Open pomelovico opened 4 years ago
官方介绍:https://classic.yarnpkg.com/zh-Hans/docs/workspaces/
总的来说,它就是一个比npm link
更好的方式去管理多包开发的方式,npm link
使得我们可以不用发布就可以在其他项目里使用本地的一个包,但是这会影响到全局的npm 系统,而yarn的workspace将这个影响降低到一个工作目录下。
我们在进行react项目开发时,可能会使用antd组件库,但由于UI规范,我们需要对antd组件库进行二次改动(俗称魔改:smiling_imp:),所以做法是fork 一份antd官方代码到本地,与我们的项目代码放到一起,目录结构大致如下:
- packages
- business // 业务代码
- ant-desgin // 组件库代码
- package.json
然后在根目录下的package.json
文件中声明启用yarn workspace功能:
"private": true,
"workspaces": {
"packages": [
"packages/*"
],
},
components
,然后将其下的package.json中的name改为@own/component
,然后我们在业务项目business
里添加包依赖:
// business/package.json
"dependncies":{
"@own/component": "0.0.1"
}
重点来了:,然后再执行yarn install
的时候,yarn就会在node_modules目录下生成一个@own/component
的软连接指向packages/components了,而不会从npm网络仓库寻找这个包:
/node_modules/@own/component -> /packages/components
这样一来,我们成功的在一个工作目录下维护了两个包,这两个包的代码也可以放在同一个git仓库中,方便管理,相比link的方式,从项目角度来说更加内聚。当我们对组件库进行了修改后,只需要重新编译一次,即可在业务代码中使用了
启用了workspace之后,yarn会把每个包的依赖尽量提升到根级node_modules目录下存放,如果需要避免yarn将 依赖安装到root下,可以使用nohoist
字段声明依赖
antd-tools
antd-tools 是antd组件库打包使用的工具,集成了编译,发布等操作,实现了按需加载的打包方式,接下来一一说明
gulp工作流
antd-tools采用gulp进行打包,入口文件在
lib/gulpfile.js
,所有的命令都在gulpfile中注册antd-tools run compile
编译组件库,同时并行编译了两种代码,分别到
es
目录和lib
目录,其区别是,es
目录是支持ES module规范的代码(import),而lib
目录下的代码是支持commonJS规范的代码(require)重点是这个
compile
函数:编译过程示意图如下: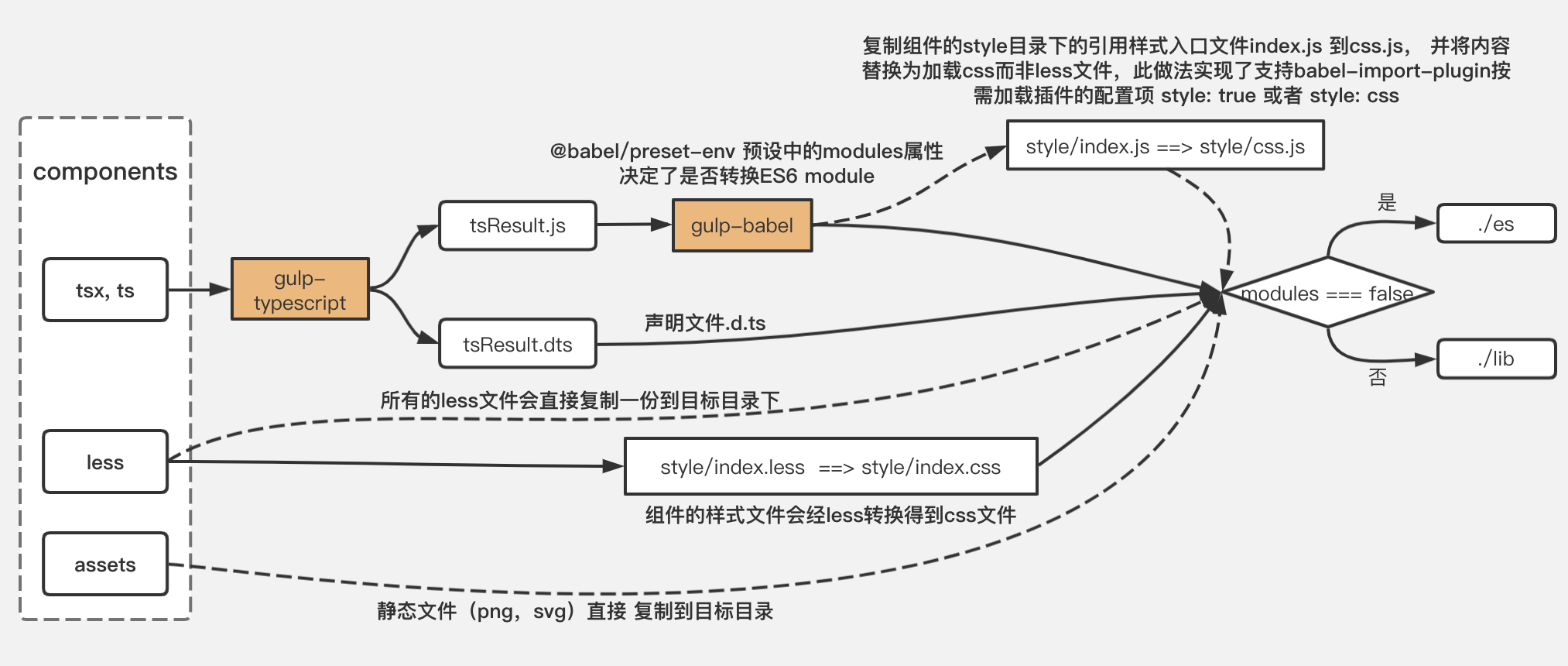
antd的编译过程中,tsc只编译到了ES6的程度,剩下的由babel进行了处理。
注:
babel-plugin-import
插件,可将import 替换为requirelib/index.js
相关库
through2
: 一般gulp的插件都会用through2
,这是因为gulp使用了vinyl-fs
,而vinyl-fs
使用了through2
,这是一个封装了Node stream的库,更容易操作streamcommander
编写命令行的工具rimraf
清除目录内容