Closed jantonso closed 2 years ago
This is possible, indeed, although I took precautions to not call the flushSync where it's not necessary (it's needed for react 18). Can you strip as much as possible from your logic and replicate the problem in a codesandbox?
@jantonso I am closing this, once you provide a runnable repro, I will take a look.
Describe the bug We were previously using
react-virtuoso@v2.10.2
withreact@16.14.0
andreact-dom@16.14.0
, which was working great!In a recent update, we upgraded to using the latest
v2.16.6
version ofreact-virtuoso
, which is causing the following error to be thrown when scrolling up/down a large list of items.Screenshots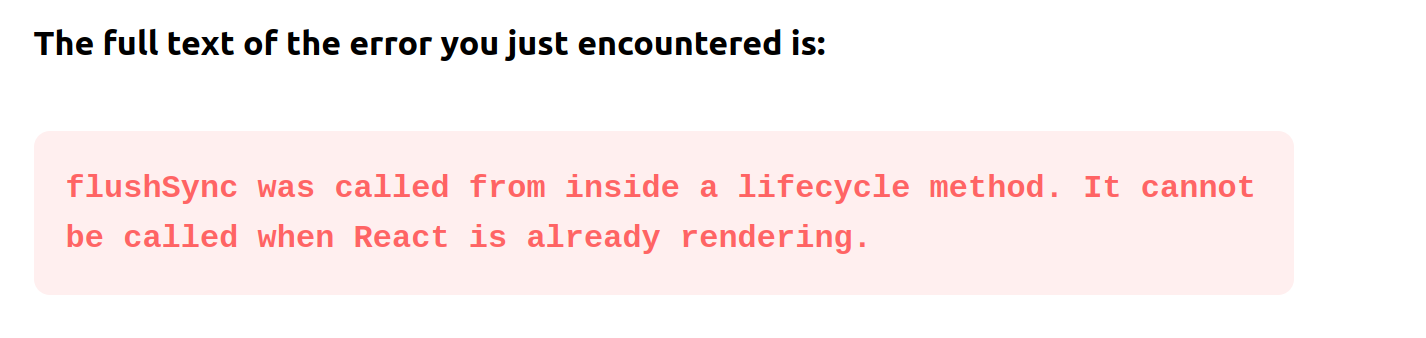
Desktop (please complete the following information):
Additional context