Open nezix opened 2 years ago
Hi @nezix ,
I actually forgot the mesh create from data function accepted vertex colors. Your mesh above is white because nvisii is reading your material component, which has its base color set to white. We don’t currently read per-vertex colors during rendering.
What I don’t like about vertex colors is that there’s an ambiguity here between whether to use the material base color or the mesh vertex colors. Those ambiguities tend to result in ill defined behavior like you’re seeing here.
My solution here would probably be to remove that vertex color input from the mesh creation function, since it isn’t used. Do you have a specific reason why you’re using per-vertex colors rather than the material base color?
Hi, Thanks for your answer. It's pretty common in my field (molecular visualization) to procedurally generate meshes and vertex coloring is an easy way to color meshes dynamically based on an atom property. We generally blend the two colors together (baseColor x vertexColor). The alternative is more involved as it requires to proceduraly create a texture. I tried not to set the base_color but it does not seem to take vertex colors into account anyway.
Gotcha. Yeah, that could work. If both material base color and vertex color are accounted for, then there’s no longer this Ill-defined behavior issue.
I’ll mark this as an enhancement, since your use case makes sense to me.
Setting colors per vertex does not seem to work on 1.1.72 / Windows 10
Here is a simple example script to try:
I expect to have a red dodecahedron but the mesh is white: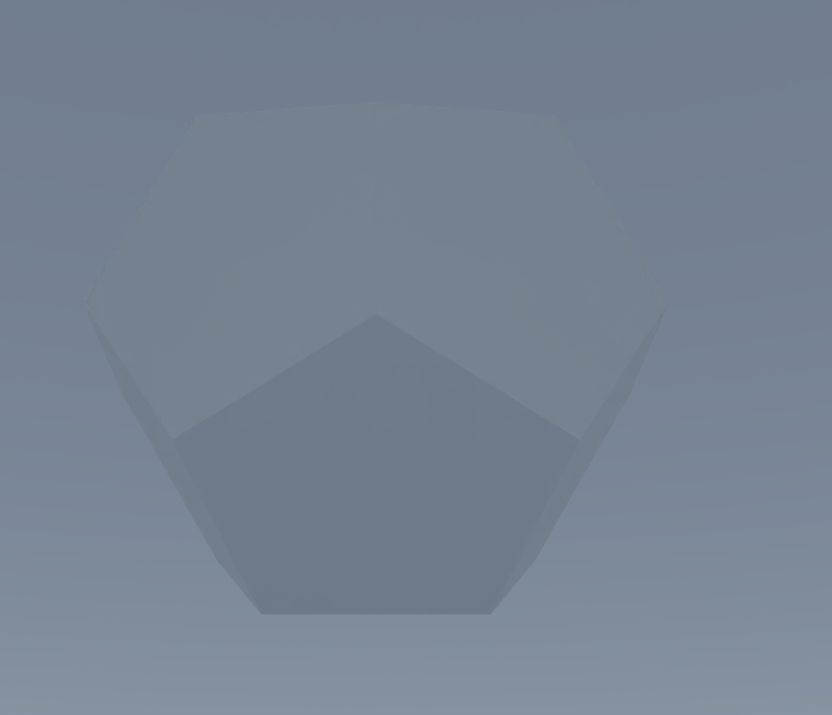