Closed gahujipo closed 1 year ago
I followed the steps in the README and set up an emulated DS18B20 which works, even more than 8 hours in line. After that I was even able to stabilise the communication so that my master was able to find DS2438. I was able to get data from the emulated DS2438 once, but after that my master isn't able to get further data. The DS2438 remains discoverable, but doesn't return any data. I tested this with an interval of 30 seconds between each read cycle. Do you have a hint what I could try else?
After adjusting the following values
constexpr uint8_t VALUE_IPL { (microsecondsToClockCycles(1) > 120) ? 25 : 21 }; // instructions per loop
constexpr timeOW_t ONEWIRE_TIME_SLOT_MAX[2] = { 148_us, 42_us }; // should be 120, measured from falling edge to next falling edge
like mentioned here: https://github.com/orgua/OneWireHub/issues/43#issuecomment-325297441 I am able to communicate for aprox. three hours with the emulated sensor where every second operation fails with an poll interval of 25sec. Has anyone further suggestions to stabilise the bus?
The DS2438 remains discoverable, but doesn't return any data.
I see exactly the same thing here with Loxone system.
I discovered a different version of DS2438. The code wasn't on Github but I copied it for a very basic MQ135 sensor:
https://github.com/raintonr/MQ135As1W
This works perfectly. Been stable here for months.
However, I recently wanted to do something more complex involving using setVADVoltage
and setVDDVoltage
(new methods in code linked above). When trying to use them both they often get reported as zero for some reason. Will try and figure that out.
On this topic, I found the following very useful page describing DS2438 communications and the mechanism to read VAD or VDD:
https://eds.zendesk.com/hc/en-us/articles/214484863-Working-with-the-DS2438-using-the-HA7E-HA7S
When a DS2438 is configured in Loxone it always reads both VAD and VDD. It's true to say, that due to other limitations in Loxone (regarding number of sensors supported on a bus) it could be useful to have 4 values presented on a single sensor, so reading the extra voltage could be useful, but how many bytes on the wire does it 'cost' to get the single, extra 10 bit voltage value?
Looking at the above link, seems like 270-ish bytes (both TX/RX) are required to configure the A/D bit, initiate voltage conversion and read results (well - if I've understood it correctly, please tell me if that's not the case).
If that is correct, using a DS2438 sounds a terribly inefficient way to get just one extra 10 bit value and one would likely be much better off emulating multiple DS1820 (or similar) devices.
In case I didn't make it clear, PR #97 seems to fix the DS2438 problem with Loxone here.
Found this through trial and error seeing which of the changes in the modified version I linked above made things work. Just looks like it was because sometimes calcCRC was skipped & guess Loxone may be causing this skip, or actually checking CRCs where other masters don't?
3fc416dece2b5c1d18fb7d6adb46abc45840189b enables emulation of VDD/VAD voltage reading which Loxone users should find useful.
I tried to emulate a DS2438 on a Wemos D1 and find the "sensor" with Loxone. The Wemos D1 has only 3,3V pins so I used a level shifter to rise the signal to 5V. But it couldn't be found.
My wiring scheme is this: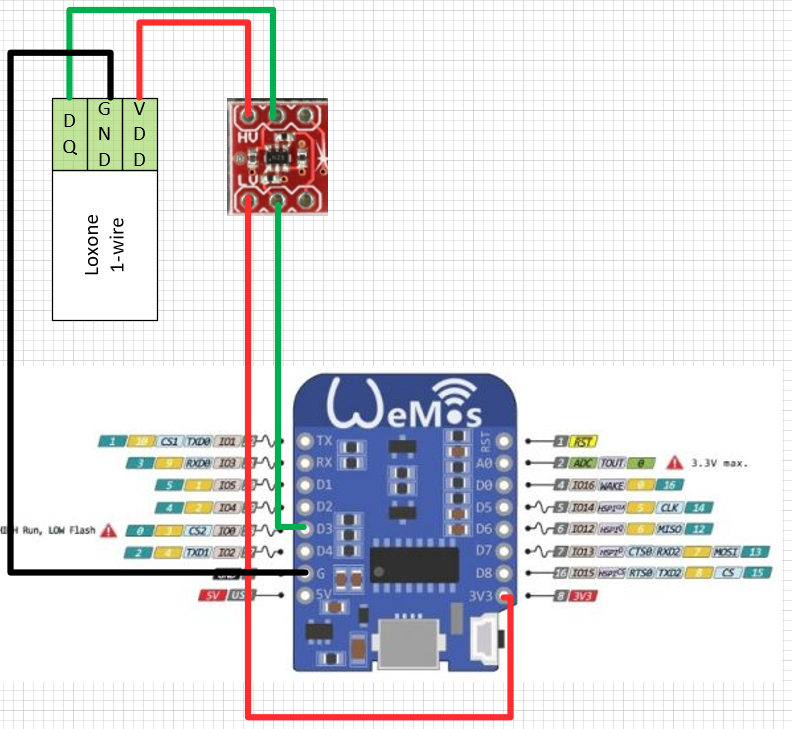
My current code is this
Is this correct or am I missing something?