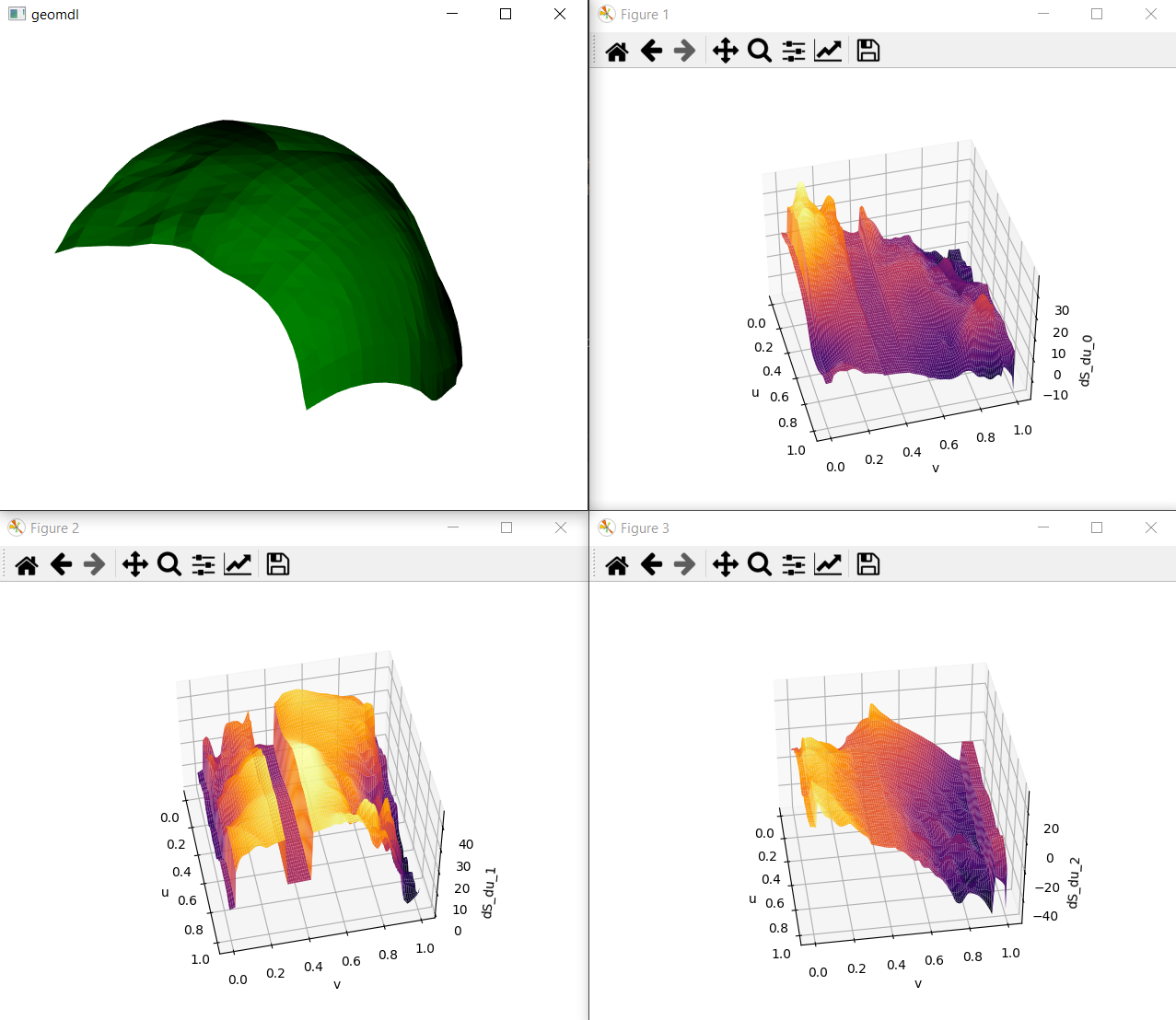
I made the same plots in Matlab using the NURBS Toolbox: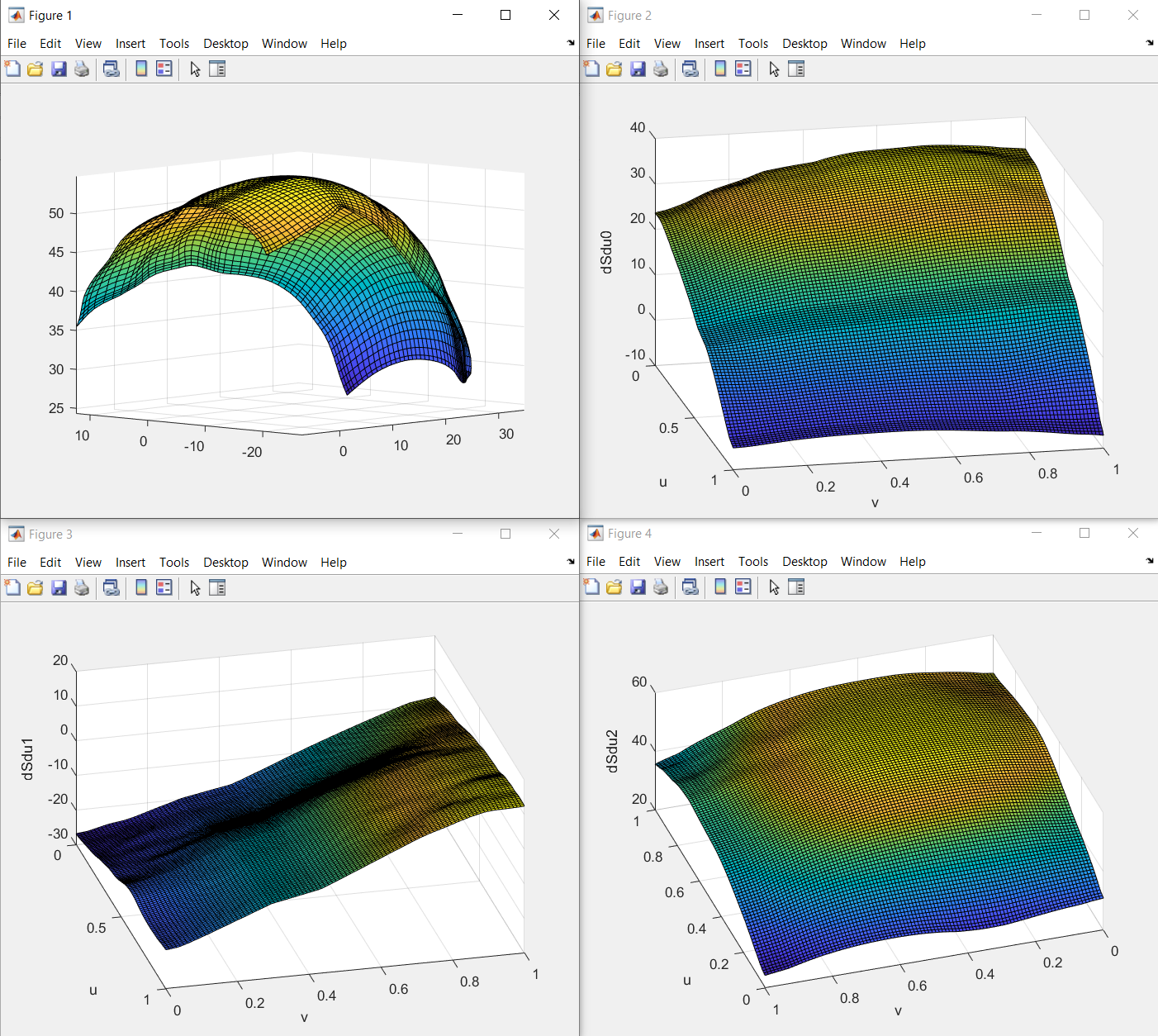
To Reproduce Steps to reproduce the behavior: I exported the surface knots vectors and ctrlpts into export.mat in the zip file. nurbs_surface.zip
Python code:
Matlab code:
Configuration: