Closed javleds closed 2 years ago
Very nice work! I'd like to see the message suggestion implemented before we merge this pull request.
Very nice work! I'd like to see the message suggestion implemented before we merge this pull request.
Thanks @eruizdechavez , what do you mean with see the implementation?
If it is at code level, we already use it in the PlusPlus conversation to build a complex message:
First of all, we create the builder (line 35).
$replyParameters = new AdditionalParametersBuilder();
If the user try to increase its own score, we add a new message (in md) and also a divider, to split message types (line 43).
$replyParameters->addMarkdown($message)->addDivider();
For each modified score, we add a new part of the text in md as well (line 54).
$replyParameters->addMarkdown($message);
Finally, when we want to reply, we build the message (line 57):
$bot->replyInThread('Points updated!', $replyParameters->build());
^^
is that what you mean?
I'd like to see the message suggestion implemented before we merge this pull request.
Thanks @eruizdechavez , what do you mean with see the implementation?
@javleds what I mean I want to see it actually implemented with environment variables, SQLite (as I suggested) or any other alternative someone proposes instead of the config files.
I am also flexible if you think that is extra work that should be done on a follow up pull request.
The origin of the messages were moved from the parametrs.yaml
to a database table.
This PR is a little bit longer, the main idea was to give a solution for the issue https://github.com/phpmx/phpmxbot/issues/25, nevertheless, to accomplish that required 2 changes:
In addition, and it is open to suggestions, we added the username and the score of the affected users.
The final output is similar to this: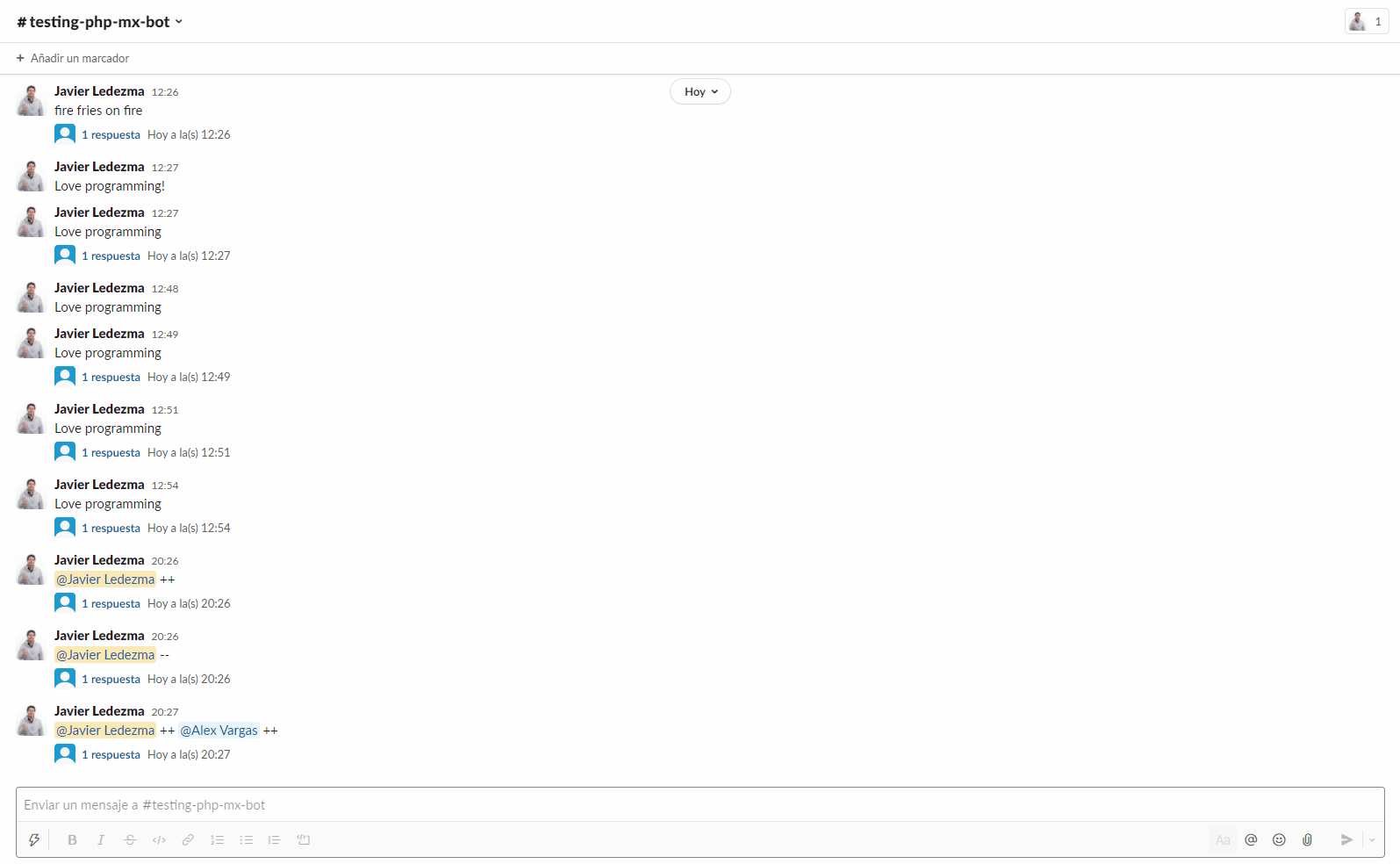
I will append a couple of segments that can be added to the wiki in case this PR gets approved, one for the class created to buid the message and other to replace the custom messages.
AdditionalParameters
Build markdown messages for slack can be a little bit hard since we have to create a complex array structures called
AdditionalParameters
. In that structure we have to define blocks, sections and fields.This data structure should be serialized in order to be processed by the BotMan and finally give a readable message on Slack.
In example, in order to send the following message:
We have to generate the following structure:
Painful, right?
To make easy the creation of that data structure we propose the
AdditionalParametersBuilder
class which builds this complex array using natural and fluent language.In order to build the previous example we have to use the following code block:
Replace the custom messages
We have created the
config/parameters.yml
file with 3 important entries:In order to change, add or remove any message we have to edit those array properties. Also we have created 2 placeholders,
{user}
and{score}
which will be replaced for the real username and score of the leaderboard.In example:
Will be rendered as: