Closed kommaqueen closed 3 years ago
Hi @darathinas. It is hard to tell based on available information, can be a combination of plugins that you use, wrong css syntax or your operating system (not really sure why you see such output in the console).
Is it possible to set up a test project that reproduces the issue?
Hi @w0rm ! Thank you for your reply! Currently, node and all the files are set up on my local server. Would perhaps seeing the gulpfile.js would be helpful?
[Parts of it were commented out, but not by my own doing, was already commented out when I went into it]
`// generated on 2020-09-25 using generator-webapp 4.0.0-8 const { src, dest, watch, series, parallel, lastRun } = require('gulp'); const gulpLoadPlugins = require('gulp-load-plugins'); const fs = require('fs'); const mkdirp = require('mkdirp'); const Modernizr = require('modernizr'); const browserSync = require('browser-sync'); const del = require('del'); const autoprefixer = require('autoprefixer'); const cssnano = require('cssnano'); const { argv } = require('yargs');
const $ = gulpLoadPlugins(); const server = browserSync.create();
const port = argv.port || 9000;
const isProd = process.env.NODE_ENV === 'production'; const isTest = process.env.NODE_ENV === 'test'; const isDev = !isProd && !isTest;
function styles() { return src('app/styles/*.scss', { sourcemaps: !isProd, }) .pipe($.plumber()) .pipe($.sass.sync({ outputStyle: 'expanded', precision: 10, includePaths: ['.'] }).on('error', $.sass.logError)) .pipe($.postcss([ autoprefixer() ])) .pipe(dest('.tmp/styles', { sourcemaps: !isProd, })) .pipe(server.reload({stream: true})); };
function scripts() { return src('app/scripts/*/.js', { sourcemaps: !isProd, }) .pipe($.plumber()) .pipe($.babel()) .pipe(dest('.tmp/scripts', { sourcemaps: !isProd ? '.' : false, })) .pipe(server.reload({stream: true})); };
async function modernizr() {
const readConfig = () => new Promise((resolve, reject) => {
fs.readFile(${__dirname}/modernizr.json
, 'utf8', (err, data) => {
if (err) reject(err);
resolve(JSON.parse(data));
})
})
const createDir = () => new Promise((resolve, reject) => {
mkdirp(${__dirname}/.tmp/scripts
, err => {
if (err) reject(err);
resolve();
})
});
const generateScript = config => new Promise((resolve, reject) => {
Modernizr.build(config, content => {
fs.writeFile(${__dirname}/.tmp/scripts/modernizr.js
, content, err => {
if (err) reject(err);
resolve(content);
});
})
});
const [config] = await Promise.all([ readConfig(), createDir() ]); await generateScript(config); }
const lintBase = (files, options) => { return src(files) .pipe($.eslint(options)) .pipe(server.reload({stream: true, once: true})) .pipe($.eslint.format()) .pipe($.if(!server.active, $.eslint.failAfterError())); } function lint() { return lintBase('app/scripts/*/.js', { fix: true }) .pipe(dest('app/scripts')); }; function lintTest() { return lintBase('test/spec/*/.js'); };
function html() { return src('app/.html') .pipe($.useref({searchPath: ['.tmp', 'app', '.']})) .pipe($.if(/.js$/, $.uglify({compress: {drop_console: true}}))) .pipe($.if(/.css$/, $.postcss([cssnano({safe: true, autoprefixer: false})]))) /.pipe($.if(/.html$/, $.htmlmin({ collapseWhitespace: true, minifyCSS: true, minifyJS: {compress: {drop_console: true}}, processConditionalComments: true, removeComments: true, removeEmptyAttributes: true, removeScriptTypeAttributes: true, removeStyleLinkTypeAttributes: true })))*/ .pipe(dest('dist')); }
function images() { return src('app/images/*/', { since: lastRun(images) }) .pipe($.imagemin()) .pipe(dest('dist/images')); };
function fonts() { return src('app/fonts/*/.{eot,svg,ttf,woff,woff2}') .pipe($.if(!isProd, dest('.tmp/fonts'), dest('dist/fonts'))); };
function extras() { return src([ 'app/', '!app/.html' ], { dot: true }).pipe(dest('dist')); };
function clean() { return del(['.tmp', 'dist']) }
function measureSize() { return src('dist/*/') .pipe($.size({title: 'build', gzip: true})); }
const build = series( clean, parallel( lint, series(parallel(styles, scripts, modernizr), html), images, fonts, extras ), measureSize );
function startAppServer() { server.init({ notify: false, port, server: { baseDir: ['.tmp', 'app'], routes: { '/node_modules': 'node_modules' } } });
watch([ 'app/*.html', 'app/images/*/', '.tmp/fonts/*/' ]).on('change', server.reload);
watch('app/styles//*.scss', styles); watch('app/scripts/*/.js', scripts); watch('modernizr.json', modernizr); watch('app/fonts//*', fonts); }
function startTestServer() { server.init({ notify: false, port, ui: false, server: { baseDir: 'test', routes: { '/scripts': '.tmp/scripts', '/node_modules': 'node_modules' } } });
watch('test/index.html').on('change', server.reload); watch('app/scripts/*/.js', scripts); watch('test/spec/*/.js', lintTest); }
function startDistServer() { server.init({ notify: false, port, server: { baseDir: 'dist', routes: { '/node_modules': 'node_modules' } } }); }
let serve; if (isDev) { serve = series(clean, parallel(styles, scripts, modernizr, fonts), startAppServer); } else if (isTest) { serve = series(clean, scripts, startTestServer); } else if (isProd) { serve = series(build, startDistServer); }
exports.serve = serve; exports.build = build; exports.default = build; `
Can you set up a test project that reproduces the issue and is limited to:
Perhaps by trimming it down you can figure the issue. But if not — I can try debugging it myself.
