Open cesarecaoduro opened 4 years ago
Seems like a CSS problem. Try adding a wrapper on the CanvasWidget
with full width and height, or try to mess with the CSS on the DevTools. Also, these are related:
Doesn't work for me. I can have the right background color but still the node are not showing
Please check this solution: https://github.com/projectstorm/react-diagrams/issues/511#issuecomment-584611504
Posted an update to this issue in #511
I'm facing the same problem trying to go through the documentation.
Firstly, I was facing with problem described in https://github.com/projectstorm/react-diagrams/issues/598. After some investigation I've found that problem is related to link
. So after commenting link I faced with white screen only.
The code is
import React from "react";
import "./App.css";
import createEngine, {
DefaultLinkModel,
DefaultNodeModel,
DiagramModel,
} from "@projectstorm/react-diagrams";
import { CanvasWidget } from "@projectstorm/react-canvas-core";
function App() {
// create an instance of the engine with all the defaults
const engine = createEngine();
// node 1
const node1 = new DefaultNodeModel({
name: "Node 1",
color: "rgb(0,192,255)",
});
node1.setPosition(100, 100);
let port1 = node1.addOutPort("Out");
// // node 2
const node2 = new DefaultNodeModel({
name: "Node 1",
color: "rgb(0,192,255)",
});
node2.setPosition(100, 100);
let port2 = node2.addOutPort("Out");
// // link them and add a label to the link
const link = port1.link<DefaultLinkModel>(port2);
link.addLabel("Hello World!");
const model = new DiagramModel();
// model.addAll(node1, node2, link);
model.addAll(node1, node2);
engine.setModel(model);
return (
<div>
<CanvasWidget engine={engine} />
</div>
);
}
export default App;
Screen is
Also, I have tried with code provided by @cesarecaoduro and have reproduced the issue.
Yeap this one https://github.com/projectstorm/react-diagrams/issues/511#issuecomment-608591533 from @mkellogg91 is working
CanvasWidget must have position: inherit to make this work. Unfortunately style is not accepted prop so we need to use className
I got it to work as follows:
import React from 'react';
import { createStyles, makeStyles } from '@material-ui/core';
import createEngine, { DefaultLinkModel, DefaultNodeModel, DiagramModel } from '@projectstorm/react-diagrams';
import { CanvasWidget } from '@projectstorm/react-canvas-core';
const useStyles = makeStyles(() =>
createStyles({
canvas: {
height: '100vh'
}
})
);
export const Amazing: React.FC = () => {
const classes = useStyles();
// create an instance of the engine with all the defaults
const engine = createEngine();
// node 1
const node1 = new DefaultNodeModel({
name: 'Node 1',
color: 'rgb(0,192,255)'
});
node1.setPosition(100, 100);
let port1 = node1.addOutPort('Out');
// node 2
const node2 = new DefaultNodeModel({
name: 'Node 2',
color: 'rgb(0,192,255)'
});
node2.setPosition(500, 100);
let port2 = node2.addInPort('In');
// link them and add a label to the link
const link = port1.link<DefaultLinkModel>(port2);
link.addLabel('Hello World!');
const model = new DiagramModel();
model.addAll(node1, node2, link);
engine.setModel(model);
return <CanvasWidget engine={engine} className={classes.canvas} />;
};
I'm actually having the same issue, besides, I am currently unable to make the diagram occupy only part of the screen. Anyone has updates on it?
I am using a basic CreateReactApp installation, added all the dependencies including the support for typescript, the code doesn't generate any error, but the canvas is completely white. In the developer console it seems that something is created but I am not able to do anything.
This is the component .tsx
And this is my package.json
And the tsconfig.json
Something is there for sure and this is driving me nuts!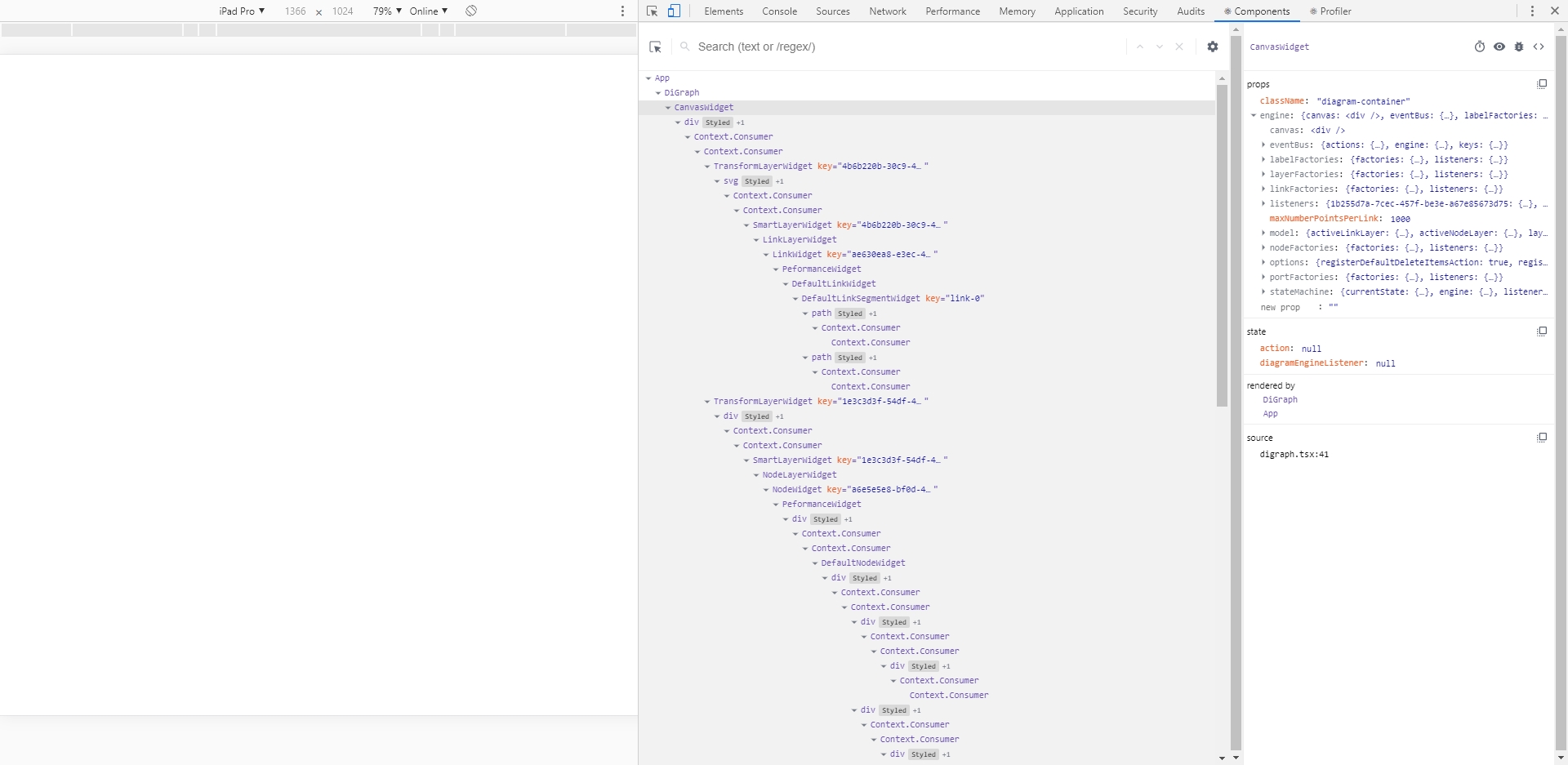