Closed xiaodaxia-2008 closed 3 years ago
Hi @xiaodaxia-2008,
this is desired behaviour: Ruckig will first and foremost try to get the kinematic state within the allowed limits as fast as possible. Only then a time-optimal trajectory is calculated.
In your example, if the velocity wouldn't get negative in the top right figure, the trajectory would stay slightly longer above the velocity limit. If the velocity would decrease to +1 directly, a positive jerk would be necessary while the velocity would still be above its limits. I hope that clears up this issue.
However, we can think about a setting that disables the braking trajectory. I'm not sure how hard this would be to implement.
Thanks for your explanation. By using online trajectory filter, I have obtained the following expected result. But time synchronization for serveal dofs could not be done.
By the way, I think it will be help to add current_jerk
attribute to InputParameters
if possible.
What do you mean by online trajectory filter exactly?
As the derivative of the jerk is not limited in Ruckig, the current jerk is not relevant for the calculated trajectory.
Please refer to Trajectory Planning for Automatic Machines and Robots chapter 4.6 Nonlinear Filters for Optimal Trajectory Planning
.
So the current jerk should always be max_jerk
or -max_jerk
?!
Thanks for the link!
As there is no current jerk in ruckig, I'm not sure what you mean?
I think it is the 3rd derivative of the calculated trajectory. As the trajectory is at most polynomial of degree 3, so the jerk is constant at each segment.
Thanks for your explanation. By using online trajectory filter, I have obtained the following expected result. But time synchronization for serveal dofs could not be done. By the way, I think it will be help to add
current_jerk
attribute toInputParameters
if possible.
could you send me this copy of the one using online trajectory filter. oridong@126.com
If the contraints are changed online, then the trajectory is not optimal as it should be. The following image shows the whole trajectory, at t = 2, max_velocity is reduces from 3 to 1, then the velocity goes to negative unexpectedly.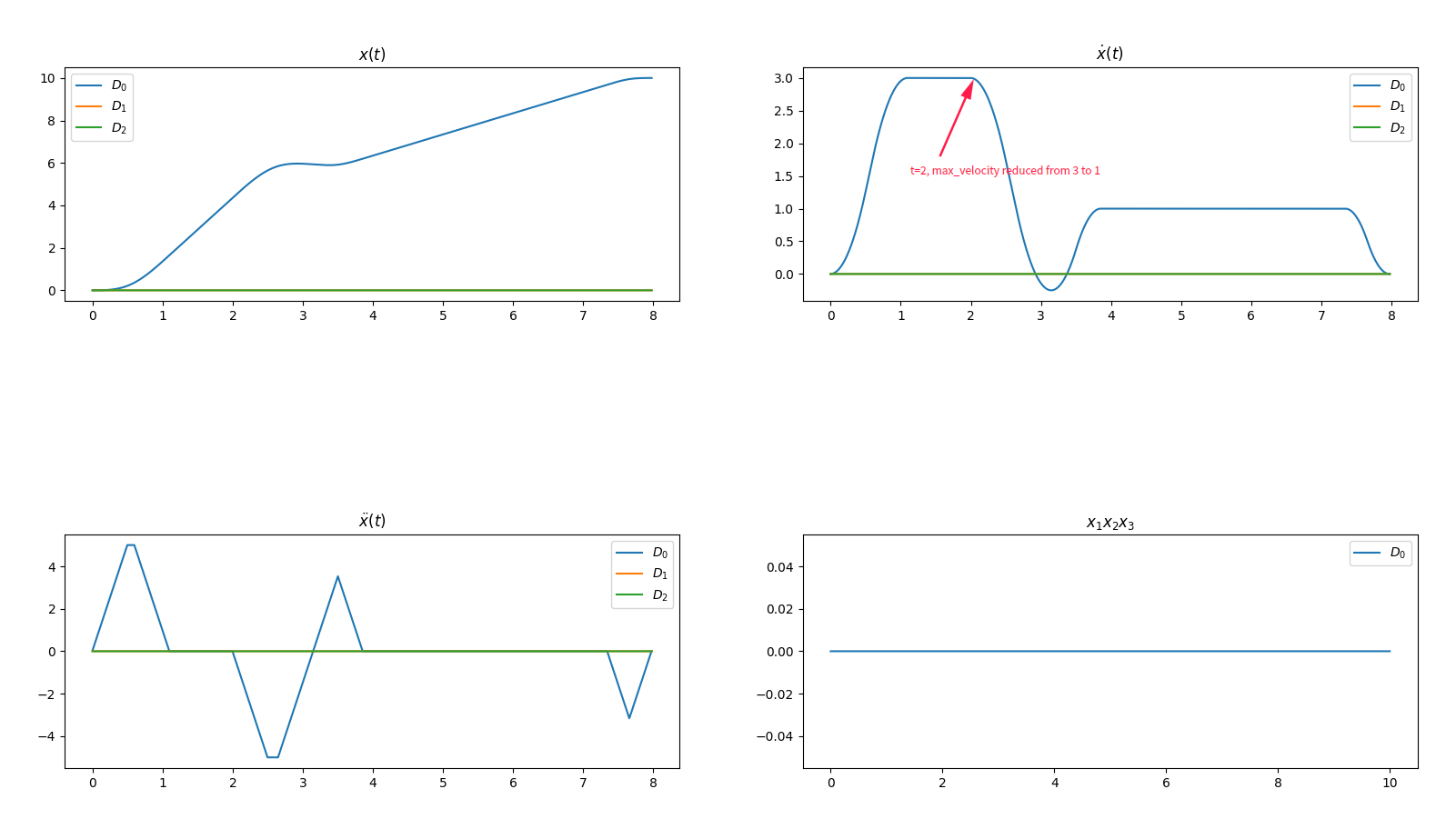
On the other hand, if the velocity constraints increases, everything seems to be fine.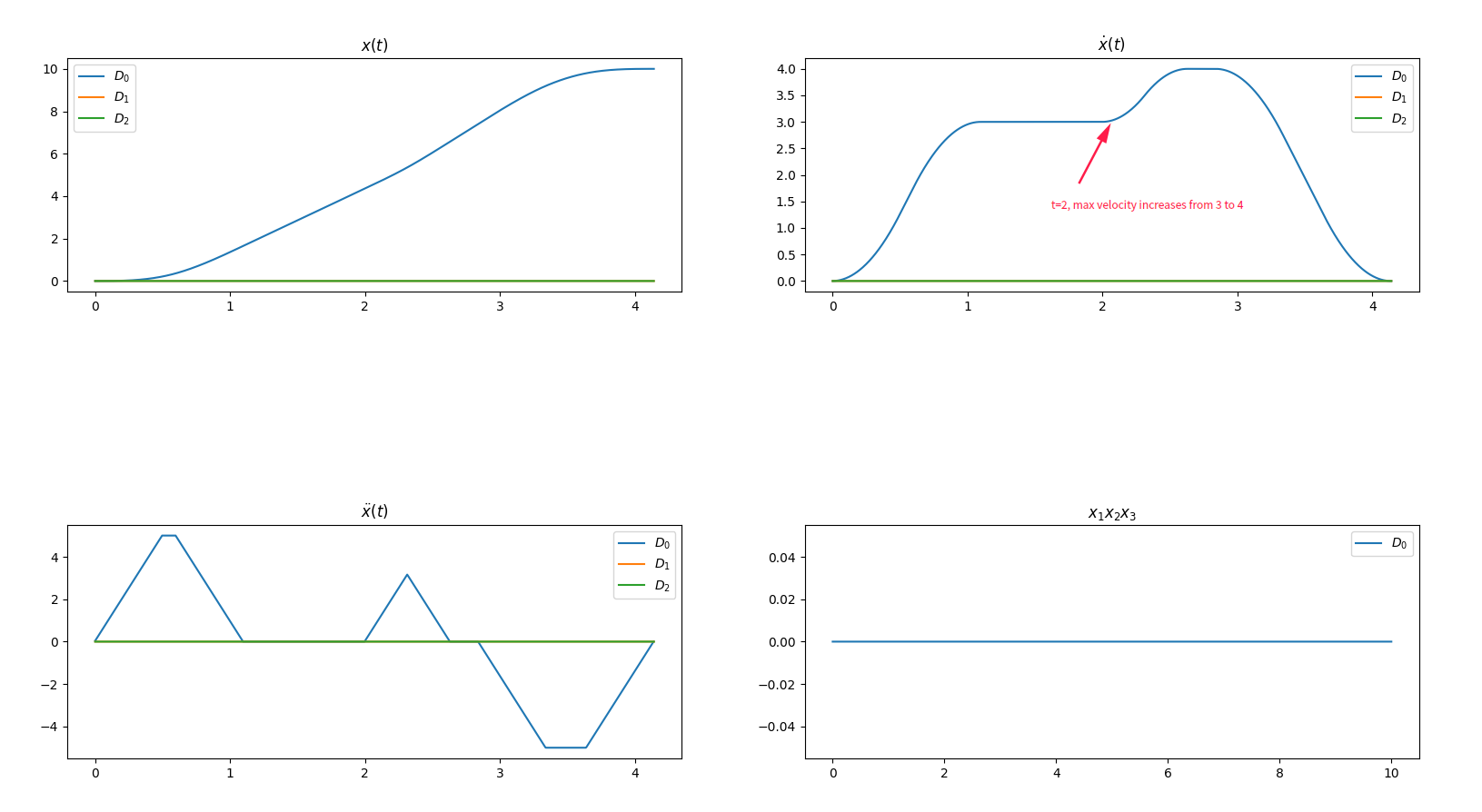
You could reproduce with the following code: