Closed palashborhanuddin closed 3 years ago
@palashborhanuddin
JDeodorant models the entire try-catch
structure as a single PDG Control Predicate node.
For the sake of Groum generation, we don't care so much about how exactly the exception handling is done (in other words, what exactly happens inside the catch blocks
). We mainly care about what exception types are handled.
So, we can model try-catch
as a single node in the Groum representation, and keep as metadata information the exception types handled in the catch
blocks.
Thanks, what about the finally block? For example, from the above code snippet, shouldn't we have the GROUM nodes for the possible ACTION/CONTROL nodes inside the finally block?
@palashborhanuddin
The same principle holds for finally
blocks. Typically, in the finally
block developers handle some resources used within the try
block (e.g., close streams, connections).
With the new try-with-resources
this handling is automatically done by Java.
Therefore, in order to abstract the try
blocks, we will omit the implementation details inside finally
block.
We can keep as metadata, only the fact that exists a finally block, in the same way we just kept the exception types being handled in the catch
blocks.
Thank you.
nodes=[1 Scanner.<init>, 2 FOR, 4 Scanner.nextLine, 3 String.trim, 6 String.equalsIgnoreCase, 5 IF, 7 File.<init>, 9 File.exists, 8 IF, 12 File.canRead, 10 IF, 11 File.isFile, 13 Scanner.<init>, 15 Scanner.hasNextLine, 14 WHILE, 16 Scanner.nextLine, 19 File.isDirectory, 18 IF, 21 File.getCanonicalPath],
edges=[
, 1 Scanner.<init>-->2 FOR
, 2 FOR-->4 Scanner.nextLine
, 4 Scanner.nextLine-->3 String.trim
, 3 String.trim-->6 String.equalsIgnoreCase
, 6 String.equalsIgnoreCase-->5 IF
, 5 IF-->7 File.<init>
, 7 File.<init>-->9 File.exists
, 9 File.exists-->8 IF
, 8 IF-->12 File.canRead
, 8 IF-->11 File.isFile
, 12 File.canRead-->10 IF
, 11 File.isFile-->10 IF
, 10 IF-->13 Scanner.<init>
, 13 Scanner.<init>-->15 Scanner.hasNextLine
, 15 Scanner.hasNextLine-->14 WHILE
, 14 WHILE-->16 Scanner.nextLine
, 10 IF-->19 File.isDirectory
, 19 File.isDirectory-->18 IF
, 18 IF-->21 File.getCanonicalPath
, 1 Scanner.<init>-->3 String.trim
, 1 Scanner.<init>-->4 Scanner.nextLine
, 3 String.trim-->7 File.<init>
, 6 String.equalsIgnoreCase-->7 File.<init>
, 7 File.<init>-->12 File.canRead
, 2 FOR-->8 IF
, 7 File.<init>-->11 File.isFile
, 9 File.exists-->12 File.canRead
, 9 File.exists-->11 File.isFile
, 7 File.<init>-->13 Scanner.<init>
, 2 FOR-->10 IF
, 9 File.exists-->13 Scanner.<init>
, 12 File.canRead-->13 Scanner.<init>
, 8 IF-->10 IF
, 11 File.isFile-->13 Scanner.<init>
, 7 File.<init>-->19 File.isDirectory
, 9 File.exists-->19 File.isDirectory
, 12 File.canRead-->19 File.isDirectory
, 11 File.isFile-->19 File.isDirectory
, 7 File.<init>-->21 File.getCanonicalPath
, 2 FOR-->18 IF
, 19 File.isDirectory-->21 File.getCanonicalPath
, 10 IF-->18 IF
, 9 File.exists-->21 File.getCanonicalPath
, 12 File.canRead-->21 File.getCanonicalPath
, 8 IF-->18 IF
, 11 File.isFile-->21 File.getCanonicalPath
, 13 Scanner.<init>-->16 Scanner.nextLine
, 10 IF-->14 WHILE
, 15 Scanner.hasNextLine-->16 Scanner.nextLine
Node and edges for the above test code.
Note that, there is no node TRY, CATCH or FINALLY statement (also statements inside CATCH and FINALLY) as there is no PDG edge for these!
PDG generated using JDeodorant,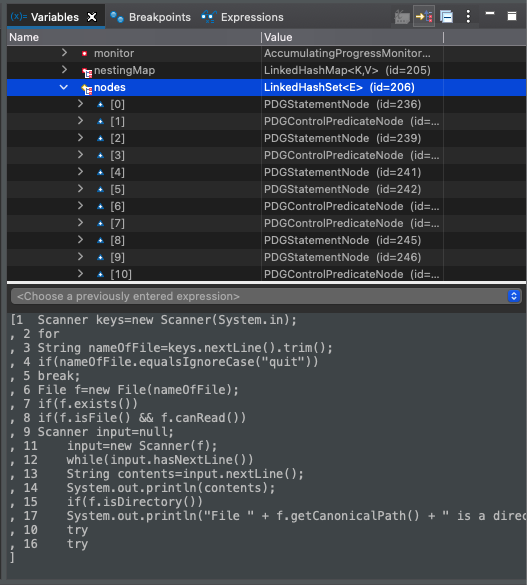
Question, why there is no PDG Node for the statements inside catch and finally blocks?