Issue №1984 opened by Bmorr1123 at 2020-06-25 04:23:04
By incorrect I mean that it accurately reports the resolution of the display, but it does not account for desktop scaling.
For example.:
My monitor is 3840x2400 and pygame.display.Info() reports that, but I have my display scaled to 200% because on my 13" screen, everything would be way too small. The problem with this is when I want to use my automatic window sizing code, pygame.display.Info() doesn't tell me the desktop scaling. This means that when I set my window to 90% of the screen size, it's actually 180% of the screen size.
We either need a way to prevent the window from being scaled by windows or just another field in pygame.display.Info() that tells us the desktop scaling. I don't know how difficult either of these would be to implement, but they would be extremely useful!
Your pygame app is being scaled by 200% when your desktop is set to scale things by 200%. This sounds like the familiar SDL issue about needing to set applications to be DPIAware on windows. You might try passing the SCALED flag to set_mode() to see if that accidentally solves this issue for you.
You'd like display info to have an extra field reporting the actual screen resolution multiplied by the inverse of the desktop scaling to be able to counteract the effects of 1. i.e you make a smaller app that would then be scaled up by windows to try and fit the screen.
See more about High DPI Awareness on all platforms here:
From the first link this bit of C code looks like a promising start to a cross-platform way of getting the current desktop 'zoom' level:
void MySDL_GetDisplayDPI(int displayIndex, float* dpi, float* defaultDpi)
{
const float kSysDefaultDpi =
# ifdef __APPLE__
72.0f;
# elif defined(_WIN32)
96.0f;
# else
static_assert(false, "No system default DPI set for this platform.");
# endif
if (SDL_GetDisplayDPI(displayIndex, NULL, dpi, NULL) != 0)
{
// Failed to get DPI, so just return the default value.
if (dpi) *dpi = kSysDefaultDpi;
}
if (defaultDpi) *defaultDpi = kSysDefaultDpi;
}
Which could then be used as a multiplier for two extra display info fields.
Though I think I read that GNOME on linux now also has a similar DPI scaling system and no doubt others have made something too since this article was written.
I think the underlying issue is that DPI scaling is still a fairly recently appearing, per-platform mess that has taken a while to settle into stable APIs and SDL hasn't yet developed a cross-platform API to deal with it.
Hello, I can see during this 4 years, the problem got fixed. I'm on windows and I don't have this issue for whatever scaling resolution I use. Is it still a thing on other OS ?
Issue №1984 opened by Bmorr1123 at 2020-06-25 04:23:04
By incorrect I mean that it accurately reports the resolution of the display, but it does not account for desktop scaling.
For example.: My monitor is 3840x2400 and pygame.display.Info() reports that, but I have my display scaled to 200% because on my 13" screen, everything would be way too small. The problem with this is when I want to use my automatic window sizing code, pygame.display.Info() doesn't tell me the desktop scaling. This means that when I set my window to 90% of the screen size, it's actually 180% of the screen size.
We either need a way to prevent the window from being scaled by windows or just another field in pygame.display.Info() that tells us the desktop scaling. I don't know how difficult either of these would be to implement, but they would be extremely useful!
