Closed carolinemcq closed 4 years ago
Can you provide the full application code where this can be reproduced? Looks like it is react-native?
Can you provide the full application code where this can be reproduced? Looks like it is react-native?
Sure, here's a link: https://snack.expo.io/@carriemcq/react-redux-firebase-setup
I believe the issue is the extra root reducer you were passing which wasn't returning any state. I'll show a working file then I'll break down some things I found
Here is a modified version of that app file:
import React from 'react';
import { createStore, combineReducers } from "redux";
import { Provider } from "react-redux";
import {
firebaseReducer,
ReactReduxFirebaseProvider,
} from "react-redux-firebase";
import { createFirestoreInstance, firestoreReducer } from "redux-firestore";
import * as firebase from "firebase/app";
import "firebase/auth";
import "firebase/firestore";
import Home from "./views/Home.js"
const firebaseConfig = {
apiKey: "AIzaSyDiogeWwC80O2D7JTvui_qDF4J6h4Kg9a4",
authDomain: "dummy-project-42fa0.firebaseapp.com",
databaseURL: "https://dummy-project-42fa0.firebaseio.com",
projectId: "dummy-project-42fa0",
storageBucket: "dummy-project-42fa0.appspot.com",
messagingSenderId: "664519164863",
appId: "1:664519164863:web:1156cdd5bdb43c9a8c970a"
};
const rrfConfig = {
userProfile: "users",
useFirestoreForProfile: true,
// enableClaims: true, // Get custom claims along with the profile
};
if (!firebase.apps.length) {
firebase.initializeApp(firebaseConfig);
// NOTE: firebase.firestore() is skipped due to an issue within snack.expo where it is loading the firebase/firestore package instead of loading from firebase the firebase/firestore path.
// firebase.firestore();
}
const rootReducer = combineReducers({
firebase: firebaseReducer,
firestore: firestoreReducer,
});
const initialState = {
action: ""
}
const store = createStore(rootReducer);
const rrfProps = {
firebase,
config: rrfConfig,
dispatch: store.dispatch,
createFirestoreInstance,
};
const App = () => (
<Provider store={store}>
<ReactReduxFirebaseProvider {...rrfProps}>
<Home />
</ReactReduxFirebaseProvider>
</Provider>
);
export default App;
Things that were changed:
Other things of note:
Cannot read property type of undefined
error firebase/firestore
and other packages shouldn't need to be installed - as described in the setup guide you should only have to do npm install --save firebase
not add the other packages. I'm assuming that it might have been done by the snack.expo.io tool since it tried to do the same for me after I removed them from the package file. Not sure this is impacting anything, but still worth noting
Bug
I was following the getting started javascript instructions posted here and get the following Console error:
"The previous state received by the reducer has unexpected type of "Function". Expected argument to be an object with the following keys: "firebase", "firestore"".
Project info:
Screenshot of error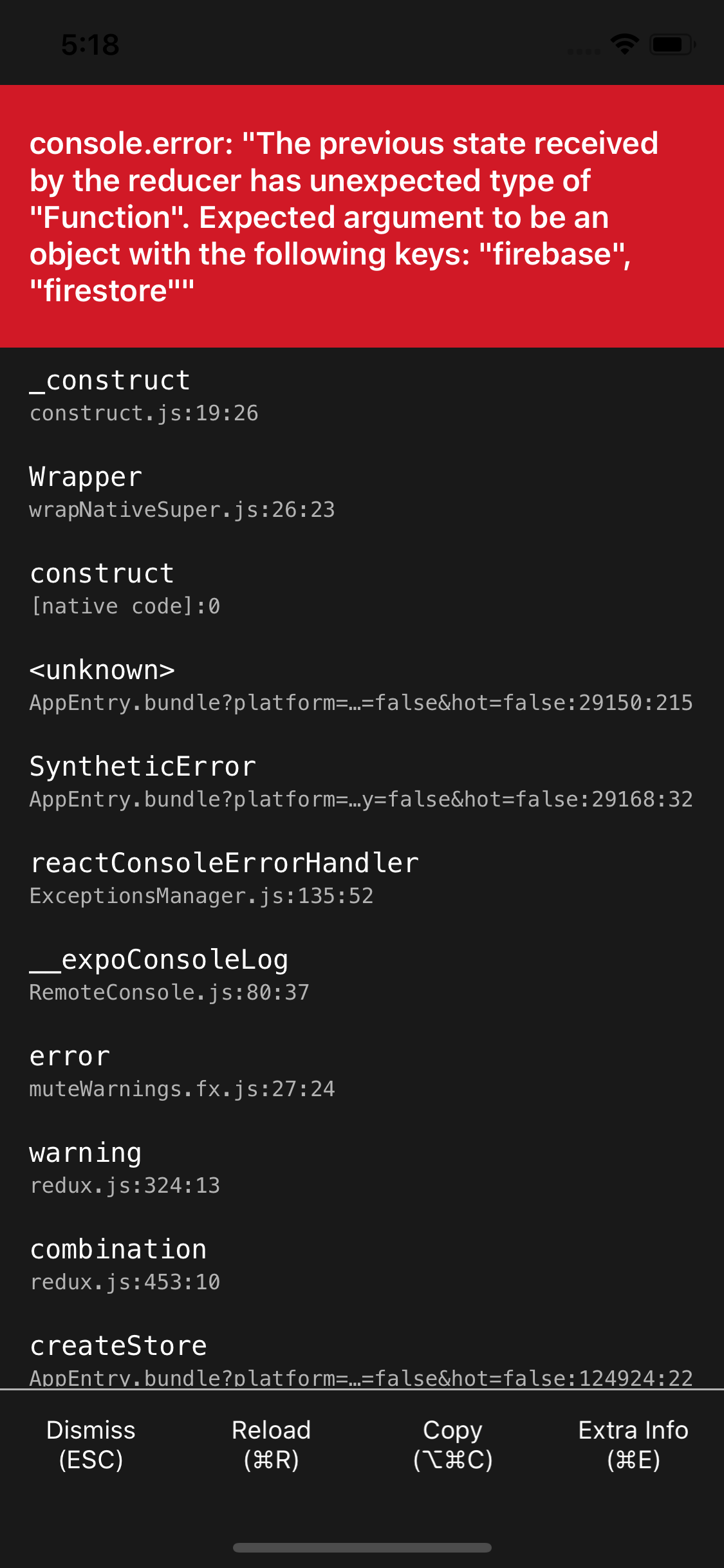