// Selecting Button A
const btnA = document.querySelector('#A');
// Selecting Button B
const btnA = document.querySelector('#B');
// Selecting the 2 divs with class name "button-wrapper"
const divs = document.querySelectorAll('.button-wrapper');
You can target them in CSS to apply targeted styling:
div.button-wrapper {
/* You can style both divs here */
}
button {
/* This styles ALL 4 buttons */
}
button#A {
/* This only styles Button A */
}
Now, define a function that's triggered by a button-click event:
```JS
// Note the "event" param.
function handleClick(event) {
// Target the button that got clicked.
const theBtnThatGotClicked = event.currentTarget;
// Find the cloest div with class name "meal".
const theClosestMeal = theBtnThatGotClicked.closest('.meal');
// Select the div with class "dish" within the "meal" div.
const theDishInside = theClosestMeal.querySelector('.dish');
}
```
Then let's write another function that
```JS
function cook(rawEmoji) {
const food = {
'🐓': '🍗',
'🐄': '🍔',
'🌽': '🍿',
};
return food[rawEmoji];
}
```
Now we can add the functionality of "cooking" to finish off this previous func.:
```JS
// Note the "event" param.
function handleClick(event) {
const theBtnThatGotClicked = event.currentTarget;
const theClosestMeal = theBtnThatGotClicked.closest('.meal');
const theDishInside = theClosestMeal.querySelector('.dish');
// Cook using the `cook` function!
theDishInside.textContent = cook(theDishInside.textContent);
theBtnThatGotClicked.disabled = true;
theBtnThatGotClicked.textContent = 'Cooked!';
}
```
StarWars Species Exercise
async
function,getAllSpecies()
,await
the fetch call to the API URL.await
the parsing of the response from JSON into JavaScript (use the.json()
method)results
property from this datapopulateTable()
await
the invocation ofgetAllSpecies()
. (Remember what the returned result from that func. is...)<tbody>
in the HTML.map()
, and make a table row out of each result<tbody>
.innerHTML
CSS
SpeciFISHity Cheatsheet!
Center the table
auto
formargin
center
fortext-align
Text-align
Compare the different effects here.
Border collapse
Check out what border-collapse does here.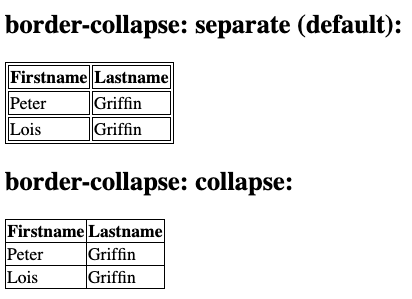
Text transform
You can capitalize all letters by setting the text-transform property:
Apply hovering rules:
While Loop
This loop will print out
myNum
's values from 8 to 28 in the console.The loop runs while
myNum
is less than 29.myNum++
, the loop will run infinitely (dangerous!), becausemyNum
will always be 8, which is less than 29.Try-Catch
We "try" something, and if it produces an error, we "catch" it.
Event Listeners
Quick overview of event listeners: https://www.w3schools.com/js/js_htmldom_eventlistener.asp
Let's target a button first:
<button>
s that exist on the HTML.Now let the button react to clicks:
Upon clicking this button, the function
_someFunction_
will be called.Class vs. ID
Let's say you have some buttons on the HTML:
Using JS to target specific elements:
You can target them in CSS to apply targeted styling:
Event Target
We have the button within a
<div>
:Now, define a function that's triggered by a button-click event: ```JS // Note the "event" param. function handleClick(event) { // Target the button that got clicked. const theBtnThatGotClicked = event.currentTarget; // Find the cloest div with class name "meal". const theClosestMeal = theBtnThatGotClicked.closest('.meal'); // Select the div with class "dish" within the "meal" div. const theDishInside = theClosestMeal.querySelector('.dish'); } ``` Then let's write another function that ```JS function cook(rawEmoji) { const food = { '🐓': '🍗', '🐄': '🍔', '🌽': '🍿', }; return food[rawEmoji]; } ``` Now we can add the functionality of "cooking" to finish off this previous func.: ```JS // Note the "event" param. function handleClick(event) { const theBtnThatGotClicked = event.currentTarget; const theClosestMeal = theBtnThatGotClicked.closest('.meal'); const theDishInside = theClosestMeal.querySelector('.dish'); // Cook using the `cook` function! theDishInside.textContent = cook(theDishInside.textContent); theBtnThatGotClicked.disabled = true; theBtnThatGotClicked.textContent = 'Cooked!'; } ```