Closed pg07codes closed 2 years ago
i resolved the above issue using compat version and not the recently released moduler firebase sdk.
import firebase from "firebase/compat/app" import "firebase/compat/database" // import "firebase/compat/[SERVICE_NAME]"
using the compat version to keep using the existing api seems to be the only way for now.
i followed the react-redux-firebase readme to create this file
store.js is just using redux toolkit to create and export a store.
and also using the demo code given on react-redux-firebase website
when i click on add this is the exception i get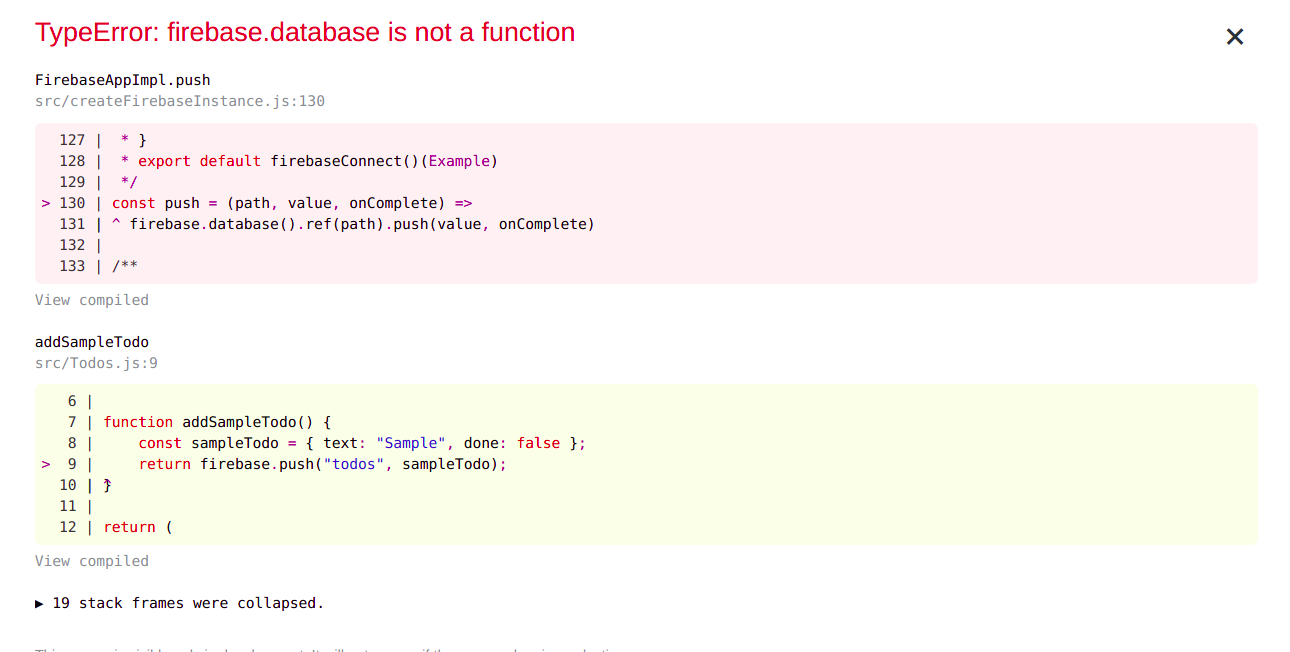
will appreciate any help with this ?
TIA