Here's my code for replication:
and here's a screenshot of the window size: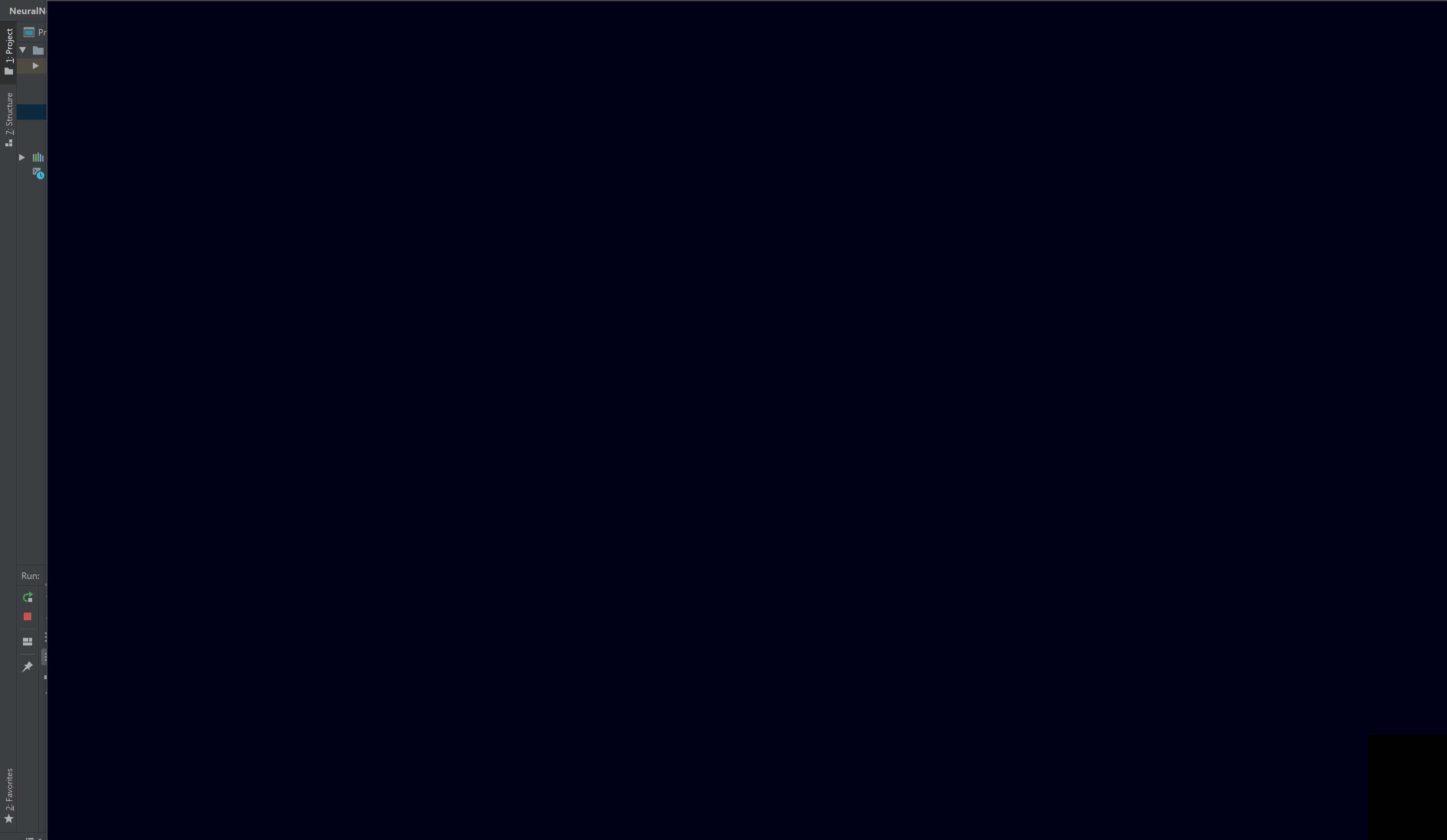
EDIT: The pygame window in the picture is 180% of my screen width, and the black rect covers the 4th quadrant if you want scale.
Comments
*MyreMylar commented at 2020-06-25 07:14:37*
So it sounds like two things are going on here:
Your pygame app is being scaled by 200% when your desktop is set to scale things by 200%. This sounds like the familiar SDL issue about needing to set applications to be DPIAware on windows. You might try passing the SCALED flag to
set_mode()
to see if that accidentally solves this issue for you.You'd like display info to have an extra field reporting the actual screen resolution multiplied by the inverse of the desktop scaling to be able to counteract the effects of 1. i.e you make a smaller app that would then be scaled up by windows to try and fit the screen.
See more about High DPI Awareness on all platforms here:
https://nlguillemot.wordpress.com/2016/12/11/high-dpi-rendering/ https://stackoverflow.com/questions/44398075/can-dpi-scaling-be-enabled-disabled-programmatically-on-a-per-session-basis https://wiki.libsdl.org/SDL_CreateWindow https://docs.microsoft.com/en-gb/windows/win32/sbscs/application-manifests?redirectedfrom=MSDN
From the first link this bit of C code looks like a promising start to a cross-platform way of getting the current desktop 'zoom' level:
Which could then be used as a multiplier for two extra display info fields.
Though I think I read that GNOME on linux now also has a similar DPI scaling system and no doubt others have made something too since this article was written.
I think the underlying issue is that DPI scaling is still a fairly recently appearing, per-platform mess that has taken a while to settle into stable APIs and SDL hasn't yet developed a cross-platform API to deal with it.