Closed Andre601 closed 5 years ago
The CommandDescription was designed so that JDA-Command doesn't come with methods out of the box that people may not like / want to use.
The main power of JDA-Command comes from the CommandAttributes - allow me to demonstrate.
package uk.rgsw.arrowsmith.playground;
import com.github.rainestormee.jdacommand.Command;
import com.github.rainestormee.jdacommand.CommandAttribute;
import com.github.rainestormee.jdacommand.CommandDescription;
import net.dv8tion.jda.core.EmbedBuilder;
import net.dv8tion.jda.core.entities.Message;
import net.dv8tion.jda.core.entities.MessageEmbed;
import java.util.Arrays;
@CommandDescription(
name = "Help",
description = "Shows all commands or gives information about a command,",
triggers = {"help", "commands"},
attributes = {
@CommandAttribute(key = "category:help"),
@CommandAttribute(key = "syntax", value = "help [command]") // Here we use a Attribute with a specified description
}
)
public class HelpCommand implements Command {
private MessageEmbed commandInfo(Command cmd, String prefix){
CommandDescription desc = cmd.getDescription();
EmbedBuilder builder = new EmbedBuilder()
.setTitle(desc.name())
.setDescription(desc.description())
.addField("Usage:", String.format("%s%s", prefix, cmd.getAttributeValueFromKey("syntax")), true)
.addField("Aliases:", String.join(", ", desc.triggers()), true)
.setColor(0x50E3C2);
return builder.build();
}
@Override
public void execute(Message message, String s) {
// Implement execute command as normal.
}
}
Edit 1 - Java formatting Edit 2 - I just realised this method is implemented by default and does not need to be defined.
Okay I've pushed a new release for JDA-Command, removing some unnecessary stuff (the @Category interface that was being used for a specific project was dropped).
I've also removed the args argument for the @CommandDescription and I am debating of removing more of these unnecessary arguments and allowing people to generate them as they wish using CommandAttributes.
import com.github.rainestormee.jdacommand.AbstractCommand;
import net.dv8tion.jda.core.entities.Message;
public abstract class Command implements AbstractCommand<Message> {} // For JDA-Specific Implementation just write this class and use it as you wish
import com.github.rainestormee.jdacommand.CommandAttribute;
import com.github.rainestormee.jdacommand.CommandDescription;
import net.dv8tion.jda.core.EmbedBuilder;
import net.dv8tion.jda.core.entities.Message;
import net.dv8tion.jda.core.entities.MessageEmbed;
@CommandDescription(
name = "Help",
description = "Shows all commands or gives information about a command,",
triggers = {"help", "commands"},
attributes = {
@CommandAttribute(key = "category:help"),
@CommandAttribute(key = "syntax", value = "help [command]") // Here we use a Attribute with a specified description
}
)
public class HelpCommand extends Command {
private MessageEmbed commandInfo(Command cmd, String prefix){
CommandDescription desc = cmd.getDescription();
EmbedBuilder builder = new EmbedBuilder()
.setTitle(desc.name())
.setDescription(desc.description())
.addField("Usage:", String.format("%s%s", prefix, cmd.getAttribute("syntax")), true)
.addField("Aliases:", String.join(", ", desc.triggers()), true)
.setColor(0x50E3C2);
return builder.build();
}
@Override
public void execute(Message message, String s) {
// Implement execute command as normal.
}
}```
What now? Should I use the first example (That I currently add) or the second one?
If you are on the newer release (1.1.3 from today) then use the second one, but please note quite a few changes have happened, I'll highlight them in my Discord server with you can find here - https://discord.gg/DkYjvhf
It would be a good addition to have a "syntax" option for
@CommandDescription
to have a simple way for showing the syntax in f.e. a help-command (Like f.e.!help <command>
shows the description and also the syntax)How it would look like:
This would be the result (Made with the Embed Visualizer):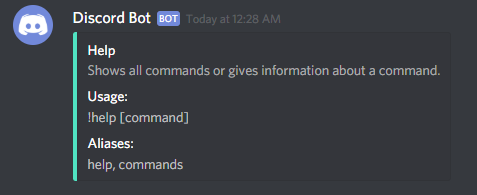