Closed anderslyman closed 2 years ago
PR'd - I realize this repo has seen no activity in 4 years, but it's here if someone wants it.
PR'd - I realize this repo has seen no activity in 4 years, but it's here if someone wants it.
Thank you very much for this PR! We'll hope it will be merged in main. =)
There is one more critical error. This serializer does not preserve Kind property.
Let's modify your example:
var dtB = new DateTimeExample { Date = DateTime.UtcNow };
Then
DateTimeExample actualDt = ByteArrayToObject<DateTimeExample>(bytesB);
Now let's examine actualDt.Date.Kind :
if (actualDt.Date.Kind != DateTimeKind.Utc)
throw new InvalidOperationException($"Expected Kind is UTC, but it is '{actualDt.Date.Kind}'!");
I've just merged the PR of @anderslyman. Thanks! Additional tests report no errors concerning XML serialization of DateTimeKind. However, there are errors during the binary serialization, confirming the problem reported by @AntiTenzor. The problem is extracted there: #20
When serializing/deserializing
DateTimeOffset
properties, the date is set to the default value of1/1/0001 12:00:00 AM +00:00
. Conversely,DateTime
properties work as expected.LINQPad script: http://share.linqpad.net/i8covu.linq
Yields: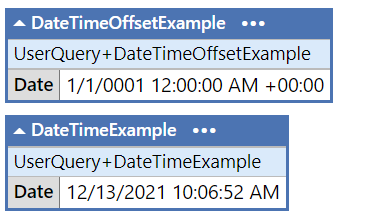