class Message(db.Model):
id = db.Column(db.Integer, primary_key=True)
message_text = db.Column(db.Text, nullable=True)
stickers = db.relationship('StickerMessageAssociation', back_populates='message')
...
and
class Sticker(db.Model):
id = db.Column(db.Integer, primary_key=True)
file_id = db.Column(db.String(255), nullable=False)
messages = db.relationship('StickerMessageAssociation', back_populates='sticker')
...
and an intermediate model to connect these two:
class StickerMessageAssociation(db.Model):
__tablename__ = 'message_to_sticker'
message_id = db.Column(db.Integer, db.ForeignKey('message.id'), primary_key=True)
sticker_id = db.Column(db.Integer, db.ForeignKey('sticker.id'), primary_key=True)
message = db.relationship('Message', back_populates='stickers')
sticker = db.relationship('Sticker', back_populates='messages')
The problem arises when I try to enable support for assigning stickers to the messages in the flask-admin via inline model editing:
class InlineStickerModelForm(InlineFormAdmin):
form_label = 'Stickers'
def __init__(self):
return super(InlineStickerModelForm, self).__init__(StickerMessageAssociation)
class MessageView(ModelView):
...
inline_models = (..., InlineStickerModelForm())
When I add a sticker to a message in the admin panel, which looks like this:
and try to save the page, I get the following error:
Tried to hack my way around scaffold_inline_form_models and in a few other directions but no luck so far. Any suggestions would be very much appreciated. Thank you!
Hi! I have two models
The problem arises when I try to enable support for assigning stickers to the messages in the flask-admin via inline model editing:
When I add a sticker to a message in the admin panel, which looks like this: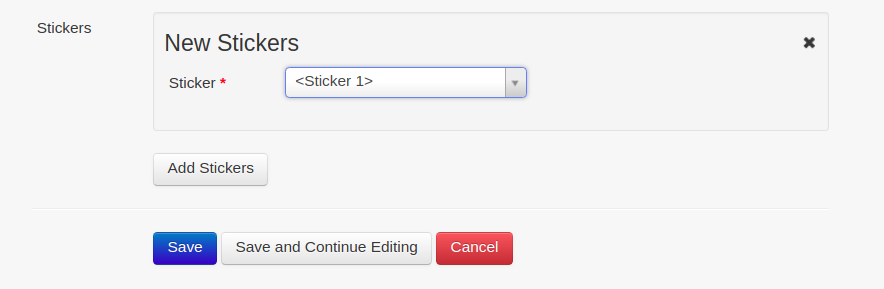
and try to save the page, I get the following error: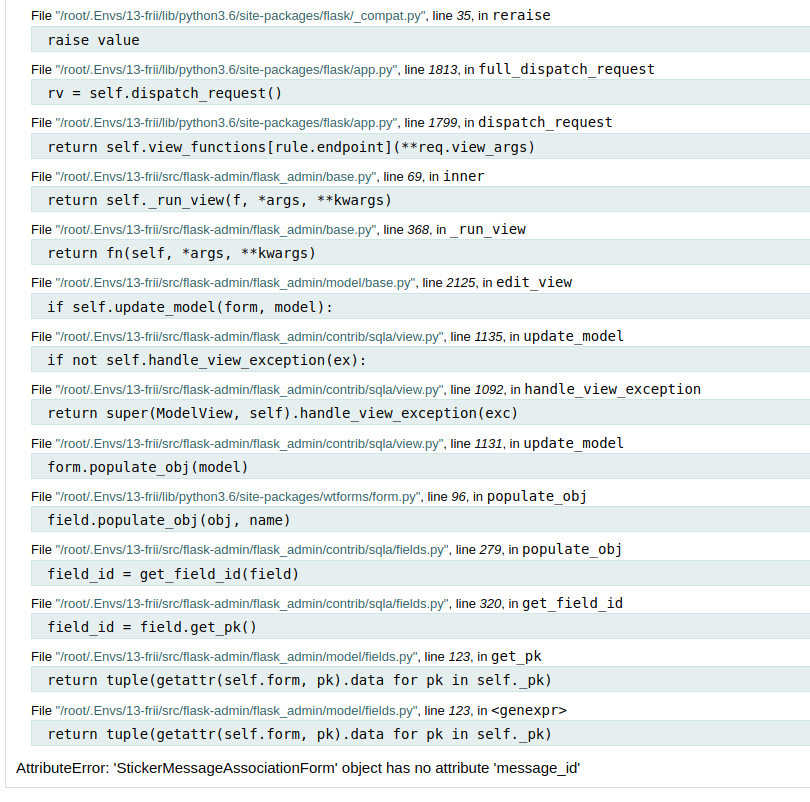
Tried to hack my way around
scaffold_inline_form_models
and in a few other directions but no luck so far. Any suggestions would be very much appreciated. Thank you!