Closed w0w closed 6 years ago
I will have a look at it, as soon as "my other work" let me to do so ;-)
Some questions from my side:
brzo_i2c
errors, right?I had a (quick) look at the datasheet (wrong link in your comment, but don't worry, I found the correct one). AFAIK, there is no way to read out the configuration or status register, right? Otherwise, we could have checked that the values were written correctly (assuming there are no read errors). Even if the the write, i.e. bits 6 and 5, was not successful (i.e. writing 0), the power-up values of these would be 0 0, so the SCAN selection mode should not be affected.
What is the size of your pullup resistor on SDA and SCL?
4.7k
Which esp-board are you using?
esp8266 nodemcu 1.0
Which version of Arduino IDE and esp8266 core do you use?
Latest
You don't get any brzo_i2c errors, right?
No brzo errros
Do you get back different values, or always the same, independant of what happens on the analog inputs of the MAX11615?
I have figured out a way to work with wire as of now but i want to use brzo and i am trying to understand more about the code, if you can point me to any reading material it will be great.
I think it worked and i have narrowed down the error to bit shifting and noise from the power supply.
I don't have any other i2c setup as of now.
Do you have a scope or logical anaylizer?
Not yet, i am ordering one soon.
It must be a stupid question to ask but what is the console.log equivalent in assembly ?
Thanks for additional info. Just a small remark: I have not yet tested brzo_i2c
with the latest Arduino core , i.e., 2.4-rc*. But normally this is not a problem...
So, if I understood you correctly, i2c communication is working?
Concerning assembly language question (which is not stupid question at all): Well, debugging is always a bit difficult with these microcontrollers. It strongly depends on the IDE you are using, how good or bad it is supported. This is also true for (inline) assembly language. At least from the docs, the Xtensa Xplorer IDE seems to give good support also for assembly, i.e. you can observe the contents of the registers etc; but I am not using it.
Otherwise, since you are on the instruction level with (inline) assembly, the only way to go is to think and think and think again before implementing something ;-) ....and to "debug" like this: If possible, split up inline assembly in parts, pass values from register to the outside world (i.e. c/c++) and then print them to the console.
A help for me to debug brzo_i2c
was the rigol scope: I could know from the observed signals, where in the assembly code something probably went wrong.
I am implementing i2c communication with MAX11615 and i am not getting desired result. Below is a snapshot to give more clarity on the slave. Datasheet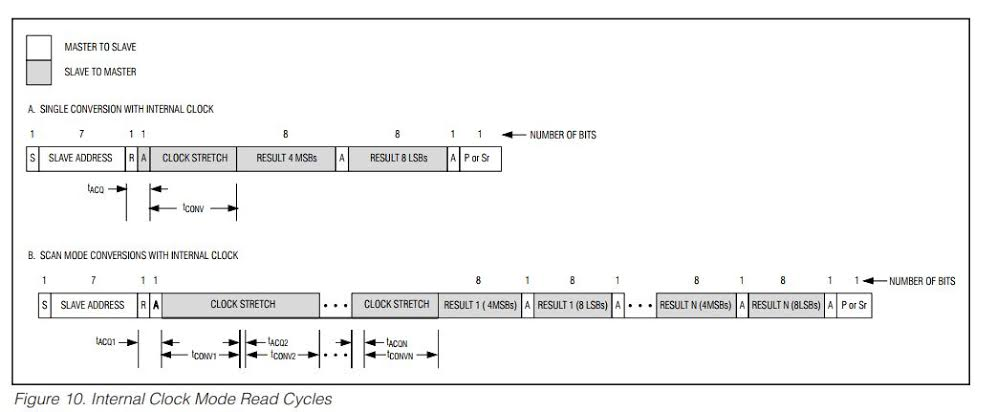
My code looks like
What is funny is the values are same for a_code, b_code, c_code, d_code. The setup and configuration byte is proper and checked with manufacturer. Is it possible that the write is not happening correctly and hence giving value from only one channel ? I am not very versed with writing assembly so not sure how to debug this.