Hi @w0rm ! Unfortunately because the scss problem is actually random – if I take out the code in question, node just finds another part of the file to say is incorrect – and I don't fully understand the complexities of gulp plugins and gulpfile tasks, a test project may be difficult... but I have uploaded the depository online and if you could take a look at it that would be much appreciated. I can give you access to it.
@darathinas what if you keep just a few lines of scss? Would this break too? In this case I am doubt that scss is the problem, but rather the combination of plugins.
@w0rm I hadn't added any new plugins since I first initialized Gulp. Of course, that may just be the nature of bugs, and you are probably right. I must note that I know very little about the intricacies of this program, only that it was working once and suddenly isn't now. I gave you access to my repository. Is that sufficient to help diagnose this problem? I'm worried now my code is stuck in this broken gulp/node thing. It seems you know your stuff very well. I appreciate your time and help already.
I offered some ideas but I don't have enough time to debug through the whole source code. Sorry!
This is where the issue arises in the gulpfile, on the postcss call
function html() {
return src('app/.html')
.pipe($.useref({searchPath: ['.tmp', 'app', '.']}))
.pipe($.if(/.js$/, $.uglify({compress: {drop_console: true}})))
.pipe($.if(/.css$/, $.postcss([cssnano({safe: true, autoprefixer: false})])))
.pipe(dest('dist'));
}
The line in the scss file that it points to is what was mentioned in the OP
#team .row {
padding-left: 0!important;
padding-right: 0!important;
}
which obviously seems very different from the output in the screenshot above.
I have been using Gulp with no problems for months and now, when I'm about to finish my site, "npm run build," and bam, I run into a build error.
A friend of mine tried to fix it for about two hours, but couldn't figure it out. We've tried linting the whole main.css file, the intermediary .tmp css file, the css file output to dist switched the encoding on main.scss from ASCII to UTF-*, tried on postcss 8 & 9, tried it without postcsss plugins... nothing worked.
Here is the error as it appears in Terminal: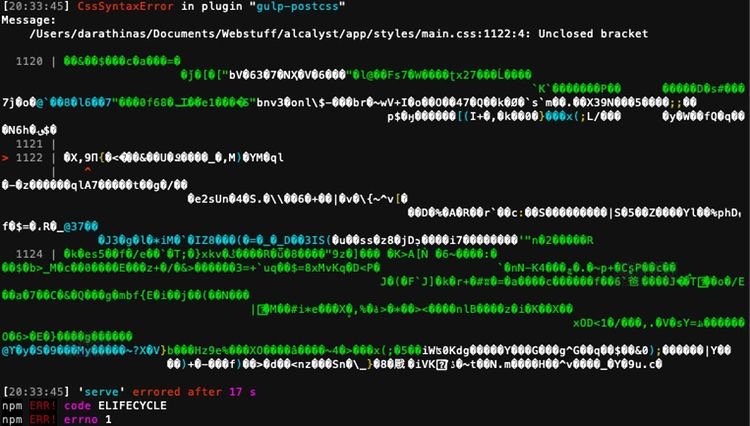
And the area it claims is missing a bracket (my css is scss and there are multiple instances of scss syntax throughout this same document and has up to now never have any issues – I also validated the entire file for such an error and found none):
Any advice or solution would be much appreciated!